Uploading files is a common feature in modern web applications. Traditionally, file inputs have been implemented using the standard HTML <input type="file">
element. In this article, you will see how to create a standard drag & drop file uploader user interface(UI) in javascript. Drag and drop upload makes it much easier for the user to upload the files.
Here to create a drag-and-drop uploader, we are going to use basic HTML, CSS, and Javascript.
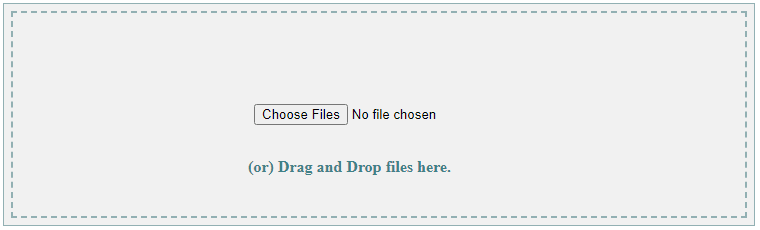
Table of Contents
Drag and drop meaning
Drag and drop is a method of moving computer files, images, videos, etc. from one place to another by clicking on them with the mouse and moving them across the screen. We can also say, Drag and drop is a functionality by which users can select an image or file and can move it to the desired location, and “drop” it there.
How does drag and drop work?
The following set of sequences is involved in the drag-and-drop process:
- Move the pointer to the object (images, files, etc.)
- Press, and hold down, the button on the mouse or other pointing device, to “grab” the object
- “Drag” the object to the desired location by moving the pointer to this one
- “Drop” the object by releasing the button
Drag and drop file upload HTML CSS
Here, we will create a drag-and-drop file upload user interface using basic HTML and CSS only.
Basic HTML
First of all, we define the basic HTML for file upload:
<div class="upload-container">
<input type="file" id="file_upload" />
</div>
<br>
<button class="upload-btn" onclick="uploadFiles()">Submit</button>
Let’s understand the above HTML,
- Here we defined an upload container area to drop uploading files.
- The HTML
<input>
element is defined with an attributetype="file"
to hold the uploading file. - The HTML
<button>
element is defined to submit the uploading files.
Drag and drop multiple file upload
We can also upload multiple files at a time using drag and drop. We just need to modify the <input>
to accept multiple files. We have to add the attribute "multiple"
like the below code. The attribute multiple="true"
is defined to accept one or more than one file at a time.
<input type="file" id="file_upload" multiple="true" />
Basic CSS for the file upload
Let’s define the basic CSS for the above HTML structure to provide the look and feel of the drag-and-drop file uploader.
Define the below CSS code in the <style> tag of the HTML page:
.upload-container {
position: relative;
}
.upload-container input {
border: 1px solid #92b0b3;
background: #f1f1f1;
outline: 2px dashed #92b0b3;
outline-offset: -10px;
padding: 100px 0px 100px 250px;
text-align: center !important;
width: 500px;
}
.upload-container input:hover {
background: #ddd;
}
.upload-container:before {
position: absolute;
bottom: 50px;
left: 245px;
content: " (or) Drag and Drop files here. ";
color: #3f8188;
font-weight: 900;
}
.upload-btn {
margin-left: 300px;
padding: 7px 20px;
}
Drag and drop file upload javascript
Now we will use Javascript code to read the uploading files and submit them to upload.
Define the below javascript code in <script> tag of the HTML page:
function uploadFiles() {
var files = document.getElementById('file_upload').files;
if(files.length==0){
alert("Please first choose or drop any file(s)...");
return;
}
var filenames="";
for(var i=0;i<files.length;i++){
filenames+=files[i].name+"\n";
}
alert("Selected file(s) :\n____________________\n"+filenames);
}
The above-defined function uploadFiles()
will be called to submit the uploading file when the user clicks on submit button.
Drag and drop file upload example with submit button
Now, we can put all code together in a single HTML page like the below filesupload.html file:
filesupload.html
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1">
<style>
.upload-container {
position: relative;
}
.upload-container input {
border: 1px solid #92b0b3;
background: #f1f1f1;
outline: 2px dashed #92b0b3;
outline-offset: -10px;
padding: 100px 0px 100px 250px;
text-align: center !important;
width: 500px;
}
.upload-container input:hover {
background: #ddd;
}
.upload-container:before {
position: absolute;
bottom: 50px;
left: 245px;
content: " (or) Drag and Drop files here. ";
color: #3f8188;
font-weight: 900;
}
.upload-btn {
margin-left: 300px;
padding: 7px 20px;
}
</style>
<script>
function uploadFiles() {
var files = document.getElementById('file_upload').files;
if(files.length==0){
alert("Please first choose or drop any file(s)...");
return;
}
var filenames="";
for(var i=0;i<files.length;i++){
filenames+=files[i].name+"\n";
}
alert("Selected file(s) :\n____________________\n"+filenames);
}
</script>
</head>
<body>
<div class="upload-container">
<input type="file" id="file_upload" multiple />
</div>
<br>
<button class="upload-btn" onclick="uploadFiles()">Submit</button>
</body>
</html>
Preview and Live Demo
If you open the above HTML page in the browser it will look like the below image:
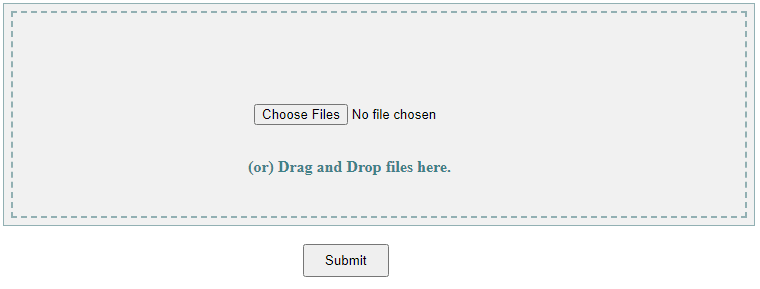
Conclusion
Implementing Drag & Drop and Browse file upload functionalities in JavaScript can significantly enhance the file uploading experience for users. While the Browse file upload is the standard method and works well in most cases, Drag & Drop adds a touch of interactivity and modernity.
You can use this UI to upload single as well as multiple files to the server by using any backend technologies such as Servlet, Spring boot, etc. Visit the link if you want to do file upload in Spring Boot, Spring Boot File Upload with Advance Progress bar in Ajax
Visit the link to do the file upload in Java Servlet, Ajax File Upload with Advance Progress Bar in Java
Related Articles:
- File upload validations in javascript
- Preview an image before uploading using Javascript
- Preview an image before uploading using jQuery
- File Upload in Java Servlet Example
- Multiple file uploads in Java with Progress bar – Ajax
- Step-by-step Java file upload | Ajax Progress bar
You might like this:
- How to create a Stopwatch in JavaScript?
- Apache POI – Read and Write Excel files in Java
- How to create password-protected Excel in Java?
- How to Read Password-protected Excel in Java?
- How to write data to an existing Excel file in Java?
- [Java code] Convert Excel file to CSV with Apache POI
if i drop the file in the text, ((or) Drag and Drop files here.), doesn’t work
I’m not sure where you’re getting your info, but great topic. I needs to spend some time learning much more or understanding more.
Thanks for wonderful information I was looking for this information for my mission.