In this article, you will see how to preview an image before uploading it to the Server. Previewing the image is the best user experience. The users could be able to see which image they are going to upload.
Here we are going to use HTML, CSS, and Javascript to develop it. Let’s see how it can be done?
You just need to follow the below simple steps:
- Define the basic HTML
- Define the basic CSS for the HTML to apply the style
- Define the javascript code to preview the image and reset
Table of Contents
Define the basic HTML
We need to define the basic HTML elements to choose an image file and preview/display it in some container.
<body>
<h1>Preview an image before uploading using Javascript</h1>
<p>Upload an image file (.JPG,.JPEG,.PNG,.GIF) </p>
<!-- input element to choose a file for uploading -->
<input type="file" accept="image/*" id="image-upload" onchange="previewImage(event)" />
<br>
<!-- img element to preview or display the uploading image -->
<img id="image-container" />
<br>
<button onclick="uploadImage()">Upload</button>
<button onclick="cancelUploading()">Cancel</button>
</body>
Let’s understand the above code with simple points:
- We are defining an HTML
<input>
element to choose an image file. - We are calling
previewImage(event)
function on the change event of<input>
. - We are defining an HTML
<img>
element to display/preview the image. - Now we are defining two HTML
<button>
elements to upload and cancel uploading. - We are calling
uploadImage()
function to upload image andcancelUploading()
function to reset uploading on specific button click.
Define the basic CSS
We are going to define some basic CSS for the above HTML element to apply the style. Let’s see the below CSS style which needs to be defined in <style> tag in HTML page:
/* to make everything center aligned */
body{
text-align:center;
}
/* to set specific height and width for image preview */
#image-container{
margin : 20px;
height: 200px;
width: 400px;
background-color:#eee;
border: 1px dashed #000;
}
Define the javascript code to preview the image and reset
Now we need to define javascript code to read the image file and to preview the image and reset if don’t need to upload it.
Here we are going to define three javascript function previewImage()
, uploadImage()
, cancelUploading()
to perform specific functionality.
function previewImage(event){
/* creating FileReader object to read the file */
var reader = new FileReader();
reader.onload = function(){
var imageContainer = document.getElementById('image-container');
imageContainer.src = reader.result;
};
/* Read the selected image as URL in base64 */
reader.readAsDataURL(event.target.files[0]);
}
function uploadImage(){
/* file validation logic goes here if required */
/* image uploading logic goes here */
alert("Upload logic need to be write here...");
}
function cancelUploading(){
/* Reset input element */
document.getElementById('image-upload').value = "";
/* Clear image preview container */
var imageContainer = document.getElementById('image-container');
imageContainer.src = "";
}
Let’s understand the above code with simple points:
- The
previewImage()
is defined to preview or display the image in an image container. - Inside
previewImage()
,FileReader
class object is created to read the uploading file. ThereadAsDataURL()
read the image as base64. - The
uploadImage()
is defined to upload image if file is valid. - The
cancelUploading()
is defined to reset the image container if we don’t need to upload it.
Complete Example
Let’s put all the above code together in a single HTML page as follow:
preview_image.html
<!DOCTYPE html>
<html>
<head>
<title>
Preview an image before uploading using Javascript
</title>
<style>
body{
text-align:center;
}
#image-container{
margin : 20px;
height: 200px;
width: 400px;
background-color:#eee;
border: 1px dashed #000;
}
</style>
</head>
<body>
<h1>Preview an image before uploading using Javascript</h1>
<p>Upload an image file (.JPG,.JPEG,.PNG,.GIF) </p>
<!-- input element to choose a file for uploading -->
<input type="file" accept="image/*" id="image-upload" onchange="previewImage(event)" />
<br>
<!-- img element to preview or display the image -->
<img id="image-container" />
<br>
<button onclick="uploadImage()">Upload</button>
<button onclick="cancelUploading()">Cancel</button>
<script>
function previewImage(event){
/* creating FileReader object to read the file */
var reader = new FileReader();
reader.onload = function(){
var imageContainer = document.getElementById('image-container');
imageContainer.src = reader.result;
};
/* Read the selected image as URL in base64 */
reader.readAsDataURL(event.target.files[0]);
}
function uploadImage(){
/* file validation logic goes here if required */
/* image uploading logic goes here */
alert("Upload logic need to be write here...");
}
function cancelUploading(){
/* Reset input element */
document.getElementById('image-upload').value = "";
/* Clear image preview container */
var imageContainer = document.getElementById('image-container');
imageContainer.src = "";
}
</script>
</body>
</html>
Preview and Live Demo
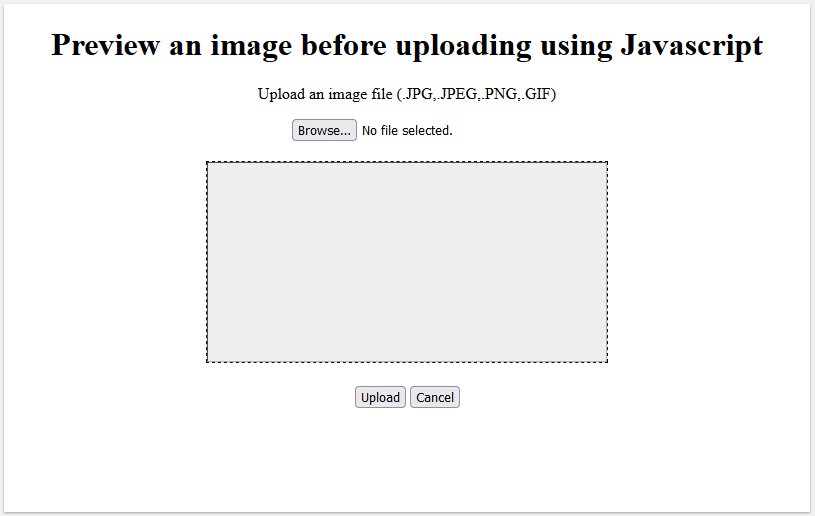
Conclusion
In this article, you have seen how to preview an image before uploading it to the server using Javascript.
You can learn how to validate files before uploading them to the server using Javascript.