We have categorized all the patterns into three categories:
- Star Patterns
- Number Patterns
- Character Patterns or Alphabet Patterns
Star Patterns
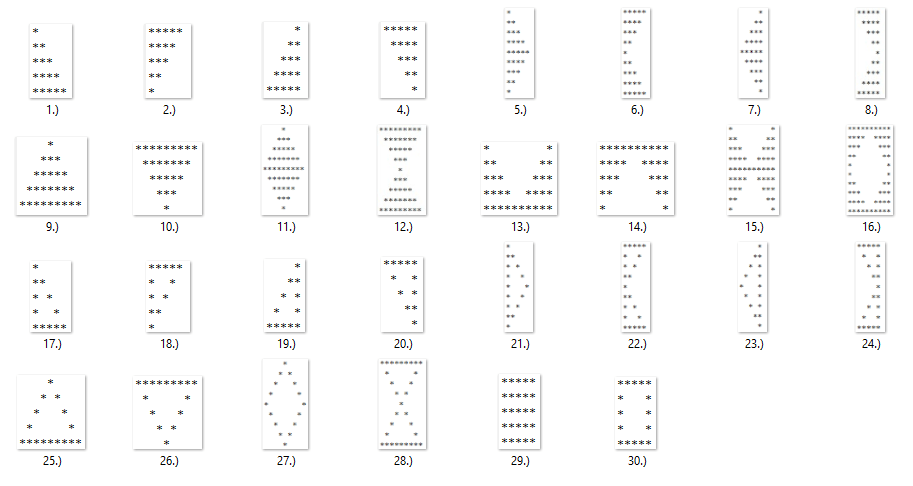
Number Patterns
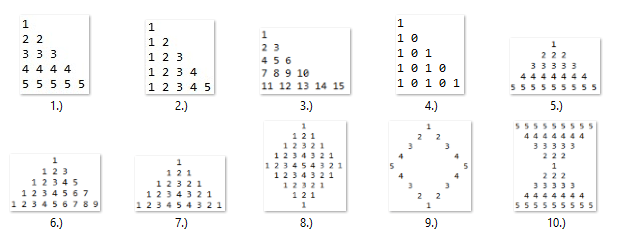
Character or Alphabet Patterns
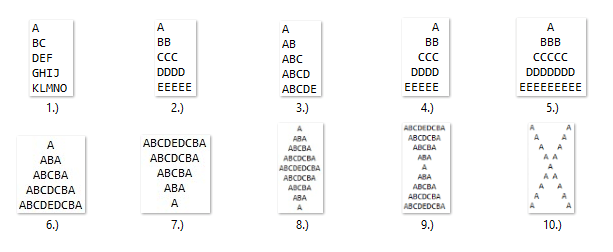
1.) Star Patterns
Pattern-1 (Right Triangle)

StarPattern1.java
package com.javacodepoint.patterns;
public class StarPattern1 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-2 (Down Right Triangle)

StarPattern2.java
package com.javacodepoint.patterns;
public class StarPattern2 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-3 (Mirrored Right Triangle)

StarPattern3.java
package com.javacodepoint.patterns;
public class StarPattern3 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star printing
for(int k=1; k<=i; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-4 (Down Mirrored Right Triangle)

StarPattern4.java
package com.javacodepoint.patterns;
public class StarPattern4 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop for the star printing
for(int k=1; k<=i; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-5 (Right Pascal’s Triangle)
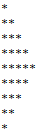
StarPattern5.java
package com.javacodepoint.patterns;
public class StarPattern5 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
// Lower half
// Outer loop for the line/row change
for(int i=(n-1); i>=1; i--) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-6 (K Shape Pattern)
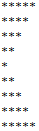
StarPattern6.java
package com.javacodepoint.patterns;
public class StarPattern6 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
// Lower half
// Outer loop for the line/row change
for(int i=2; i<=n; i++) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-7 (Mirrored Right Pascal’s Triangle)
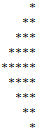
StarPattern7.java
package com.javacodepoint.patterns;
public class StarPattern7 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star printing
for(int k=1; k<=i; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
// Lower half
// Outer loop for the line/row change
for(int i=(n-1); i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star printing
for(int k=1; k<=i; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-8 (Reverse K Shape Pattern)
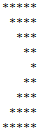
StarPattern8.java
package com.javacodepoint.patterns;
public class StarPattern8 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// First half
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star printing
for(int k=1; k<=i; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
// Second half
// Outer loop for the line/row change
for(int i=2; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star printing
for(int k=1; k<=i; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-9 (Pyramid Pattern)

StarPattern9.java
package com.javacodepoint.patterns;
public class StarPattern9 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-10 (Reversed Pyramid Pattern)

StarPattern10.java
package com.javacodepoint.patterns;
public class StarPattern10 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop for the star printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-11 (Diamond Star Pattern)
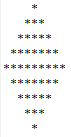
StarPattern11.java
package com.javacodepoint.patterns;
public class StarPattern11 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
// Lower half
// Outer loop for the line/row change
for(int i=n-1; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop for the star printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-12 (Sandglass Star Pattern)
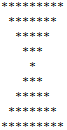
StarPattern12.java
package com.javacodepoint.patterns;
public class StarPattern12 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop for the star printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
// Lower half
// Outer loop for the line/row change
for(int i=2; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-13

StarPattern13.java
package com.javacodepoint.patterns;
public class StarPattern13 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Inner loop2 for the space printing
for(int k=1; k<=2*(n-i); k++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop3 for the again star printing
for(int l=1; l<=i; l++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-14

StarPattern14.java
package com.javacodepoint.patterns;
public class StarPattern14 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Inner loop2 for the space printing
for(int k=1; k<=2*(n-i); k++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop3 for the again star printing
for(int l=1; l<=i; l++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-15 (H Shape Pattern)
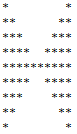
StarPattern15.java
package com.javacodepoint.patterns;
public class StarPattern15 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Inner loop2 for the space printing
for(int k=1; k<=2*(n-i); k++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop3 for the again star printing
for(int l=1; l<=i; l++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
// Lower half (skip the first line printing)
// Outer loop for the line/row change
for(int i=n-1; i>=1; i--) {
// Inner loop1 for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Inner loop2 for the space printing
for(int k=1; k<=2*(n-i); k++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop3 for the again star printing
for(int l=1; l<=i; l++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-16
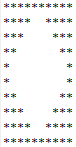
StarPattern16.java
package com.javacodepoint.patterns;
public class StarPattern16 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Inner loop2 for the space printing
for(int k=1; k<=2*(n-i); k++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop3 for the again star printing
for(int l=1; l<=i; l++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
// Lower half
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the star printing
for(int j=1; j<=i; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Inner loop2 for the space printing
for(int k=1; k<=2*(n-i); k++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop3 for the again star printing
for(int l=1; l<=i; l++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-17 (Empty Right Triangle)

StarPattern17.java
package com.javacodepoint.patterns;
public class StarPattern17 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop for the star and space printing
for(int j=1; j<=i; j++) {
if( j == 1 || j == i || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-18 (Down Empty Right Triangle)

StarPattern18.java
package com.javacodepoint.patterns;
public class StarPattern18 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop for the star and space printing
for(int j=1; j<=i; j++) {
if( j == 1 || j == i || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-19 (Mirrored Empty Right Triangle)

StarPattern19.java
package com.javacodepoint.patterns;
public class StarPattern19 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=i; k++) {
if( k == 1 || k == i || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-20 (Down Mirrored Empty Right Triangle)

StarPattern20.java
package com.javacodepoint.patterns;
public class StarPattern20 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=i; k++) {
if( k == 1 || k == i || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-21 (Empty Pascal’s Triangle)
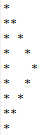
StarPattern21.java
package com.javacodepoint.patterns;
public class StarPattern21 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
if( j == 1 || j == i ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
// Lower half (skip the first line to print)
// Outer loop for the line/row change
for(int i=n-1; i>=1; i--) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
if( j == 1 || j == i || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-22
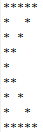
StarPattern22.java
package com.javacodepoint.patterns;
public class StarPattern22 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
if( j == 1 || j == i || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
// Lower half (skip the first line to print)
// Outer loop for the line/row change
for(int i=2; i<=n; i++) {
// Inner loop for the star printing
for(int j=1; j<=i; j++) {
if( j == 1 || j == i || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-23 (Mirrored Empty Pascal’s Triangle)
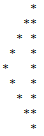
StarPattern23.java
package com.javacodepoint.patterns;
public class StarPattern23 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=i; k++) {
if( k == 1 || k == i ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
// Lower half
// Outer loop for the line/row change
for(int i=(n-1); i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=i; k++) {
if( k == 1 || k == i || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-24
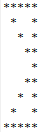
StarPattern24.java
package com.javacodepoint.patterns;
public class StarPattern24 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=i; k++) {
if( k == 1 || k == i || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
// Lower half
// Outer loop for the line/row change
for(int i=2; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=i; k++) {
if( k == 1 || k == i || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-25 (Empty Triangle)

StarPattern25.java
package com.javacodepoint.patterns;
public class StarPattern25 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=(2*i)-1; k++) {
if( k == 1 || k == (2*i)-1 || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-26 (Down Empty Triangle)

StarPattern26.java
package com.javacodepoint.patterns;
public class StarPattern26 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=(2*i)-1; k++) {
if( k == 1 || k == (2*i)-1 || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-27 (Empty Diamond Pattern)
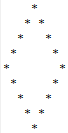
StarPattern27.java
package com.javacodepoint.patterns;
public class StarPattern27 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=(2*i)-1; k++) {
if( k == 1 || k == (2*i)-1 ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
// Lower half (skip the first line of printing)
// Outer loop for the line/row change
for(int i=n-1; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=(2*i)-1; k++) {
if( k == 1 || k == (2*i)-1 || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-28 (Sandglass Pattern)
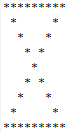
StarPattern28.java
package com.javacodepoint.patterns;
public class StarPattern28 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Upper half
// Outer loop for the line/row change
for(int i=n; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=(2*i)-1; k++) {
if( k == 1 || k == (2*i)-1 || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
// Lower half (skip the first line of printing)
// Outer loop for the line/row change
for(int i=2; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the star and space printing
for(int k=1; k<=(2*i)-1; k++) {
if( k == 1 || k == (2*i)-1 || i==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
Pattern-29 (Square Pattern)

StarPattern29.java
package com.javacodepoint.patterns;
public class StarPattern29 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop for the star printing
for(int j=1; j<=n; j++) {
// Printing the star without changing the line
System.out.print("*");
}
// Line/Row change
System.out.println();
}
}
}
Pattern-30 (Empty Square Pattern)

StarPattern30.java
package com.javacodepoint.patterns;
public class StarPattern30 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines/rows
int n = 5;
// Outer loop for the line/row change
for(int i=1; i<=n; i++) {
// Inner loop for the star and space printing
for(int j=1; j<=n; j++) {
if( i == 1 || j == 1 || i==n || j==n ) {
// Printing the star without changing the line
System.out.print("*");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line/Row change
System.out.println();
}
}
}
2.) Number Patterns
Pattern-1

Let’s see the java code to understand this pattern.
NumberPattern1.java
package com.javacodepoint.patterns;
public class NumberPattern1 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Outer loop for the line change
for(int i=1; i<=n; i++) {
// Inner loop for the number printing
for(int j=1; j<=i; j++) {
// Printing the number without changing the line
System.out.print(i+" ");
}
// Line change
System.out.println();
}
}
}
Pattern-2

Let’s see the java code to understand this pattern.
NumberPattern2.java
package com.javacodepoint.patterns;
public class NumberPattern2 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Outer loop for the line change
for(int i=1; i<=n; i++) {
// Inner loop for the number printing
for(int j=1; j<=i; j++) {
// Printing the number without changing the line
System.out.print(j+" ");
}
// Line change
System.out.println();
}
}
}
Pattern-3
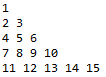
Let’s see the java code to understand this pattern.
NumberPattern3.java
package com.javacodepoint.patterns;
public class NumberPattern3 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Number to be printed
int num=1;
// Outer loop for the line change
for(int i=1; i<=n; i++) {
// Inner loop for the number printing
for(int j=1; j<=i; j++) {
// Printing the number without changing the line
System.out.print((num++)+" ");
}
// Line change
System.out.println();
}
}
}
Pattern-4

Let’s see the java code to understand this pattern.
NumberPattern4.java
package com.javacodepoint.patterns;
public class NumberPattern4 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Outer loop for the line change
for(int i=1; i<=n; i++) {
// Inner loop for the number printing
for(int j=1; j<=i; j++) {
// Printing the number without changing the line
if(j%2 == 0)
System.out.print("0 ");
else
System.out.print("1 ");
}
// Line change
System.out.println();
}
}
}
Pattern-5
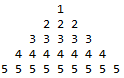
Let’s see the java code to understand this pattern.
NumberPattern5.java
package com.javacodepoint.patterns;
public class NumberPattern5 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Outer loop for the line change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the number printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the number without changing the line
System.out.print(i+" ");
}
// Line change
System.out.println();
}
}
}
Pattern-6
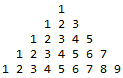
Let’s see the java code to understand this pattern.
NumberPattern6.java
package com.javacodepoint.patterns;
public class NumberPattern6 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Outer loop for the line change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the number printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the number without changing the line
System.out.print(k+" ");
}
// Line change
System.out.println();
}
}
}
Pattern-7
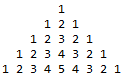
Let’s see the java code to understand this pattern.
NumberPattern7.java
package com.javacodepoint.patterns;
public class NumberPattern7 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Outer loop for the line change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the 2 space without changing the line
System.out.print(" ");
}
int num=0;
// Inner loop2 for the number printing
for(int k=1; k<=(2*i)-1; k++) {
if(k <= i)
// Printing first half by increasing the number
System.out.print((++num)+" ");
else
// Printing second half by decreasing the number
System.out.print((--num)+" ");
}
// Line change
System.out.println();
}
}
}
Pattern-8
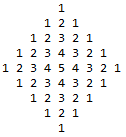
Let’s see the java code to understand this pattern.
NumberPattern8.java
package com.javacodepoint.patterns;
public class NumberPattern8 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Upper half
// Outer loop for the line change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the 2 spaces without changing the line
System.out.print(" ");
}
int num=0;
// Inner loop2 for the number printing
for(int k=1; k<=(2*i)-1; k++) {
if(k <= (2*i)/2 )
// Printing first half by increasing the number
System.out.print((++num)+" ");
else
// Printing second half by decreasing the number
System.out.print((--num)+" ");
}
// Line change
System.out.println();
}
// Lower half
// Outer loop for the line change
for(int i=n-1; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the 2 spaces without changing the line
System.out.print(" ");
}
int num=0;
// Inner loop2 for the number printing
for(int k=1; k<=(2*i)-1; k++) {
if(k <= (2*i)/2 )
// Printing first half by increasing the number
System.out.print((++num)+" ");
else
// Printing second half by decreasing the number
System.out.print((--num)+" ");
}
// Line change
System.out.println();
}
}
}
Pattern-9
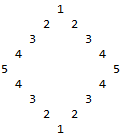
Let’s see the java code to understand this pattern.
NumberPattern9.java
package com.javacodepoint.patterns;
public class NumberPattern9 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Upper half
// Outer loop for the line change
for(int i=1; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the 2 spaces without changing the line
System.out.print(" ");
}
// Inner loop2 for the number printing
for(int k=1; k<=(2*i)-1; k++) {
if( k==1 || k== (2*i)-1 )
// Printing first & last number only
System.out.print(i+" ");
else
// Printing middle with spaces (Each times 2 spaces)
System.out.print(" ");
}
// Line change
System.out.println();
}
// Lower half
// Outer loop for the line change
for(int i=n-1; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the 2 spaces without changing the line
System.out.print(" ");
}
// Inner loop2 for the number printing
for(int k=1; k<=(2*i)-1; k++) {
if( k==1 || k== (2*i)-1 )
// Printing first & last number only
System.out.print(i+" ");
else
// Printing middle with spaces (Each times 2 spaces)
System.out.print(" ");
}
// Line change
System.out.println();
}
}
}
Pattern-10
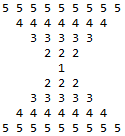
Let’s see the java code to understand this pattern.
NumberPattern10.java
package com.javacodepoint.patterns;
public class NumberPattern10 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Upper half
// Outer loop for the line change
for(int i=n; i>=1; i--) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the 2 spaces without changing the line
System.out.print(" ");
}
// Inner loop2 for the number printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the number without changing the line
System.out.print(i+" ");
}
// Line change
System.out.println();
}
// Lower half
// Outer loop for the line change
for(int i=2; i<=n; i++) {
// Inner loop1 for the space printing
for(int j=1; j<=(n-i); j++) {
// Printing the 2 spaces without changing the line
System.out.print(" ");
}
// Inner loop2 for the number printing
for(int k=1; k<=(2*i)-1; k++) {
// Printing the number without changing the line
System.out.print(i+" ");
}
// Line change
System.out.println();
}
}
}
3.) Character or Alphabet Patterns
Pattern-1

Let’s see the java code below to understand this pattern:
package com.javacodepoint.patterns;
public class CharacterPattern1 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Initializing the character with alphabet A (ASCII value)
int character = 65;
// Outer loop for the line change
for (int i = 1; i <= n; i++) {
// Inner loop for the character printing
for (int j = 1; j <= i; j++) {
// Printing the character without changing the line
System.out.print((char)character++);
}
// Line change
System.out.println();
}
}
}
Pattern-2

Let’s see the java code below to understand this pattern:
package com.javacodepoint.patterns;
public class CharacterPattern2 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Initializing the character with alphabet A (ASCII value)
int character = 65;
// Outer loop for the line change
for (int i = 1; i <= n; i++) {
// Inner loop for the character printing
for (int j = 1; j <= i; j++) {
// Printing the character without changing the line
System.out.print((char)character);
}
character++;
// Line change
System.out.println();
}
}
}
Pattern-3

Let’s see the java code below to understand this pattern:
package com.javacodepoint.patterns;
public class CharacterPattern3 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Outer loop for the line change
for (int i = 1; i <= n; i++) {
// Initializing the character with alphabet A (ASCII value)
int character = 65;
// Inner loop for the character printing
for (int j = 1; j <= i; j++) {
// Printing the character without changing the line
System.out.print((char)character++);
}
// Line change
System.out.println();
}
}
}
Pattern-4

Let’s see the java code below to understand this pattern:
package com.javacodepoint.patterns;
public class CharacterPattern4 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Initializing the character with alphabet A (ASCII value)
int character = 65;
// Outer loop for the line change
for (int i = 1; i <= n; i++) {
// Inner loop1 for the space printing
for (int j = 1; j <= n-i; j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the character printing
for (int k = 1; k <= i; k++) {
// Printing the character without changing the line
System.out.print((char)character);
}
character++;
// Line change
System.out.println();
}
}
}
Pattern-5

Let’s see the java code below to understand this pattern:
package com.javacodepoint.patterns;
public class CharacterPattern5 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Initializing the character with alphabet A (ASCII value)
int character = 65;
// Outer loop for the line change
for (int i = 1; i <= n; i++) {
// Inner loop1 for the space printing
for (int j = 1; j <= n-i; j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the character printing
for (int k = 1; k <= (2*i)-1; k++) {
// Printing the character without changing the line
System.out.print((char)character);
}
character++;
// Line change
System.out.println();
}
}
}
Pattern-6

Let’s see the java code below to understand this pattern:
package com.javacodepoint.patterns;
public class CharacterPattern6 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Outer loop for the line change
for (int i = 1; i <= n; i++) {
// Inner loop1 for the space printing
for (int j = 1; j <= n-i; j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Initializing the character before alphabet A (ASCII value)
int character = 64;
// Inner loop2 for the character printing
for (int k = 1; k <= (2*i)-1; k++) {
if(k <= i) {
// Printing the character without changing the line
System.out.print((char)++character);
}else {
// Printing the character without changing the line
System.out.print((char)--character);
}
}
// Line change
System.out.println();
}
}
}
Pattern-7

Let’s see the java code below to understand this pattern:
package com.javacodepoint.patterns;
public class CharacterPattern7 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Outer loop for the line change
for (int i = n; i >= 1; i--) {
// Inner loop1 for the space printing
for (int j = 1; j <= n-i; j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Initializing the character before alphabet A (ASCII value)
int character = 64;
// Inner loop2 for the character printing
for (int k = 1; k <= (2*i)-1; k++) {
if(k <= i) {
// Printing the character without changing the line
System.out.print((char)++character);
}else {
// Printing the character without changing the line
System.out.print((char)--character);
}
}
// Line change
System.out.println();
}
}
}
Pattern-8
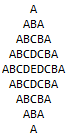
Let’s see the java code below to understand this pattern:
package com.javacodepoint.patterns;
public class CharacterPattern8 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Upper half
// Outer loop for the line change
for (int i = 1; i <= n; i++) {
// Inner loop1 for the space printing
for (int j = 1; j <= n-i; j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Initializing the character before alphabet A (ASCII value)
int character = 64;
// Inner loop2 for the character printing
for (int k = 1; k <= (2*i)-1; k++) {
if(k <= i) {
// Printing the character without changing the line
System.out.print((char)++character);
}else {
// Printing the character without changing the line
System.out.print((char)--character);
}
}
// Line change
System.out.println();
}
// Lower half
// Outer loop for the line change
for (int i = n-1; i >= 1; i--) {
// Inner loop1 for the space printing
for (int j = 1; j <= n-i; j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Initializing the character before alphabet A (ASCII value)
int character = 64;
// Inner loop2 for the character printing
for (int k = 1; k <= (2*i)-1; k++) {
if(k <= i) {
// Printing the character without changing the line
System.out.print((char)++character);
}else {
// Printing the character without changing the line
System.out.print((char)--character);
}
}
// Line change
System.out.println();
}
}
}
Pattern-9
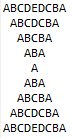
Let’s see the java code below to understand this pattern:
package com.javacodepoint.patterns;
public class CharacterPattern9 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Upper half
// Outer loop for the line change
for (int i = n; i >= 1; i--) {
// Inner loop1 for the space printing
for (int j = 1; j <= n-i; j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Initializing the character before alphabet A (ASCII value)
int character = 64;
// Inner loop2 for the character printing
for (int k = 1; k <= (2*i)-1; k++) {
if(k <= i) {
// Printing the character without changing the line
System.out.print((char)++character);
}else {
// Printing the character without changing the line
System.out.print((char)--character);
}
}
// Line change
System.out.println();
}
// Lower half
// Outer loop for the line change
for (int i = 2; i <= n; i++) {
// Inner loop1 for the space printing
for (int j = 1; j <= n-i; j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Initializing the character before alphabet A (ASCII value)
int character = 64;
// Inner loop2 for the character printing
for (int k = 1; k <= (2*i)-1; k++) {
if(k <= i) {
// Printing the character without changing the line
System.out.print((char)++character);
}else {
// Printing the character without changing the line
System.out.print((char)--character);
}
}
// Line change
System.out.println();
}
}
}
Pattern-10
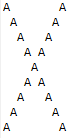
Let’s see the java code below to understand this pattern:
package com.javacodepoint.patterns;
public class CharacterPattern10 {
// Main Method
public static void main(String[] args) {
// Initializing required number of lines
int n = 5;
// Upper half
// Outer loop for the line change
for (int i = n; i >= 1; i--) {
// Inner loop1 for the space printing
for (int j = 1; j <= n-i; j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the character printing
for (int k = 1; k <= (2*i)-1; k++) {
if(k == 1 || k == (2*i)-1) {
// Printing the character without changing the line
System.out.print("A");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line change
System.out.println();
}
// Lower half
// Outer loop for the line change
for (int i = 2; i <= n; i++) {
// Inner loop1 for the space printing
for (int j = 1; j <= n-i; j++) {
// Printing the space without changing the line
System.out.print(" ");
}
// Inner loop2 for the character printing
for (int k = 1; k <= (2*i)-1; k++) {
if(k == 1 || k == (2*i)-1) {
// Printing the character without changing the line
System.out.print("A");
}else {
// Printing the space without changing the line
System.out.print(" ");
}
}
// Line change
System.out.println();
}
}
}
Conclusion
Here you have seen 30 different Star patterns, 10 Alphabet patterns, and 10 Number patterns program logic in java.
If you are interested to learn the Tricks for developing the logic for pattern printing programs, visit the link below to which will help you in writing logic for almost all types of pattern programs in java.
Pattern programs [Tricks] in java.