A hollow diamond pattern is a variation of the diamond pattern where the interior of the diamond is empty, creating a hollow appearance. In this pattern, the characters forming the outline of the diamond are typically represented by asterisks (*), and the interior is empty, represented by spaces.
Here’s an example of a hollow diamond pattern with 5 rows:
package com.javacodepoint.patterns;
public class HollowDiamondPattern {
public static void main(String[] args) {
int rows = 5;
int spaces = rows - 1;
// Upper half of the hollow diamond
for (int i = 1; i <= rows; i++) {
for (int j = 1; j <= spaces; j++) {
System.out.print(" ");
}
spaces--;
for (int k = 1; k <= 2 * i - 1; k++) {
if (k == 1 || k == 2 * i - 1) {
System.out.print("*");
} else {
System.out.print(" ");
}
}
System.out.println();
}
spaces = 1;
// Lower half of the hollow diamond
for (int i = 1; i <= rows - 1; i++) {
for (int j = 1; j <= spaces; j++) {
System.out.print(" ");
}
spaces++;
for (int k = 1; k <= 2 * (rows - i) - 1; k++) {
if (k == 1 || k == 2 * (rows - i) - 1) {
System.out.print("*");
} else {
System.out.print(" ");
}
}
System.out.println();
}
}
}
OUTPUT:
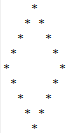
In this pattern, the first and last rows have one asterisk each, representing the top and bottom points of the diamond. The second and fourth rows have two asterisks each, and the third row has three asterisks. The interior of the diamond is empty, represented by spaces.
See also: Top 30-star pattern programs in Java.