In this article, you will see how to print Star patterns in java. Here you will learn the simple tricks to develop the logic for Star pattern printing. These tricks will not only help you to understand the Star pattern programs but also help you to understand Alphabet/Character patterns and Number patterns.
Pattern printing programs help you to enhance your logical skills, coding, and looping concept. These programs are frequently asked by the interviewer to check the logical skills of the programmer.
Table of Contents
Tricks to develop pattern programs logic
Here you are going to learn 8 simple tricks to develop almost all types of pattern programs. All the tricks will be in three steps, first two steps are simple but the third step is a little tricky. The third step is very important for developing the logic.
Let’s see all the 8 tricks one by one:
Trick-1
*
**
***
****
*****
Let’s develop the logic for the above pattern:
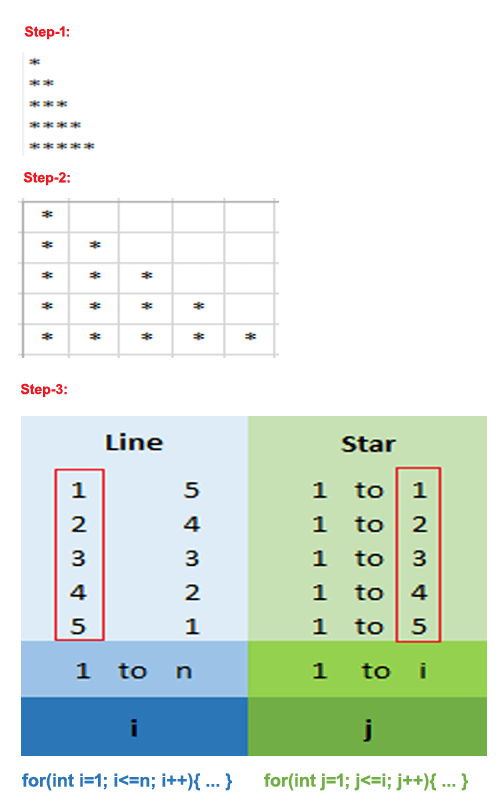
Let me explain the above steps with simple points:
Step-1:
- Given pattern
Step-2:
- The given pattern can be converted in to the box format like the above.
- The box format is repersented in Rows(1,2,3,4,5) and Columns(1,2,3,4,5).
Step-3:
- Let’s assume variable n(required number of rows), eg. n=5.
- In the above image, the blue color area representing the Line(row) whereas the green color area representing the Star printing.
- Line can be represented either in increasing order(1,2,3,4,5) or in decreasing order(5,4,3,2,1).
- In the given pattern, first-line is printing 1 star, second-line is printing 2 stars, third-line is printing 3 stars, and so on. Now you can see in the green area, we have mentioned it like 1 to 1, 1 to 2, 1 to 3, 1 to 4, and 1 to 5.
- Now match the common patterns from both(blue area and green area), here you can see we have matched it with red color box.
- Now as we have selected lines in increasing order(1,2,3,4,5), so we can write it like 1 to n and then it can be further assumed as the variable i.
- Similarly in the green area, we can write it like 1 to i and then it can be further assumed as the vriable j.
- Now we can write a for loop for the variable i is like for(int i=1; i<=n; i++){ …} to change the line and another for loop for the variable j is like for(int j=1; j<=i; j++){ …} to print the star. Hence, here we required only two for loops to print the given pattern.
Let’s see the complete logic below:
package com.javacodepoint.patterns;
public class StarPattern {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Trick-2
*****
****
***
**
*
Let’s develop the logic for it:
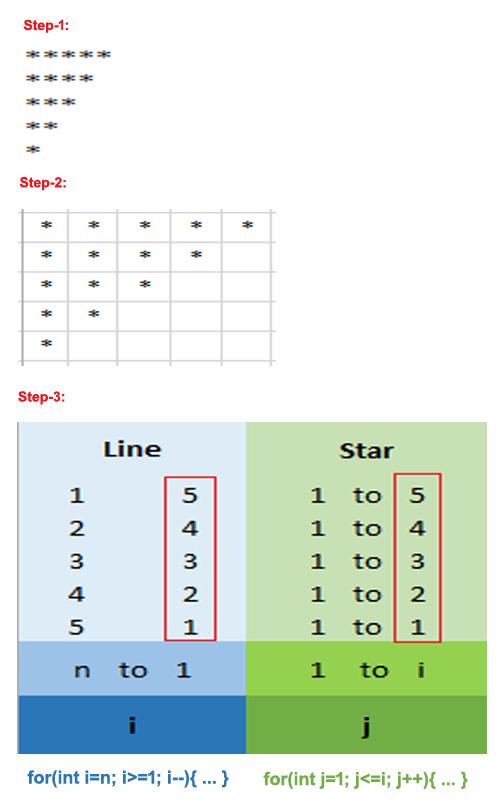
Let understand the above steps:
Step-1:
- Given pattern
Step-2:
- The given pattern can be converted in to the box format like the above.
- The box format is repersented in Rows(1,2,3,4,5) and Columns(1,2,3,4,5).
Step-3:
- Let’s assume n=5 (required number of rows).
- Here also the blue color area representing the Line(row) whereas the green color area representing the Star printing.
- Line can be represented either in increasing order(1,2,3,4,5) or in decreasing order(5,4,3,2,1).
- In the given pattern, first-line is printing 5 star, second-line is printing 4 stars, third-line is printing 3 stars, and so on. Now you can see in the green area, we have mentioned it like 1 to 5, 1 to 4, 1 to 3, 1 to 2, and 1 to 1.
- Now match the common patterns from both(blue area and green area), here you can see we have matched it with red color box.
- Now as we have selected lines in decreasing order(5,4,3,2,1), so we can write it like n to 1 and then it can be further assumed as the variable i.
- Similarly in the green area, we can write it like 1 to i and then it can be further assumed as the vriable j.
- Now we can write a for loop for the variable i is like for(int i=n; i>=1; i–){ …} to change the line and another for loop for the variable j is like for(int j=1; j<=i; j++){ …} to print the star. Hence, here also we required only two for loops to print the given pattern.
Let’s see the complete logic below:
package com.javacodepoint.patterns;
public class StarPattern {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Trick-3
![Star pattern programs [Tricks] in java 1 3. min](https://javacodepoint.com/wp-content/uploads/2021/08/3.-min.png)
Follow the below steps to develop the logic:
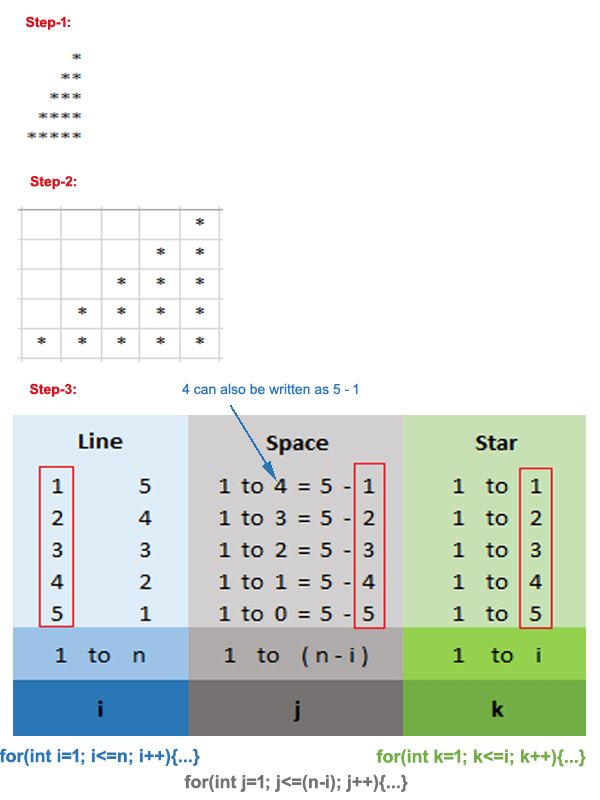
Let me explain the above steps:
Step-1:
- Given pattern
Step-2:
- The given pattern can be converted in to the box format like the above.
- The box format is repersented in Rows(1,2,3,4,5) and Columns(1,2,3,4,5).
Step-3:
- Let’s assume n=5(required number of rows).
- In the above image, the blue color area representing the Line(row), the grey color area representing the Space printing, and the green color area representing the Star printing.
- As we have seen already the Line can be represented either in increasing order(1,2,3,4,5) or in decreasing order(5,4,3,2,1).
- In the pattern, first-line is printing 4 spaces, second-line is printing 3 spaces, third-line is printing 2 spaces, and so on. Now look at gray area we have mentioned it like 1 to 4, 1 to 3, 1 to 2, 1 to 1, and 1 to 0. Now just understand here, 4 can be written as 5-1 means 4=5-1, that’s why you can see in the first line we have written it like 1 to 4 = 5 – 1, similarly we can write for the reamining lines.
- In the pattern, first-line is printing 1 star, second-line printing 2 stars, third-line printing 3 stars, and so on. Now you can see in the green area, we have mentioned it like 1 to 1, 1 to 2, 1 to 3, 1 to 4, and 1 to 5.
- Now match the common patterns from all(blue area, grey area, and green area), here you can see we have matched it with red color box.
- Now as we have selected lines in increasing order(1,2,3,4,5), so we can write it like 1 to n and then it can be further assumed as the variable i.
- In grey area, we can write 1 to ( n-i ) and then it can be further assumed as the variable j.
- Similarly in the green area, we can write it like 1 to i and then it can be further assumed as the vriable k.
- Now we can write a for loop for the variable i is like for(int i=1; i<=n; i++){ …} to change the line and a for loop for the variable j is like for(int j=1; j<=( n-i ); j++){ …} to print the spaces, and another for loop for the variable k is like for(int k=1; k<=i; k++){ … } to print the stars. Hence, here we required three for loops to print the given pattern.
Let’s see the complete logic below:
package com.javacodepoint.patterns;
public class StarPattern {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star printing
for(int k=1; k<=i; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Trick-4
![Star pattern programs [Tricks] in java 2 4. min](https://javacodepoint.com/wp-content/uploads/2021/08/4.-min.png)
Follow the below steps to develop the logic:
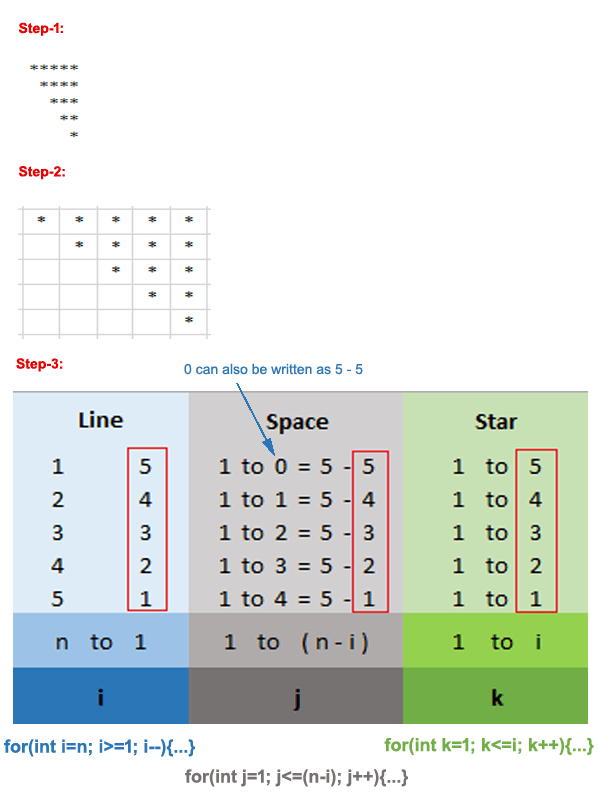
Let me explain the above steps:
Step-1:
- Given pattern
Step-2:
- The given pattern can be converted in to the box format like the above.
- The box format is repersented in Rows(1,2,3,4,5) and Columns(1,2,3,4,5).
Step-3:
- Let’s assume n=5(required number of rows).
- In the above image, the blue color area representing the Line(row), the grey color area representing the Space printing, and the green color area representing the Star printing.
- As we have seen already the Line can be represented either in increasing order(1,2,3,4,5) or in decreasing order(5,4,3,2,1).
- In the pattern, first-line is printing 0 space, second-line is printing 1 space, third-line is printing 2 spaces, and so on. Now look at gray area we have mentioned it like 1 to 0, 1 to 1, 1 to 2, 1 to 3, and 1 to 4. Now just understand here, 0 can be written as 5-5 means 0=5-5, that’s why you can see in the first line we have written it like 1 to 0 = 5 – 5, similarly we can write for the reamining lines.
- In the pattern, first-line is printing 5 star, second-line printing 4 stars, third-line printing 3 stars, and so on. Now you can see in the green area, we have mentioned it like 1 to 5, 1 to 4, 1 to 3, 1 to 2, and 1 to 1.
- Now match the common patterns from all(blue area, grey area, and green area), here you can see we have matched it with red color box.
- Now as we have selected lines in decreasing order(5,4,3,2,1), so we can write it like n to 1 and then it can be further assumed as the variable i.
- In grey area, we can write 1 to ( n-i ) and then it can be further assumed as the variable j.
- Similarly in the green area, we can write it like 1 to i and then it can be further assumed as the vriable k.
- Now we can write a for loop for the variable i is like for(int i=n; i>=1; i–){ …} to change the line and a for loop for the variable j is like for(int j=1; j<=( n-i ); j++){ …} to print the spaces, and another for loop for the variable k is like for(int k=1; k<=i; k++){ … } to print the stars. Hence, here also we required three for loops to print the given pattern.
Let’s see the complete logic below:
package com.javacodepoint.patterns;
public class StarPattern {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop for the star printing
for(int k=1; k<=i; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Trick-5
![Star pattern programs [Tricks] in java 3 9. min](https://javacodepoint.com/wp-content/uploads/2021/08/9.-min.png)
Follow the below steps to develop the logic:
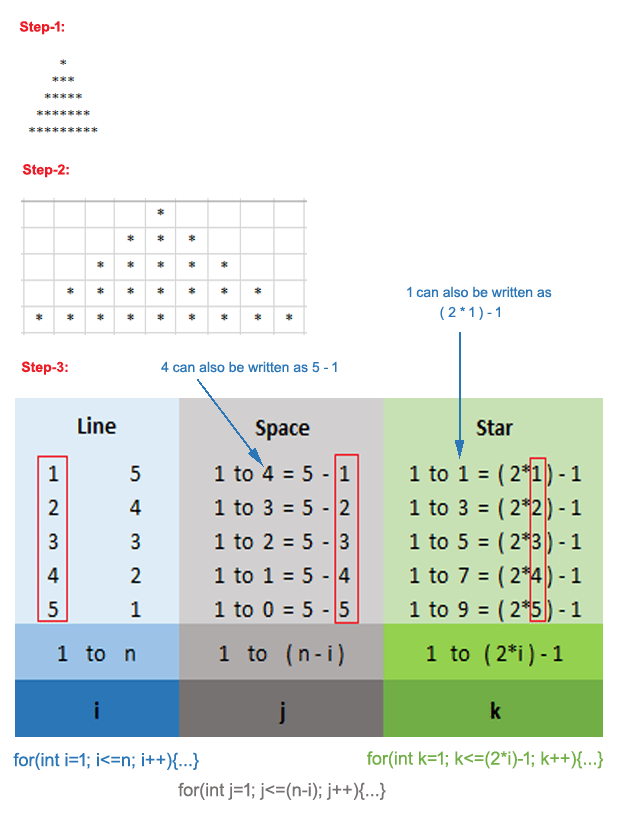
Let me explain the above steps:
Step-1:
- Given pattern
Step-2:
- The given pattern can be converted in to the box format like the above.
- The box format is repersented in Rows(1,2,3,4,5) and Columns(1,2,3,4,5).
Step-3:
- Let’s assume n=5(required number of rows).
- In the above image, the blue color area representing the Line(row), the grey color area representing the Space printing, and the green color area representing the Star printing.
- As we have seen already the Line can be represented either in increasing order(1,2,3,4,5) or in decreasing order(5,4,3,2,1).
- In the pattern, first-line is printing 4 spaces, second-line is printing 3 spaces, third-line is printing 2 spaces, and so on. Now look at gray area we have mentioned it like 1 to 4, 1 to 3, 1 to 2, 1 to 1, and 1 to 0. Now just understand here, 4 can be written as 5-1 means 4=5-1, that’s why you can see in the first line we have written it like 1 to 4 = 5 – 1, similarly we can write for the reamining lines.
- In the pattern, first-line is printing 1 star, second-line printing 3 stars, third-line printing 5 stars, and so on. Now you can see in the green area, we have mentioned it like 1 to 1, 1 to 3, 1 to 5, 1 to 7, and 1 to 9. Now understand again here, 1 can also be written as (2*1)-1, that’s why you can see in the first line we have written it like 1 to 1 = (2*1) – 1, similarly we can write for the reamining lines.
- Now match the common patterns from all(blue area, grey area, and green area), here you can see we have matched it with red color box.
- Now as we have selected lines in increasing order(1,2,3,4,5), so we can write it like 1 to n and then it can be further assumed as the variable i.
- In grey area, we can write 1 to ( n-i ) and then it can be further assumed as the variable j.
- Similarly in the green area, we can write it like 1 to (2*i)-1 and then it can be further assumed as the vriable k.
- Now we can write a for loop for the variable i is like for(int i=1; i<=n; i++){ …} to change the line and a for loop for the variable j is like for(int j=1; j<=( n-i ); j++){ …} to print the spaces, and another for loop for the variable k is like for(int k=1; k<=(2*i)-1; k++){ … } to print the stars. Hence, here also we required three for loops to print the given pattern.
Let’s see the complete logic below:
package com.javacodepoint.patterns;
public class StarPattern {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Trick-6
![Star pattern programs [Tricks] in java 4 10. min](https://javacodepoint.com/wp-content/uploads/2021/08/10.-min.png)
Follow the below steps to develop the logic:
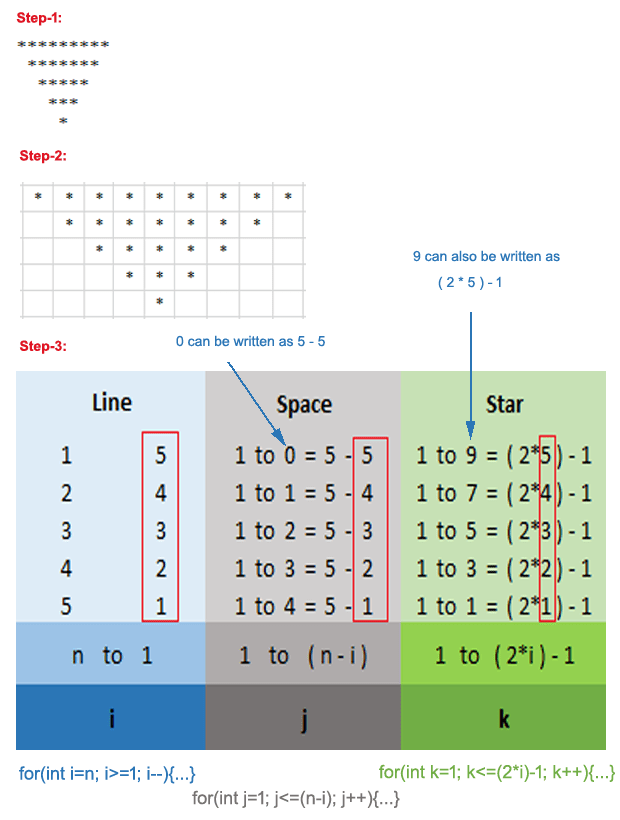
Let me explain the above steps:
Step-1:
- Given pattern
Step-2:
- The given pattern can be converted in to the box format like the above.
- The box format is repersented in Rows(1,2,3,4,5) and Columns(1,2,3,4,5).
Step-3:
- Let’s assume n=5(required number of rows).
- In the above image, the blue color area representing the Line(row), the grey color area representing the Space printing, and the green color area representing the Star printing.
- As we have seen already the Line can be represented either in increasing order(1,2,3,4,5) or in decreasing order(5,4,3,2,1).
- In the pattern, first-line is printing 0 space, second-line is printing 1 space, third-line is printing 2 spaces, and so on. Now look at gray area we have mentioned it like 1 to 0, 1 to 1, 1 to 2, 1 to 3, and 1 to 4. Now just understand here, 4 can be written as 5-1 means 4=5-1, that’s why you can see in the first line we have written it like 1 to 4 = 5 – 1, similarly we can write for the reamining lines.
- In the pattern, first-line is printing 9 stars, second-line printing 7 stars, third-line printing 5 stars, and so on. Now you can see in the green area, we have mentioned it like 1 to 9, 1 to 7, 1 to 5, 1 to 3, and 1 to 1. Now understand again here, 9 can also be written as (2*5)-1, that’s why you can see in the first line we have written it like 1 to 9 = (2*5) – 1, similarly we can write for the reamining lines.
- Now match the common patterns from all(blue area, grey area, and green area), here you can see we have matched it with red color box.
- Now as we have selected lines in decreasing order(5,4,3,2,1), so we can write it like n to 1 and then it can be further assumed as the variable i.
- In grey area, we can write 1 to ( n-i ) and then it can be further assumed as the variable j.
- Similarly in the green area, we can write it like 1 to (2*i)-1 and then it can be further assumed as the vriable k.
- Now we can write a for loop for the variable i is like for(int i=n; i>=1; i–){ …} to change the line and a for loop for the variable j is like for(int j=1; j<=( n-i ); j++){ …} to print the spaces, and another for loop for the variable k is like for(int k=1; k<=(2*i)-1; k++){ … } to print the stars. Hence, here also we required three for loops to print the given pattern.
Let’s see the complete logic below:
package com.javacodepoint.patterns;
public class StarPattern {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop for the star printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Trick-7
![Star pattern programs [Tricks] in java 5 13. min](https://javacodepoint.com/wp-content/uploads/2021/08/13.-min.png)
Follow the below steps to develop the logic:
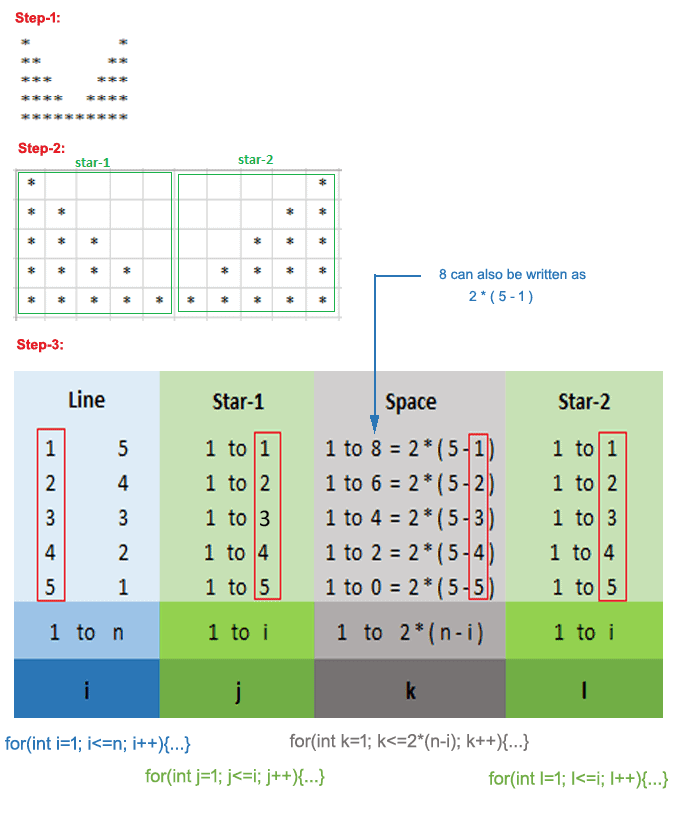
Let me explain the above steps:
Step-1:
- Given pattern
Step-2:
- The given pattern can be converted in to the box format. We have divided the pattern into two parts(star-1 and star-2) as you can see in the above image.
- The box format is repersented in Rows and Columns.
Step-3:
- Let’s assume n=5.
- In the above image, the blue color area representing the Line(row), the grey color area representing the Space printing, and the two green color area representing the Star-1 and Star-2 for printing stars.
- Line can be represented either in increasing order(1,2,3,4,5) or in decreasing order(5,4,3,2,1).
- Here we have divided the pattern into two parts(only for star printing). Now as you can see in both Star-1 and Star-2 area, first-line is printing 1 star, second-line is printing 2 stars, third-line is printing 3 stars, and so on. Now look at both green area we have mentioned it like 1 to 1, 1 to 2, 1 to 3, 1 to 4, and 1 to 5.
- Now look at grey area, first-line is printing 8 spaces, second-line printing 6 spaces, third-line printing 4 spaces, and so on. So we have mentioned it like 1 to 8, 1 to 6, 1 to 4, 1 to 2, and 1 to 0. Now understand here, 8 can also be written as 2*(5-1), that’s why in the first line we have written it like 1 to 8 = 2*(5-1), similarly we have written for the reamining lines.
- Now match the common patterns from all(blue area, grey area, and both green area), here you can see we have matched it with red color box.
- Now as we have selected lines in increasing order(1,2,3,4,5), so we can write it like 1 to n and then it can be further assumed as the variable i.
- Now in both green area, we can write 1 to i and then further we can assume as the variable j and l respectively.
- Now in grey area, we can write 1 to 2*( n-i ) and then it can be further assumed as the variable k.
- Now here we required 4 for loops(for the variable i,j,k,l) to print the given pattern.
- The loop for the variable i is like for(int i=1; i<=n; i++){ … }.
- The loop for the variable j is like for(int j=1; j<=i; j++){ … }.
- The loop for the variable k is like for(int k=1; k<=2*(n-i); k++){ … }.
- The loop for the variable l is like for(int l=1; l<=i; l++){ … }.
Let’s see the complete logic below:
package com.javacodepoint.patterns;
public class StarPattern {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Inner loop2 for the space printing
for(int k=1; k<=2*(n-i); k++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop3 for the again star printing
for(int l=1; l<=i; l++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Trick-8
![Star pattern programs [Tricks] in java 6 14. min](https://javacodepoint.com/wp-content/uploads/2021/08/14.-min.png)
Follow the below steps to develop the logic:
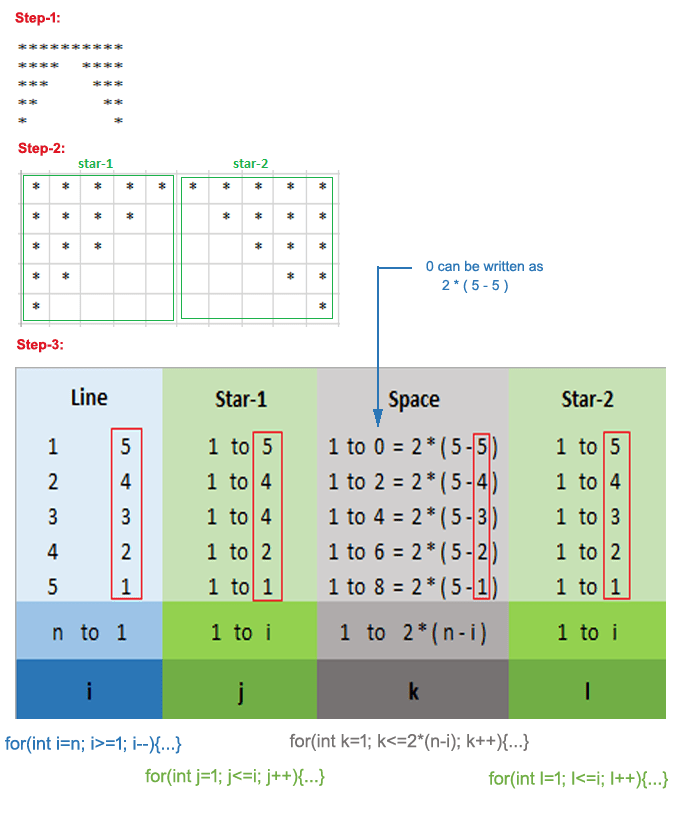
Let me explain the above steps:
Step-1:
- Given pattern
Step-2:
- The given pattern can be converted in to the box format. We have divided the pattern into two parts(star-1 and star-2) as you can see in the above image.
- The box format is repersented in Rows and Columns.
Step-3:
- Let’s assume n=5.
- In the above image, the blue color area representing the Line(row), the grey color area representing the Space printing, and the two green color area representing the Star-1 and Star-2 for printing stars.
- Line can be represented either in increasing order(1,2,3,4,5) or in decreasing order(5,4,3,2,1).
- Here we have divided the pattern into two parts(only for star printing). Now as you can see in both Star-1 and Star-2 area, first-line is printing 5 stars, second-line is printing 4 stars, third-line is printing 3 stars, and so on. Now look at both green area we have mentioned it like 1 to 5, 1 to 4, 1 to 3, 1 to 2, and 1 to 1.
- Now look at grey area, first-line is printing 8 spaces, second-line printing 6 spaces, third-line printing 4 spaces, and so on. So we have mentioned it like 1 to 8, 1 to 6, 1 to 4, 1 to 2, and 1 to 0. Now understand here, 8 can also be written as 2*(5-1), that’s why in the first line we have written it like 1 to 8 = 2*(5-1), similarly we have written for the reamining lines.
- Now match the common patterns from all(blue area, grey area, and both green area), here you can see we have matched it with red color box.
- Now as we have selected lines in decreasing order(5,4,3,2,1), so we can write it like n to 1 and then it can be further assumed as the variable i.
- Now in both green area, we can write 1 to i and then further we can assume as the variable j and l respectively.
- Now in grey area, we can write 1 to 2*( n-i ) and then it can be further assumed as the variable k.
- Now here also we required 4 for loops(for the variable i,j,k,l) to print the given pattern.
- The loop for the variable i is like for(int i=n; i>=1; i–){ … }.
- The loop for the variable j is like for(int j=1; j<=i; j++){ … }.
- The loop for the variable k is like for(int k=1; k<=2*(n-i); k++){ … }.
- The loop for the variable l is like for(int l=1; l<=i; l++){ … }.
Let’s see the complete logic below:
package com.javacodepoint.patterns;
public class StarPattern {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Inner loop2 for the space printing
for(int k=1; k<=2*(n-i); k++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop3 for the again star printing
for(int l=1; l<=i; l++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Uses of Tricks for other patterns
You have seen the 8 basic tricks to develop the logic for printing the patterns. Now, these tricks will help you to develop other types of pattern logic as well. Let’s see some of them for understanding:
Example-1
![Star pattern programs [Tricks] in java 7 5. 1](https://javacodepoint.com/wp-content/uploads/2021/08/5.-1.png)
To develop the logic for this, we required Trick-1 and Trick-2
Given Pattern Logic = Trick-1 + Trick-2
Let’s understand this with some simple steps:
Step-1:
First of all, convert this pattern into a box format(rows & columns) like the below image-
![Star pattern programs [Tricks] in java 8 5 min 2](https://javacodepoint.com/wp-content/uploads/2021/08/5-min-2.png)
Step-2:
When we combine Trick-1 & Trick-2 patterns, we are getting one extra row in the middle of the pattern(represented by the red box). Here we can divide the pattern into two parts upper half & lower half. For the upper half, we will use Tick-1 and for the lower half, we will use Trick-2 to develop the logic.
Step-3:
While developing the logic, from the lower half we will skip the first row/line star printing. So in order to skip first row/line printing, we will initialize the loop count by decreasing it by 1.
Let’s see the complete logic for this pattern below:-
package com.javacodepoint.patterns;
public class StarPattern {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
// Lower half
// Outer loop for the line/row change
for(int i=(n-1); i>=1; i--) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Example-2
![Star pattern programs [Tricks] in java 9 6. min 1](https://javacodepoint.com/wp-content/uploads/2021/08/6.-min-1.png)
To develop the logic for this also, we required the Trick-1 and Trick-2
Given Pattern Logic = Trick-2 + Trick-1
Convert the pattern into a box format(rows & columns) like the below image-
![Star pattern programs [Tricks] in java 10 6 min 1](https://javacodepoint.com/wp-content/uploads/2021/08/6-min-1.png)
Here everything will be the same as Example-1 but the only difference is Trick-2 logic should come first then Trick-1 logic.
Let’s see the complete logic for this pattern below:-
package com.javacodepoint.patterns;
public class StarPattern {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
// Lower half
// Outer loop for the line/row change
for(int i=2; i<=n; i++) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Example-3
![Star pattern programs [Tricks] in java 11 7. min 1](https://javacodepoint.com/wp-content/uploads/2021/08/7.-min-1.png)
To develop the logic for this, we required Trick-3 and Trick-4
Given Pattern Logic = Trick-3 + Trick-4
Let’s understand this with some simple steps:
Step-1:
First of all, convert this pattern into a box format(rows & columns) like the below image-
![Star pattern programs [Tricks] in java 12 7 min 2](https://javacodepoint.com/wp-content/uploads/2021/08/7-min-2.png)
Here also when we combine Trick-3 & Trick-4 patterns, we will get one extra row in the middle of the pattern(represented by the red box). The pattern is divided into two parts upper half & lower half. For the upper half, we will use Tick-3 and for the lower half, we will use Trick-4 to develop the logic.
Step-2:
While developing the logic, from the lower half we will skip the first row/line star printing. So in order to skip first row/line printing, we will initialize the loop count by decreasing 1.
See the complete logic below-
package com.javacodepoint.patterns;
public class StarPattern {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star printing
for(int k=1; k<=i; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
// Lower half
// Outer loop for the line/row change
for(int i=(n-1); i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star printing
for(int k=1; k<=i; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Example-4
![Star pattern programs [Tricks] in java 13 11. min](https://javacodepoint.com/wp-content/uploads/2021/08/11.-min.png)
To develop the logic for this, we required Trick-5 and Trick-6
Given Pattern Logic = Trick-5 + Trick-6
Let’s convert this pattern into a box format(rows & columns) like the below image-
![Star pattern programs [Tricks] in java 14 11 min](https://javacodepoint.com/wp-content/uploads/2021/08/11-min.png)
Here also when we combine Trick-5 & Trick-6 patterns, we will get one extra row(represented by the red box). The pattern is divided into two parts upper half & lower half. For the upper half, we will use Tick-5 and for the lower half, we will use Trick-6 to develop the logic. Now while developing the logic, from the lower half we need to skip the first row/line star printing. So we should initialize the loop count by decreasing 1.
See the complete logic below-
package com.javacodepoint.patterns;
public class StarPattern {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
// Lower half
// Outer loop for the line/row change
for(int i=n-1; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop for the star printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Example-5
![Star pattern programs [Tricks] in java 15 12. min](https://javacodepoint.com/wp-content/uploads/2021/08/12.-min.png)
To develop the logic for this also, we required Trick-5 and Trick-6 only
Given Pattern Logic = Trick-6 + Trick-5
Let’s convert this pattern into a box format(rows & columns) like the below image-
![Star pattern programs [Tricks] in java 16 12 min](https://javacodepoint.com/wp-content/uploads/2021/08/12-min.png)
Here everything will be the same as Example-4 but the only difference is Trick-6 logic should come first then Trick-5 logic.
See the complete logic below-
package com.javacodepoint.patterns;
public class StarPattern {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop for the star printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
// Lower half
// Outer loop for the line/row change
for(int i=2; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Example-6
![Star pattern programs [Tricks] in java 17 15. min](https://javacodepoint.com/wp-content/uploads/2021/08/15.-min.png)
To develop the logic for this, we required Trick-7 and Trick-8
Given Pattern Logic = Trick-7 + Trick-8
Let’s convert this pattern into a box format(rows & columns) like the below image-
![Star pattern programs [Tricks] in java 18 15 min](https://javacodepoint.com/wp-content/uploads/2021/08/15-min.png)
Here also when we combine Trick-7 & Trick-8 patterns, we will get one extra row(represented by the red box). The pattern is divided into two parts upper half & lower half. For the upper half, we will use Tick-7 and for the lower half, we will use Trick-8 to develop the logic. Now while developing the logic, from the lower half we need to skip the first row/line star printing. So we should initialize the loop count by decreasing 1.
See the complete logic below-
package com.javacodepoint.patterns;
public class StarPattern {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Inner loop2 for the space printing
for(int k=1; k<=2*(n-i); k++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop3 for the again star printing
for(int l=1; l<=i; l++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
// Lower half (skip the first line printing)
// Outer loop for the line/row change
for(int i=n-1; i>=1; i--) {
// Inner loop1 for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Inner loop2 for the space printing
for(int k=1; k<=2*(n-i); k++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop3 for the again star printing
for(int l=1; l<=i; l++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Conclusion
In this article, you have seen 8 simple pattern printing tricks with a clear explanation. You have also seen that with the help of these tricks you can able to develop logic for other pattern printing programs.
With the help of these tricks, you can also develop the logic for Character Pattern programs and Number Pattern programs.
You can learn top 30 star pattern programs logic here: Top 30 star pattern programs in java