In this article, you will learn to Create cells in Excel Files in Java using Apache POI. When it comes to dealing with Excel files programmatically, Apache POI stands out as a powerful library, enabling developers to create, modify, and manage Excel spreadsheets with ease.
Table of Contents
Introduction to Apache POI
Apache POI, an open-source Java library, provides APIs for working with various Microsoft document formats, including Excel spreadsheets (.xls and .xlsx). It empowers developers to read, write, and modify Excel files programmatically, offering flexibility and control over spreadsheet manipulation.
Setting up Apache POI in Your Project
Before we begin, ensure that you have Apache POI added to your Java project’s dependencies. You can download the latest version of Apache POI from the Apache POI website or include it as a dependency in your project’s build. If you’re using Maven, include the following dependencies in your pom.xml
file:
<!-- https://mvnrepository.com/artifact/org.apache.poi/poi -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>5.1.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.poi/poi-ooxml -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>5.1.0</version>
</dependency>
Learn the complete Apache POI setup in Eclipse IDE: Install Apache POI in Eclipse.
For Gradle projects, add the following dependencies to your build.gradle
file:
implementation 'org.apache.poi:poi:5.1.0'
implementation 'org.apache.poi:poi-ooxml:5.1.0'
NOTE: These lines specify the dependencies for the poi
 and poi-ooxml
 artifacts with version 5.1.0
. Make sure to sync your Gradle project after adding these dependencies to download and include the Apache POI library in your project.
Create Cells in Excel Files using Apache POI
Let’s get into the heart of Excel creation: creating cells within Excel files using Apache POI. Below is a simple example demonstrating how to create a new Excel file and populate cells with data:
Follow these simple steps to create cells in Excel using Apache POI:
- Create a new Excel workbook using
XSSFWorkbook
. - Create a new sheet named “Sheet1”.
- Create a row at index 0 within the sheet.
- Create cells at specific indices within the row and set their values.
- Write the workbook content to a file named “output.xlsx”.
package com.javacodepoint.example;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class CreateCellsExcelExample {
public static void main(String[] args) {
// Create a new Excel workbook
try (Workbook workbook = new XSSFWorkbook()) {
Sheet sheet = workbook.createSheet("Sheet1");
// Create a row at index 0
Row row = sheet.createRow(0);
// Create cells and set values
Cell cell1 = row.createCell(0);
cell1.setCellValue("Hello");
Cell cell2 = row.createCell(1);
cell2.setCellValue("World");
// Write the workbook content to a file
try (FileOutputStream fileOut = new FileOutputStream("output.xlsx")) {
workbook.write(fileOut);
}
System.out.println("Cells created in Excel successfully!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
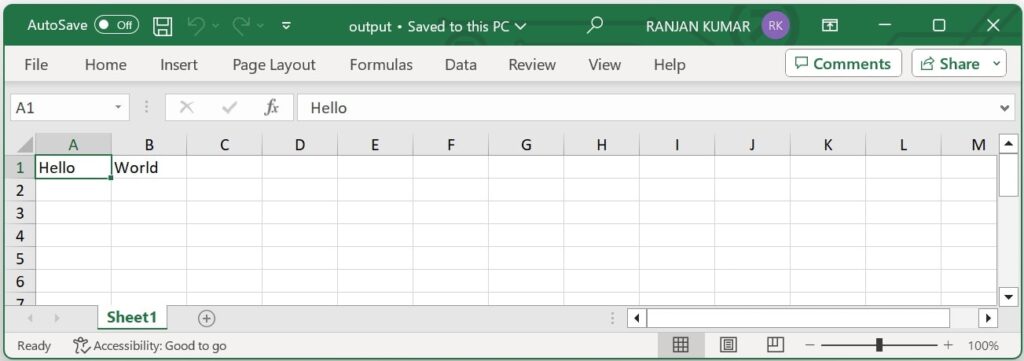
Details of Used Classes and Methods
In the example provided for creating cells in an Excel file using Apache POI in Java, several classes and methods from the Apache POI library are utilized. Let’s dive into the details of the classes and methods used:
- Workbook (org.apache.poi.ss.usermodel.Workbook):
- This interface represents a high-level abstraction of an Excel workbook.
- In the example, the
XSSFWorkbook
class is used to create a new Excel workbook in the.xlsx
format.
- Sheet (org.apache.poi.ss.usermodel.Sheet):
- Represents an Excel worksheet within a workbook.
- Used to create and manage sheets within the workbook.
- In the example, the
createSheet()
method of theWorkbook
interface is called to create a new sheet named “Sheet1”.
- Row (org.apache.poi.ss.usermodel.Row):
- Represents a row within an Excel worksheet.
- Used to create and manage rows within a sheet.
- In the example, the
createRow()
method of theSheet
interface is called to create a new row at index 0 within the sheet.
- Cell (org.apache.poi.ss.usermodel.Cell):
- Represents a cell within an Excel worksheet.
- Used to create and manage cells within a row.
- In the example, the
createCell()
method of theRow
interface is called to create cells at specific indices within the row.
- FileOutputStream (java.io.FileOutputStream):
- Represents an output stream for writing data to a file.
- Used to write the workbook content to an Excel file.
- In the example, an instance of
FileOutputStream
is used to write the workbook content to a file named “output.xlsx”.
- XSSFWorkbook (org.apache.poi.xssf.usermodel.XSSFWorkbook):
- Represents an Excel workbook in the
.xlsx
format. - This class is used to create a new Excel workbook.
- Represents an Excel workbook in the
- setCellValue():
- Method of the
Cell
interface. - Used to set the value of a cell.
- In the example, the
setCellValue()
method is called to set the values of the cells created in the worksheet.
- Method of the
- write():
- Method of the
Workbook
interface. - Used to write the workbook content to an output stream.
- In the example, the
write()
method is called to write the workbook content to theFileOutputStream
.
- Method of the
Conclusion
In this article, we explored the basics of creating cells in Excel files in Java using Apache POI, a powerful Java library for working with Microsoft Office documents. By following the steps outlined in this guide, you can generate Excel files programmatically, customize their content, and automate spreadsheet-related tasks with ease. Apache POI’s rich feature set and flexibility make it an indispensable tool for Java developers working with Excel files in their applications.
Related Articles:
- How to Create a New Workbook POI Example
- How to Create a Sheet Apache POI Example
- Apache POI – Read and Write Excel files in Java
- How to create password-protected Excel in Java?
- How to write data to an existing Excel file in Java?