A Diamond Pattern is a geometric arrangement of characters, often represented by asterisks (*), forming the shape of a diamond. It consists of two parts: the upper half and the lower half. The upper half forms an upward-pointing triangle, while the lower half completes the diamond shape by forming a downward-pointing triangle.
Diamond patterns are created using nested loops to control the number of characters and spaces in each row. They are a popular programming exercise to practice nested loops and pattern printing. Diamond patterns can be customized by changing the number of rows and the character used.
Here is the Java program for it:
package com.javacodepoint.patterns;
public class DiamondPattern {
public static void main(String[] args) {
int rows = 5;
int spaces = rows - 1;
// Upper half of the diamond
for (int i = 1; i <= rows; i++) {
for (int j = 1; j <= spaces; j++) {
System.out.print(" ");
}
spaces--;
for (int k = 1; k <= 2 * i - 1; k++) {
System.out.print("*");
}
System.out.println();
}
spaces = 1;
// Lower half of the diamond
for (int i = 1; i <= rows - 1; i++) {
for (int j = 1; j <= spaces; j++) {
System.out.print(" ");
}
spaces++;
for (int k = 1; k <= 2 * (rows - i) - 1; k++) {
System.out.print("*");
}
System.out.println();
}
}
}
OUTPUT:
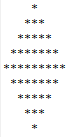
See also: Top 30-star pattern programs in Java.