Problem statement: Develop a Java program (Word Search Solver Game) that can find all occurrences of a given set of words within a grid of letters, horizontally, vertically, or diagonally.
The Word Search Solver Game presents players with a grid of letters where they must find a given set of words. Words can appear horizontally, vertically, or diagonally within the grid.
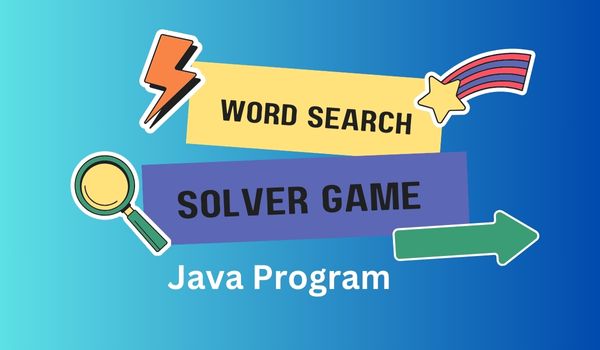
Word Search Solver Game Algorithm:
- Grid Initialization: Create a grid of letters.
- Word List: Provide a list of words that players must find within the grid.
- Search Algorithm: Implement an algorithm to search for each word within the grid.
- Start at each cell in the grid.
- Explore all eight directions (horizontal, vertical, and diagonal) to check for word matches.
- Keep track of the starting and ending positions of the words.
- Repeat this process for each word in the word list.
- Display: Present the grid to the player.
- User Input: Allow the player to input words they have found.
- Validation: Verify if the words provided by the player match those in the word list.
- Game End: End the game when all words have been found or when the player decides to quit.
Word Search Solver Game Rules:
- Players are presented with a grid of letters and a list of words to find.
- Words can appear horizontally, vertically, or diagonally in any direction.
- Players input the words they find, and the program validates whether the words are in the list.
- The game ends when all words have been found, or the player decides to quit.
Word Search Solver Game Implementation:
Components:
- Grid: Representing the grid of letters.
- Word Search Solver Class: Implementing the search algorithm.
- Main Method: Driving the game flow, taking user input, and displaying results.
Data Structures:
- 2D Array for the grid of letters.
- List or Array to store the words to be found.
Algorithm for Word Search:
- Start from each cell in the grid.
- Explore all eight directions from that cell.
- Keep track of visited cells to avoid reusing letters.
- Repeat this process for each word in the word list.
Word Search Solver Game Java Program:
The below example code illustrates how you can start implementing the Word Search Solver Game in Java.
package com.javacodepoint.game;
import java.util.Arrays;
import java.util.List;
public class WordSearchSolver {
private char[][] grid;
private List<String> wordList;
public WordSearchSolver(char[][] grid, List<String> wordList) {
this.grid = grid;
this.wordList = wordList;
}
public void solveWordSearch() {
for (String word : wordList) {
boolean found = false;
for (int i = 0; i < grid.length; i++) {
for (int j = 0; j < grid[0].length; j++) {
if (searchFromPosition(word, i, j)) {
found = true;
System.out.println("Word \"" + word + "\" found at position (" + i + "," + j + ")");
}
}
}
if (!found) {
System.out.println("Word \"" + word + "\" not found!");
}
}
}
private boolean searchFromPosition(String word, int row, int col) {
if (word.length() == 0) {
return true; // Empty word is always found
}
if (row < 0 || row >= grid.length || col < 0 || col >= grid[0].length) {
return false; // Out of bounds
}
if (grid[row][col] != word.charAt(0)) {
return false; // First letter doesn't match
}
char original = grid[row][col];
grid[row][col] = '*'; // Mark the cell as visited
// Explore all eight directions
boolean found = searchFromPosition(word.substring(1), row - 1, col)
|| searchFromPosition(word.substring(1), row + 1, col)
|| searchFromPosition(word.substring(1), row, col - 1)
|| searchFromPosition(word.substring(1), row, col + 1)
|| searchFromPosition(word.substring(1), row - 1, col - 1)
|| searchFromPosition(word.substring(1), row - 1, col + 1)
|| searchFromPosition(word.substring(1), row + 1, col - 1)
|| searchFromPosition(word.substring(1), row + 1, col + 1);
grid[row][col] = original; // Restore the cell
return found;
}
public void printGrid() {
for (int i = 0; i < grid.length; i++) {
for (int j = 0; j < grid[0].length; j++) {
System.out.print(grid[i][j] + " ");
}
System.out.println(); // Move to the next line for the next row
}
}
public static void main(String[] args) {
char[][] grid = {
{ 'A', 'B', 'C' },
{ 'D', 'E', 'F' },
{ 'G', 'H', 'I' }
};
List<String> wordList = Arrays.asList("ABC", "FI", "EH", "ADG");
WordSearchSolver solver = new WordSearchSolver(grid, wordList);
solver.printGrid();
solver.solveWordSearch();
}
}
OUTPUT:
A B C
D E F
G H I
Word “ABC” found at position (0,0)
Word “FI” found at position (1,2)
Word “EH” found at position (1,1)
Word “ADG” found at position (0,0)
Here’s a brief explanation of the code:
- The
WordSearchSolver
class represents the solver for the word search game. - The
solveWordSearch
method iterates through each word in the word list and searches for it in the grid. - The
searchFromPosition
method is a recursive function that starts searching for the given word from a specific position in the grid. - The
printGrid
method iterates through each cell of the grid and prints the characters along with spaces. - It checks if the current cell matches the first letter of the word.
- If it matches, it explores all eight directions (horizontal, vertical, and diagonal) recursively to find the remaining letters of the word.
- It marks visited cells with a special character to avoid revisiting them.
- After exploring, it restores the original state of the grid.
- The
main
method demonstrates how to use theWordSearchSolver
class to solve a word search puzzle with a given grid and word list.
Conclusion
The Word Search Solver Game in Java presents an entertaining challenge where players search for the words within a grid of letters. With its recursive search algorithms and interactive interface, the game offers engaging gameplay suitable for all ages. It’s a fun and stimulating way to sharpen problem-solving skills while enjoying the timeless allure of word puzzles.
See also: Tic-Tac-Toe Game | Hangman Game | Generating OTP in Java | Search Matrix