In this article, you will see the top 30-star pattern program in Java. Here we tried to provide the logic with a clear explanation for the Java professional. Pattern programs are frequently asked in the fresher interview to check their logical skills.
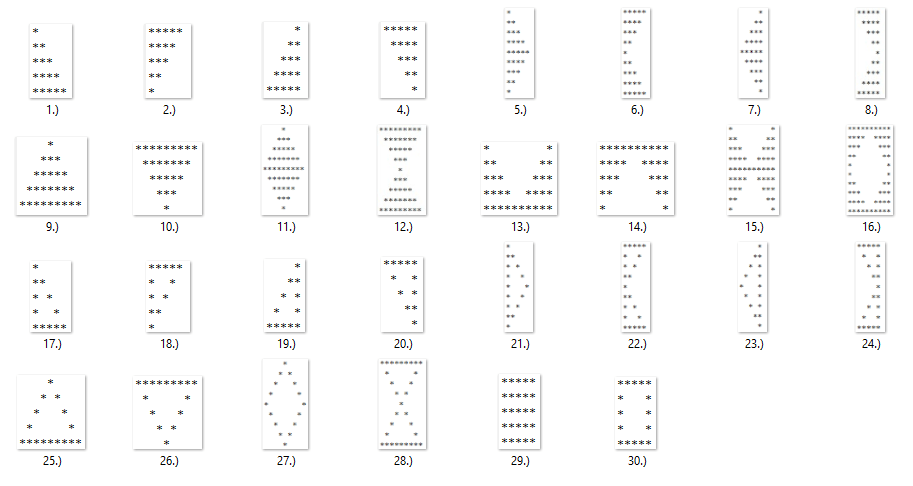
Before going through the logic for all these pattern programs, we recommend you learn some basic tricks to develop the logic. Tricks will help you to develop the logic for Alphabet/Character pattern, and Number pattern programs also.
Visit the link: Star pattern programs [Tricks] in Java.
Let us see the programs for all the above patterns one by one.
Table of Contents
Pattern-1 (Right Triangle)

StarPattern1.java
package com.javacodepoint.patterns;
public class StarPattern1 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-2 (Down Right Triangle)

StarPattern2.java
package com.javacodepoint.patterns;
public class StarPattern2 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-3 (Mirrored Right Triangle)

StarPattern3.java
package com.javacodepoint.patterns;
public class StarPattern3 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star printing
for(int k=1; k<=i; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-4 (Down Mirrored Right Triangle)

StarPattern4.java
package com.javacodepoint.patterns;
public class StarPattern4 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop for the star printing
for(int k=1; k<=i; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-5 (Right Pascal’s Triangle)
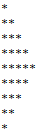
StarPattern5.java
package com.javacodepoint.patterns;
public class StarPattern5 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
// Lower half
// Outer loop for the line/row change
for(int i=(n-1); i>=1; i--) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-6 (K Shape Pattern)
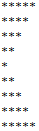
StarPattern6.java
package com.javacodepoint.patterns;
public class StarPattern6 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
// Lower half
// Outer loop for the line/row change
for(int i=2; i<=n; i++) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-7 (Mirrored Right Pascal’s Triangle)
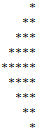
StarPattern7.java
package com.javacodepoint.patterns;
public class StarPattern7 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star printing
for(int k=1; k<=i; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
// Lower half
// Outer loop for the line/row change
for(int i=(n-1); i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star printing
for(int k=1; k<=i; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-8 (Reverse K Shape Pattern)
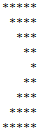
StarPattern8.java
package com.javacodepoint.patterns;
public class StarPattern8 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// First half
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star printing
for(int k=1; k<=i; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
// Second half
// Outer loop for the line/row change
for(int i=2; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star printing
for(int k=1; k<=i; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-9 (Pyramid Pattern)

StarPattern9.java
package com.javacodepoint.patterns;
public class StarPattern9 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-10 (Reversed Pyramid Pattern)

StarPattern10.java
package com.javacodepoint.patterns;
public class StarPattern10 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop for the star printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-11 (Diamond Star Pattern)
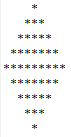
StarPattern11.java
package com.javacodepoint.patterns;
public class StarPattern11 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
// Lower half
// Outer loop for the line/row change
for(int i=n-1; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop for the star printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-12 (Sandglass Star Pattern)
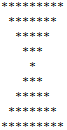
StarPattern12.java
package com.javacodepoint.patterns;
public class StarPattern12 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop for the star printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
// Lower half
// Outer loop for the line/row change
for(int i=2; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-13

StarPattern13.java
package com.javacodepoint.patterns;
public class StarPattern13 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Inner loop2 for the space printing
for(int k=1; k<=2*(n-i); k++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop3 for the again star printing
for(int l=1; l<=i; l++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-14

StarPattern14.java
package com.javacodepoint.patterns;
public class StarPattern14 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Inner loop2 for the space printing
for(int k=1; k<=2*(n-i); k++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop3 for the again star printing
for(int l=1; l<=i; l++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-15 (H Shape Pattern)
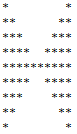
StarPattern15.java
package com.javacodepoint.patterns;
public class StarPattern15 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Inner loop2 for the space printing
for(int k=1; k<=2*(n-i); k++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop3 for the again star printing
for(int l=1; l<=i; l++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
// Lower half (skip the first line printing)
// Outer loop for the line/row change
for(int i=n-1; i>=1; i--) {
// Inner loop1 for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Inner loop2 for the space printing
for(int k=1; k<=2*(n-i); k++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop3 for the again star printing
for(int l=1; l<=i; l++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-16
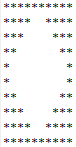
StarPattern16.java
package com.javacodepoint.patterns;
public class StarPattern16 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Inner loop2 for the space printing
for(int k=1; k<=2*(n-i); k++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop3 for the again star printing
for(int l=1; l<=i; l++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
// Lower half
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Inner loop2 for the space printing
for(int k=1; k<=2*(n-i); k++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop3 for the again star printing
for(int l=1; l<=i; l++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-17 (Empty Right Triangle)

StarPattern17.java
package com.javacodepoint.patterns;
public class StarPattern17 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop for the star and space printing
for(int j=1; j<=i; j++) {
if( j == 1 || j == i || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-18 (Down Empty Right Triangle)

StarPattern18.java
package com.javacodepoint.patterns;
public class StarPattern18 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop for the star and space printing
for(int j=1; j<=i; j++) {
if( j == 1 || j == i || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-19 (Mirrored Empty Right Triangle)

StarPattern19.java
package com.javacodepoint.patterns;
public class StarPattern19 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=i; k++) {
if( k == 1 || k == i || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-20 (Down Mirrored Empty Right Triangle)

StarPattern20.java
package com.javacodepoint.patterns;
public class StarPattern20 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=i; k++) {
if( k == 1 || k == i || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-21 (Empty Pascal’s Triangle)
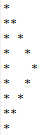
StarPattern21.java
package com.javacodepoint.patterns;
public class StarPattern21 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
if( j == 1 || j == i ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
// Lower half (skip the first line to print)
// Outer loop for the line/row change
for(int i=n-1; i>=1; i--) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
if( j == 1 || j == i || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-22
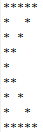
StarPattern22.java
package com.javacodepoint.patterns;
public class StarPattern22 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
if( j == 1 || j == i || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
// Lower half (skip the first line to print)
// Outer loop for the line/row change
for(int i=2; i<=n; i++) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
if( j == 1 || j == i || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-23 (Mirrored Empty Pascal’s Triangle)
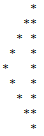
StarPattern23.java
package com.javacodepoint.patterns;
public class StarPattern23 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=i; k++) {
if( k == 1 || k == i ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
// Lower half
// Outer loop for the line/row change
for(int i=(n-1); i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=i; k++) {
if( k == 1 || k == i || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-24
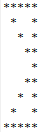
StarPattern24.java
package com.javacodepoint.patterns;
public class StarPattern24 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=i; k++) {
if( k == 1 || k == i || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
// Lower half
// Outer loop for the line/row change
for(int i=2; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=i; k++) {
if( k == 1 || k == i || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-25 (Empty Triangle)

StarPattern25.java
package com.javacodepoint.patterns;
public class StarPattern25 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=(2*i)-1; k++) {
if( k == 1 || k == (2*i)-1 || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-26 (Down Empty Triangle)

StarPattern26.java
package com.javacodepoint.patterns;
public class StarPattern26 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=(2*i)-1; k++) {
if( k == 1 || k == (2*i)-1 || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-27 (Empty Diamond Pattern)
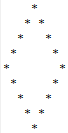
StarPattern27.java
package com.javacodepoint.patterns;
public class StarPattern27 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=(2*i)-1; k++) {
if( k == 1 || k == (2*i)-1 ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
// Lower half (skip the first line of printing)
// Outer loop for the line/row change
for(int i=n-1; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=(2*i)-1; k++) {
if( k == 1 || k == (2*i)-1 || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-28 (Sandglass Pattern)
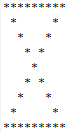
StarPattern28.java
package com.javacodepoint.patterns;
public class StarPattern28 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=(2*i)-1; k++) {
if( k == 1 || k == (2*i)-1 || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
// Lower half (skip the first line of printing)
// Outer loop for the line/row change
for(int i=2; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=(2*i)-1; k++) {
if( k == 1 || k == (2*i)-1 || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-29 (Square Pattern)

StarPattern29.java
package com.javacodepoint.patterns;
public class StarPattern29 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop for the star printing
for(int j=1; j<=n; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-30 (Empty Square Pattern)

StarPattern30.java
package com.javacodepoint.patterns;
public class StarPattern30 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop for the star and space printing
for(int j=1; j<=n; j++) {
if( i == 1 || j == 1 || i==n || j==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Conclusion
In this article, you have learned pattern programs in Java. Here you have seen the top 30 star pattern programs written in Java.
You can learn the Tricks to develop the logic for almost all types of pattern printing programs.
Learn also: Top 10 Number pattern programs in Java.