In this article, you will see the Top 10 number pattern programs in java. These pattern programs will give you a better understanding of the logic for the number patterns.
The pattern programs are practically based on mathematical logic and matrices’ fundamentals. We recommend you, learn simple tricks to develop the logic for pattern printing in another article here: Pattern Printing Tricks.
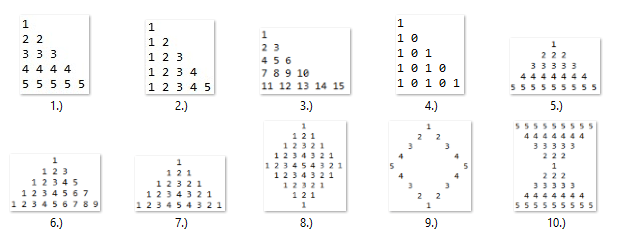
Let’s start understanding these patterns one by one.
Table of Contents
Pattern-1

Let’s see the java code to understand this pattern.
NumberPattern1.java
package com.javacodepoint.patterns;
public class NumberPattern1 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Outer loop for the line change
for(int i=1; i<=n; i++) {
// Inner loop for the number printing
for(int j=1; j<=i; j++) {
// Printing the number without changing the line
System.out.print(i+" ");
}
// Line change
System.out.println();
}
}
}
Pattern-2

Let’s see the java code to understand this pattern.
NumberPattern2.java
package com.javacodepoint.patterns;
public class NumberPattern2 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Outer loop for the line change
for(int i=1; i<=n; i++) {
// Inner loop for the number printing
for(int j=1; j<=i; j++) {
// Printing the number without changing the line
System.out.print(j+" ");
}
// Line change
System.out.println();
}
}
}
Pattern-3
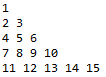
Let’s see the java code to understand this pattern.
NumberPattern3.java
package com.javacodepoint.patterns;
public class NumberPattern3 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Number to be printed
int num=1;
// Outer loop for the line change
for(int i=1; i<=n; i++) {
// Inner loop for the number printing
for(int j=1; j<=i; j++) {
// Printing the number without changing the line
System.out.print((num++)+" ");
}
// Line change
System.out.println();
}
}
}
Pattern-4

Let’s see the java code to understand this pattern.
NumberPattern4.java
package com.javacodepoint.patterns;
public class NumberPattern4 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Outer loop for the line change
for(int i=1; i<=n; i++) {
// Inner loop for the number printing
for(int j=1; j<=i; j++) {
// Printing the number without changing the line
if(j%2 == 0)
System.out.print("0 ");
else
System.out.print("1 ");
}
// Line change
System.out.println();
}
}
}
Pattern-5
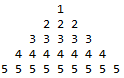
Let’s see the java code to understand this pattern.
NumberPattern5.java
package com.javacodepoint.patterns;
public class NumberPattern5 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Outer loop for the line change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the number printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the number without changing the line
System.out.print(i+" ");
}
// Line change
System.out.println();
}
}
}
Pattern-6
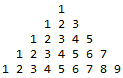
Let’s see the java code to understand this pattern.
NumberPattern6.java
package com.javacodepoint.patterns;
public class NumberPattern6 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Outer loop for the line change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the number printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the number without changing the line
System.out.print(k+" ");
}
// Line change
System.out.println();
}
}
}
Pattern-7
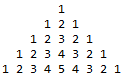
Let’s see the java code to understand this pattern.
NumberPattern7.java
package com.javacodepoint.patterns;
public class NumberPattern7 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Outer loop for the line change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the 2 space without changing the line
System.out.print(" ");
}
int num=0;
// Inner loop2 for the number printing
for(int k=1; k<=(2*i)-1; k++) {
if(k <= i)
// Printing first half by increasing the number
System.out.print((++num)+" ");
else
// Printing second half by decreasing the number
System.out.print((--num)+" ");
}
// Line change
System.out.println();
}
}
}
Pattern-8
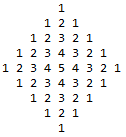
Let’s see the java code to understand this pattern.
NumberPattern8.java
package com.javacodepoint.patterns;
public class NumberPattern8 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Upper half
// Outer loop for the line change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the 2 spaces without changing the line
System.out.print(" ");
}
int num=0;
// Inner loop2 for the number printing
for(int k=1; k<=(2*i)-1; k++) {
if(k <= (2*i)/2 )
// Printing first half by increasing the number
System.out.print((++num)+" ");
else
// Printing second half by decreasing the number
System.out.print((--num)+" ");
}
// Line change
System.out.println();
}
// Lower half
// Outer loop for the line change
for(int i=n-1; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the 2 spaces without changing the line
System.out.print(" ");
}
int num=0;
// Inner loop2 for the number printing
for(int k=1; k<=(2*i)-1; k++) {
if(k <= (2*i)/2 )
// Printing first half by increasing the number
System.out.print((++num)+" ");
else
// Printing second half by decreasing the number
System.out.print((--num)+" ");
}
// Line change
System.out.println();
}
}
}
Pattern-9
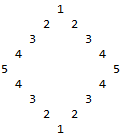
Let’s see the java code to understand this pattern.
NumberPattern9.java
package com.javacodepoint.patterns;
public class NumberPattern9 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Upper half
// Outer loop for the line change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the 2 spaces without changing the line
System.out.print(" ");
}
// Inner loop2 for the number printing
for(int k=1; k<=(2*i)-1; k++) {
if( k==1 || k== (2*i)-1 )
// Printing first & last number only
System.out.print(i+" ");
else
// Printing middle with spaces (Each times 2 spaces)
System.out.print(" ");
}
// Line change
System.out.println();
}
// Lower half
// Outer loop for the line change
for(int i=n-1; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the 2 spaces without changing the line
System.out.print(" ");
}
// Inner loop2 for the number printing
for(int k=1; k<=(2*i)-1; k++) {
if( k==1 || k== (2*i)-1 )
// Printing first & last number only
System.out.print(i+" ");
else
// Printing middle with spaces (Each times 2 spaces)
System.out.print(" ");
}
// Line change
System.out.println();
}
}
}
Pattern-10
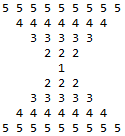
Let’s see the java code to understand this pattern.
NumberPattern10.java
package com.javacodepoint.patterns;
public class NumberPattern10 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Upper half
// Outer loop for the line change
for(int i=n; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the 2 spaces without changing the line
System.out.print(" ");
}
// Inner loop2 for the number printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the number without changing the line
System.out.print(i+" ");
}
// Line change
System.out.println();
}
// Lower half
// Outer loop for the line change
for(int i=2; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the 2 spaces without changing the line
System.out.print(" ");
}
// Inner loop2 for the number printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the number without changing the line
System.out.print(i+" ");
}
// Line change
System.out.println();
}
}
}
Conclusion
In this article, you have seen the top 10 Number pattern programs in java.