In this blog post, you will learn how to write the ‘Hello world’ program, compile it, and execute it in java language. The ‘Hello world’ program is a very simple program that will print the ‘Hello world‘ on the screen.
Table of Contents
Required software dependency
The below-listed software is required for writing, compiling, and executing the ‘Hello world’ program in java.
- Text Editor: A text editor software required for writing the java program such as Notepad, Notepad++, EditPlus, etc.
- JDK (Java Development Kit): It is required to compile and execute the java programs.
- Command Prompt: It is used to execute the command, for example, javac, java, etc.
Note: Make sure your system should have JDK (Java Development Kit) software installed to compile and execute java programs. If not follow another article, JDK Installation on Windows.
How to write the Hello world program in Java?
You just have to follow the below simple steps:-
STEP-1: Open any text editor (for example- Notepad), and write the below code for the ‘Hello World!‘ program:
// my first java program
public class Test{
// main method
public static void main(String[] args) {
// It prints Hello World! on screen
System.out.println("Hello World!");
}
}
STEP-2: Now save it with the filename ‘Test.java‘ in any folder in your system. In our case, we are saving it in D:\java\programs\

STEP-3: Open command prompt. In order to open it, Press the Window key + R, you will see the below image, here type cmd and press enter key.
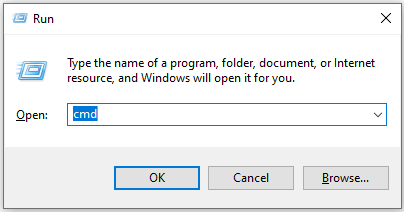
STEP-4: Once your command prompt open, go to your folder location where you have saved the Test.java file like the below image:
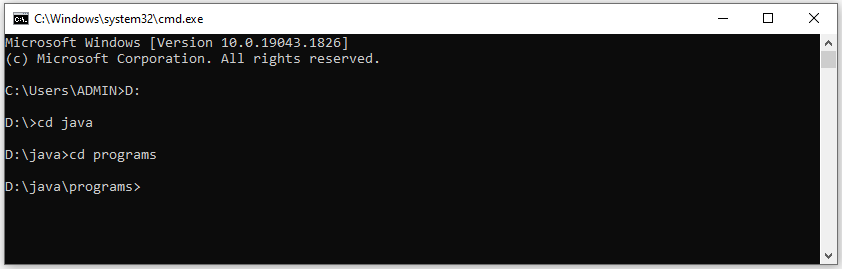
STEP-5: Now compile and execute the java program. To compile it run the command javac Test.java, and to execute it run the command java Test as below image:
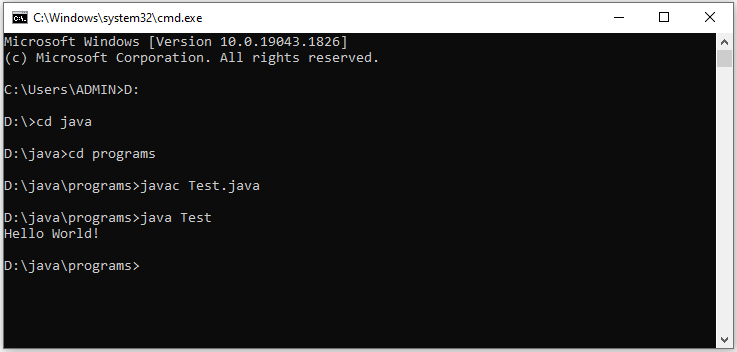
Java Hello world program Explanation
// My first java program
public class Test{
// main method
public static void main(String[] args) {
// It prints Hello World! on screen
System.out.println("Hello World!");
}
}
This Hello World program is explained below in detail:-
// My first java program
The above statement is a single-line comment (which starts with //) in java. Comments are intended for users reading the code to understand the intent and functionality of the program. It will tell the java compiler to ignore that line to compile.
class Test{
// ... ......
}
Every java application should start with a class definition. Here, Test is the name of the class.
public static void main(String args[]){
// ... ......
}
This is the main method. Every application in Java must contain the main method. The execution of the program starts from the main method only.
System.out.println("Hello World!");
The above statement is a print statement in java. It prints the text Hello World! here on the screen or console.
Java Hello world program in Eclipse IDE
The Eclipse IDE is one of the most popular IDE for developing java applications. It provides a single interface to write, compile, and execute java programs. It is very easy to use. But as a beginner java developer, it is not recommended to use because if you use a simple text editor instead of any IDE you will remember writing the correct syntaxes.
If you don’t have Eclipse IDE installed in your system, then download it from its official Website and install it, Download Eclipse IDE.
Steps to create java Hello world program in Eclipse
Step-1: Open Eclipse IDE, go to File menu > New > Project… > Java Project > click Next. Now give a project name. eg- ‘JavaProgrmas‘ as the below image-
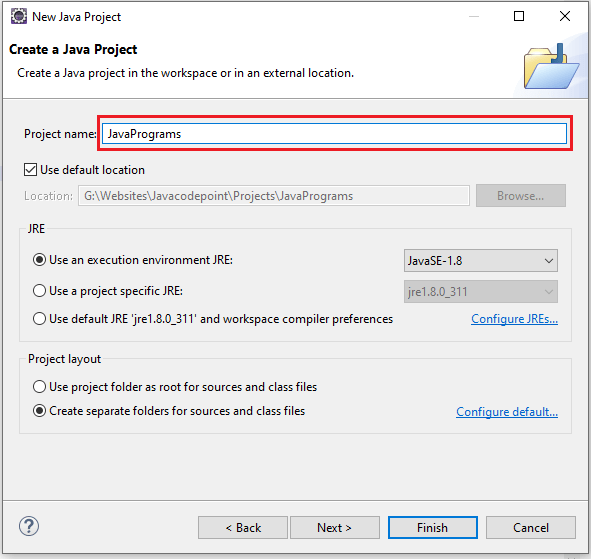
Now click on the Finish button to create the java project. Once it is created, you will see the below project structure got created.
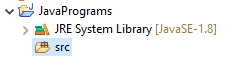
Step-2: Right-click on src > New > Package, then provide a package name. eg- com.javacodepoint.programs.
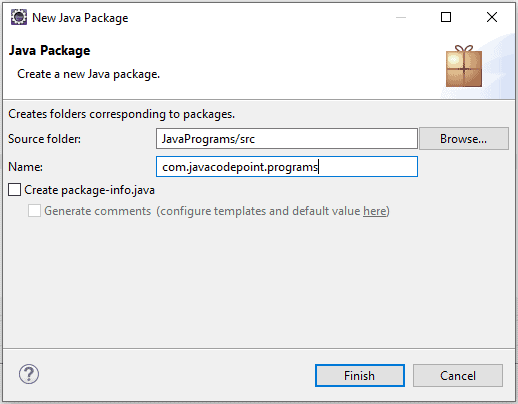
Step-3: Right-click on created package > New > Class, then give a class name. eg- Test.
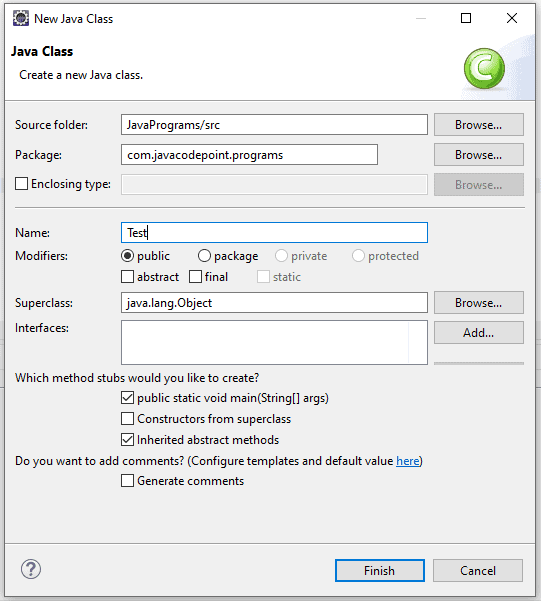
Step-4: Write the below code for the Hello world program in java:
package com.javacodepoint.programs;
// My first java program
public class Test {
// main method
public static void main(String[] args) {
// It prints Hello World! on the console
System.out.println("Hello World!");
}
}
Step-5: Run the program. Right-click on created ‘Test‘ class > Run As > Java Application, then you will see the program outputs on Console.
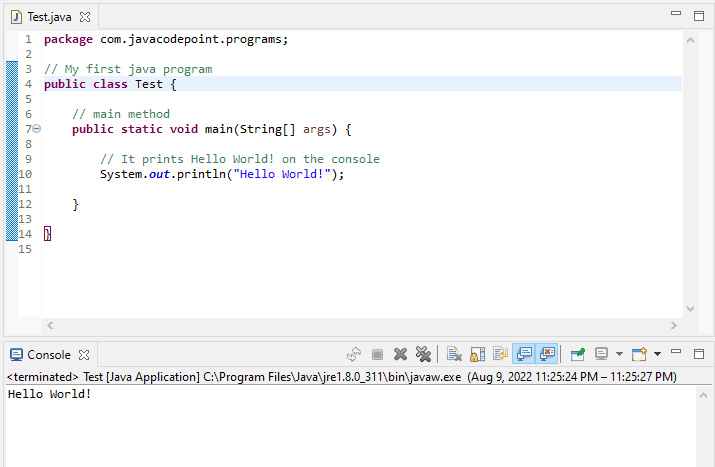
That’s all about creating and executing the Java Hello world program in Eclipse IDE.
Continue reading, Java Program to Add two Numbers.
This is very interesting, You are a very skilled blogger.
I have joined your feed and look forward to seeking more of your magnificent post.
Also, I have shared your web site in my social networks!