In this article, you will learn Binary to decimal conversion using Java. Here you will see multiple ways to convert a binary number into a decimal equivalent number in Java.
For example-
Binary number 101 => 5 (decimal equivalent)
Binary number 1111 => 15 (decimal equivalent)
How to convert binary to decimal?
Binary to Decimal conversion is very simple, look into the below diagram for conversion in the steps:
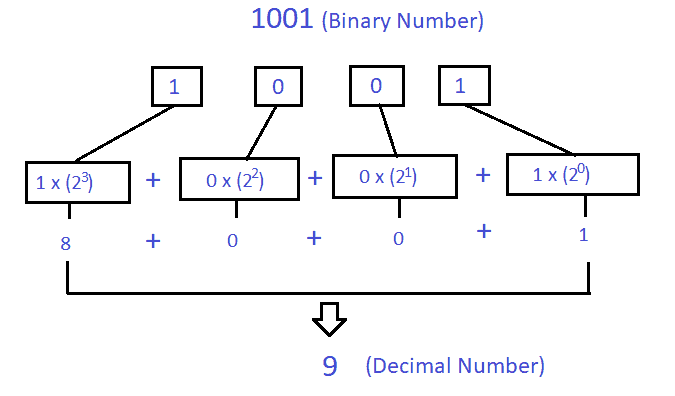
Java Program to Convert Binary to Decimal
Here, we will see multiple java programs for binary-to-decimal conversion in Java.
- Binary to Decimal – if the binary number is in integer format
- Binary to Decimal – if the binary number is in string format
- Binary to Decimal using an inbuilt function
- Binary to Decimal using Scanner
- Binary to Decimal using Recursion
Binary to Decimal conversion in Java – if the binary number is in integer format
package com.javacodepoint.conversion;
/**
* Binary to Decimal conversion example, if binary number is in integer form.
*/
public class BinaryToDecimalExample1 {
// method for binary to decimal
public static int binaryToDecimal(int binary) {
int decimal = 0;
// Initialize base to 1, i.e 2^0
int base = 1;
while (binary > 0) {
int lastDigit = binary % 10;
decimal += lastDigit * base;
base = base * 2;
// remove the last digit
binary = binary / 10;
}
return decimal;
}
// main method to test
public static void main(String[] args) {
System.out.println("Decimal Number of 1001 is: " + binaryToDecimal(1001));
System.out.println("Decimal Number of 1111 is: " + binaryToDecimal(1111));
System.out.println("Decimal Number of 1100110 is: " + binaryToDecimal(1100110));
System.out.println("Decimal Number of 1000000001 is: " + binaryToDecimal(1000000001));
}
}
OUTPUT:
Decimal Number of 1001 is: 9
Decimal Number of 1111 is: 15
Decimal Number of 1100110 is: 102
Decimal Number of 1000000001 is: 513
Binary to Decimal conversion in Java – if the binary number is in string format
package com.javacodepoint.conversion;
/**
* Binary to Decimal conversion example, if binary number is in string form.
*/
public class BinaryToDecimalExample2 {
// method for binary to decimal
public static int binaryToDecimal(String binary) {
int decimal = 0;
// initialize base to 1, i.e 2^0
int base = 1;
// loop the binary string in reverse way
for (int i = binary.length() - 1; i >= 0; i--) {
if (binary.charAt(i) == '1') {
decimal += base;
}
// each time base will become doubled
base = base * 2;
}
return decimal;
}
// main method to test
public static void main(String[] args) {
System.out.println("Decimal Number of 1001 is: " + binaryToDecimal("1001"));
System.out.println("Decimal Number of 1111 is: " + binaryToDecimal("1111"));
System.out.println("Decimal Number of 1100110 is: " + binaryToDecimal("1100110"));
System.out.println("Decimal Number of 1000000001 is: " + binaryToDecimal("1000000001"));
}
}
OUTPUT:
Decimal Number of 1001 is: 9
Decimal Number of 1111 is: 15
Decimal Number of 1100110 is: 102
Decimal Number of 1000000001 is: 513
Binary to Decimal in java inbuilt function
To convert a binary number to a decimal equivalent number in Java, there is an inbuild function provided by java parseInt() which is available in the java.lang.Integer
class.
public static int parseInt(String s, int radix) – Parses the string argument as a signed integer in the radix specified by the second argument. The characters in the string must all be digits of the specified radix (a nonnegative value), except that the first character may be an ASCII minus sign or plus sign.
For example-
parseInt(“0”, 10) returns 0
parseInt(“473”, 10) returns 473
parseInt(“+42”, 10) returns 42
parseInt(“-0”, 10) returns 0
parseInt(“-FF”, 16) returns -255
parseInt(“1100110”, 2) returns 102
BinaryToDecimalExample3.java (binary to decimal program using an inbuilt function)
package com.javacodepoint.conversion;
/**
* Binary to Decimal conversion example, using parseInt() method.
*/
public class BinaryToDecimalExample3 {
public static void main(String[] args) {
System.out.println("Decimal Number of 101 is: " + Integer.parseInt("101", 2));
System.out.println("Decimal Number of 1010101 is: " + Integer.parseInt("1010101", 2));
System.out.println("Decimal Number of 1000111 is: " + Integer.parseInt("1000111", 2));
System.out.println("Decimal Number of 1000000001 is: " + Integer.parseInt("1000000001", 2));
}
}
OUTPUT:
Decimal Number of 101 is: 5
Decimal Number of 1010101 is: 85
Decimal Number of 1000111 is: 71
Decimal Number of 1000000001 is: 513
Java program for Binary to Decimal using Scanner
In this example, we are reading a binary number from the user using the java.util.Scanner
class.
BinaryToDecimalExample4.java
package com.javacodepoint.conversion;
import java.util.Scanner;
/**
* Binary to Decimal conversion using Scanner.
*/
public class BinaryToDecimalExample4 {
// method to convert binary to decimal
public static int binaryToDecimal(int binary) {
int decimal = 0;
// Initialize base to 1, i.e 2^0
int base = 1;
while (binary > 0) {
int lastDigit = binary % 10;
decimal += lastDigit * base;
base = base * 2;
// remove the last digit
binary = binary / 10;
}
return decimal;
}
// main method to test
public static void main(String[] args) {
// Create scanner object
Scanner sc = new Scanner(System.in);
// read binary number from user
System.out.println("Enter a binary number:");
int binary = sc.nextInt();
System.out.println("Decimal Number of " + binary + " is: " + binaryToDecimal(binary));
}
}
OUTPUT:
Enter a binary number:
1010
Decimal Number of 1010 is: 10
Java program to convert Binary to Decimal using Recursion
Recursion in java is a technique in which a method calls itself continuously. A method in java that calls itself is called a recursive method. Below is a simple recursive method to convert a binary number into a decimal number.
// recursive method for binary to decimal
public static int binaryToDecimal(int binary) {
// if binary number become 0
if (binary == 0) {
return 0;
}
return (binary % 10 + 2 * binaryToDecimal(binary / 10));
}
Learn more about binary to decimal conversion using recursion in java, here: Binary to Decimal using Recursion in Java.