In this article, you will see theĀ Top 10 Character/Alphabet patternĀ programs logic in java. We recommend you to see the pattern printing programs [Tricks] to develop the logic for almost all types of pattern programs in java.
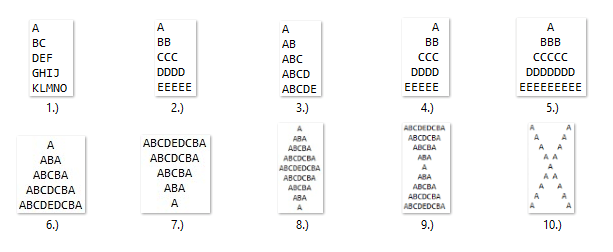
Let’s get started:
Table of Contents
Pattern-1

Letās see the java code below to understand this pattern:
package com.javacodepoint.patterns;
public class CharacterPattern1 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Initializing the character with alphabet A (ASCII value)
int character = 65;
// Outer loop for the line change
for (int i = 1; i <= n; i++) {
// Inner loop for the character printing
for (int j = 1; j <= i; j++) {
// Printing the character without changing the line
System.out.print((char)character++);
}
// Line change
System.out.println();
}
}
}
Pattern-2

Letās see the java code below to understand this pattern:
package com.javacodepoint.patterns;
public class CharacterPattern2 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Initializing the character with alphabet A (ASCII value)
int character = 65;
// Outer loop for the line change
for (int i = 1; i <= n; i++) {
// Inner loop for the character printing
for (int j = 1; j <= i; j++) {
// Printing the character without changing the line
System.out.print((char)character);
}
character++;
// Line change
System.out.println();
}
}
}
Pattern-3

Letās see the java code below to understand this pattern:
package com.javacodepoint.patterns;
public class CharacterPattern3 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Outer loop for the line change
for (int i = 1; i <= n; i++) {
// Initializing the character with alphabet A (ASCII value)
int character = 65;
// Inner loop for the character printing
for (int j = 1; j <= i; j++) {
// Printing the character without changing the line
System.out.print((char)character++);
}
// Line change
System.out.println();
}
}
}
Pattern-4

Letās see the java code below to understand this pattern:
package com.javacodepoint.patterns;
public class CharacterPattern4 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Initializing the character with alphabet A (ASCII value)
int character = 65;
// Outer loop for the line change
for (int i = 1; i <= n; i++) {
// Inner loop1 for the space printing
for (int j = 1; j <= n-i; j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the character printing
for (int k = 1; k <= i; k++) {
// Printing the character without changing the line
System.out.print((char)character);
}
character++;
// Line change
System.out.println();
}
}
}
Pattern-5

Letās see the java code below to understand this pattern:
package com.javacodepoint.patterns;
public class CharacterPattern5 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Initializing the character with alphabet A (ASCII value)
int character = 65;
// Outer loop for the line change
for (int i = 1; i <= n; i++) {
// Inner loop1 for the space printing
for (int j = 1; j <= n-i; j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the character printing
for (int k = 1; k <= (2*i)-1; k++) {
// Printing the character without changing the line
System.out.print((char)character);
}
character++;
// Line change
System.out.println();
}
}
}
Pattern-6

Letās see the java code below to understand this pattern:
package com.javacodepoint.patterns;
public class CharacterPattern6 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Outer loop for the line change
for (int i = 1; i <= n; i++) {
// Inner loop1 for the space printing
for (int j = 1; j <= n-i; j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Initializing the character before alphabet A (ASCII value)
int character = 64;
// Inner loop2 for the character printing
for (int k = 1; k <= (2*i)-1; k++) {
if(k <= i) {
// Printing the character without changing the line
System.out.print((char)++character);
}else {
// Printing the character without changing the line
System.out.print((char)--character);
}
}
// Line change
System.out.println();
}
}
}
Pattern-7

Letās see the java code below to understand this pattern:
package com.javacodepoint.patterns;
public class CharacterPattern7 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Outer loop for the line change
for (int i = n; i >= 1; i--) {
// Inner loop1 for the space printing
for (int j = 1; j <= n-i; j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Initializing the character before alphabet A (ASCII value)
int character = 64;
// Inner loop2 for the character printing
for (int k = 1; k <= (2*i)-1; k++) {
if(k <= i) {
// Printing the character without changing the line
System.out.print((char)++character);
}else {
// Printing the character without changing the line
System.out.print((char)--character);
}
}
// Line change
System.out.println();
}
}
}
Pattern-8
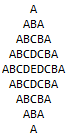
Letās see the java code below to understand this pattern:
package com.javacodepoint.patterns;
public class CharacterPattern8 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Upper half
// Outer loop for the line change
for (int i = 1; i <= n; i++) {
// Inner loop1 for the space printing
for (int j = 1; j <= n-i; j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Initializing the character before alphabet A (ASCII value)
int character = 64;
// Inner loop2 for the character printing
for (int k = 1; k <= (2*i)-1; k++) {
if(k <= i) {
// Printing the character without changing the line
System.out.print((char)++character);
}else {
// Printing the character without changing the line
System.out.print((char)--character);
}
}
// Line change
System.out.println();
}
// Lower half
// Outer loop for the line change
for (int i = n-1; i >= 1; i--) {
// Inner loop1 for the space printing
for (int j = 1; j <= n-i; j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Initializing the character before alphabet A (ASCII value)
int character = 64;
// Inner loop2 for the character printing
for (int k = 1; k <= (2*i)-1; k++) {
if(k <= i) {
// Printing the character without changing the line
System.out.print((char)++character);
}else {
// Printing the character without changing the line
System.out.print((char)--character);
}
}
// Line change
System.out.println();
}
}
}
Pattern-9
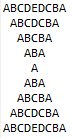
Letās see the java code below to understand this pattern:
package com.javacodepoint.patterns;
public class CharacterPattern9 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Upper half
// Outer loop for the line change
for (int i = n; i >= 1; i--) {
// Inner loop1 for the space printing
for (int j = 1; j <= n-i; j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Initializing the character before alphabet A (ASCII value)
int character = 64;
// Inner loop2 for the character printing
for (int k = 1; k <= (2*i)-1; k++) {
if(k <= i) {
// Printing the character without changing the line
System.out.print((char)++character);
}else {
// Printing the character without changing the line
System.out.print((char)--character);
}
}
// Line change
System.out.println();
}
// Lower half
// Outer loop for the line change
for (int i = 2; i <= n; i++) {
// Inner loop1 for the space printing
for (int j = 1; j <= n-i; j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Initializing the character before alphabet A (ASCII value)
int character = 64;
// Inner loop2 for the character printing
for (int k = 1; k <= (2*i)-1; k++) {
if(k <= i) {
// Printing the character without changing the line
System.out.print((char)++character);
}else {
// Printing the character without changing the line
System.out.print((char)--character);
}
}
// Line change
System.out.println();
}
}
}
Pattern-10
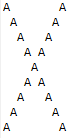
Letās see the java code below to understand this pattern:
package com.javacodepoint.patterns;
public class CharacterPattern10 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Upper half
// Outer loop for the line change
for (int i = n; i >= 1; i--) {
// Inner loop1 for the space printing
for (int j = 1; j <= n-i; j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the character printing
for (int k = 1; k <= (2*i)-1; k++) {
if(k == 1 || k == (2*i)-1) {
// Printing the character without changing the line
System.out.print("A");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line change
System.out.println();
}
// Lower half
// Outer loop for the line change
for (int i = 2; i <= n; i++) {
// Inner loop1 for the space printing
for (int j = 1; j <= n-i; j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the character printing
for (int k = 1; k <= (2*i)-1; k++) {
if(k == 1 || k == (2*i)-1) {
// Printing the character without changing the line
System.out.print("A");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line change
System.out.println();
}
}
}
Conclusion
In this article, you have seen the top 10 character pattern programs in java. Here you have seen, Right triangle pattern, Down right triangle pattern, Diamond pattern, Sandglass pattern, X Shape pattern, etc…
If you are interested to learn how to develop the logic for the pattern program through some tricks, then you can visit the link here: Pattern Printing Tricks.
Learn top 30 star pattern programs in java.