This article shows you how to convert Binary to Decimal using recursion in Java. Recursion in java is a technique in which a method calls itself continuously. A method in java that calls itself is called a recursive method.
How to convert binary to decimal?
Binary to Decimal conversion is straightforward, look into the below diagram for conversion in steps:
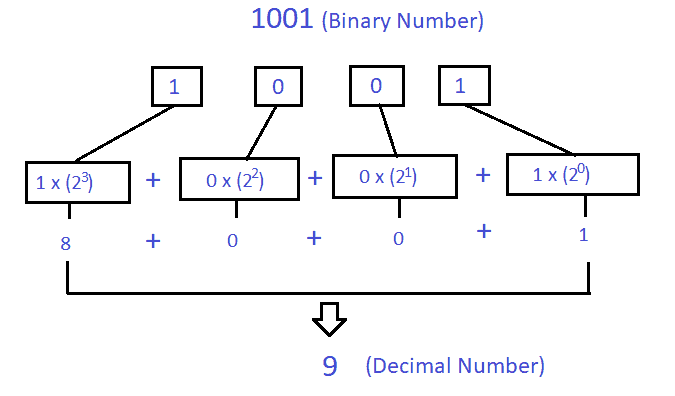
Java Program to Convert Binary to Decimal
Here, we will see two java programs for binary-to-decimal conversion using recursion in Java.
- Binary to Decimal – if the binary number is in integer format
- Binary to Decimal – if the binary number is in string format
Binary to Decimal using Recursion – if the binary number is in integer format
BinaryToDecimalRecursion.java
package com.javacodepoint.conversion;
/**
* Binary to Decimal conversion using Recursion.
*/
public class BinaryToDecimalRecursion {
// recursive method for binary to decimal
public static int binaryToDecimal(int binary) {
// if binary number become 0
if (binary == 0) {
return 0;
}
return (binary % 10 + 2 * binaryToDecimal(binary / 10));
}
// main method to test
public static void main(String[] args) {
// Binary number is in integer format
System.out.println("Decimal Number of 1001 is: " + binaryToDecimal(1001));
System.out.println("Decimal Number of 1111 is: " + binaryToDecimal(1111));
System.out.println("Decimal Number of 1100110 is: " + binaryToDecimal(1100110));
System.out.println("Decimal Number of 1000000001 is: " + binaryToDecimal(1000000001));
}
}
OUTPUT:
Decimal Number of 1001 is: 9
Decimal Number of 1111 is: 15
Decimal Number of 1100110 is: 102
Decimal Number of 1000000001 is: 513
Binary to Decimal using Recursion – if the binary number is in string format
BinaryToDecimalRecursion2.java
package com.javacodepoint.conversion;
/**
* Binary to Decimal conversion using Recursion.
*/
public class BinaryToDecimalRecursion2 {
// recursive method for binary to decimal
public static int binaryToDecimal(String binary, int i) {
int length = binary.length();
// if we reached to last character
if (i == length - 1) {
return binary.charAt(i) - '0';
}
// add current term and recursion for
// remaining terms
return ((binary.charAt(i) - '0') << (length - i - 1)) + binaryToDecimal(binary, i + 1);
}
// main method
public static void main(String[] args) {
// Binary number is in string format
System.out.println("Decimal Number of 101 is: " + binaryToDecimal("101", 0));
System.out.println("Decimal Number of 1010 is: " + binaryToDecimal("1010", 0));
System.out.println("Decimal Number of 1111000 is: " + binaryToDecimal("1111000", 0));
System.out.println("Decimal Number of 1000000001 is: " + binaryToDecimal("1000000001", 0));
}
}
OUTPUT:
Decimal Number of 101 is: 5
Decimal Number of 1010 is: 10
Decimal Number of 1111000 is: 120
Decimal Number of 1000000001 is: 513
There are multiple ways for Binary to Decimal conversion also explained in another article, visit Java Binary to decimal conversion Program Examples.