In this article, you will see how to create a “Stopwatch” application. Creating a Stopwatch application is very simple in Javascript. There might be multiple solutions for it but here we will use Javascript setTimeout() function to develop this application.
The setTimeout() is a javascript method available in the window object. The setTimeout() method calls a function after a specified number of milliseconds.
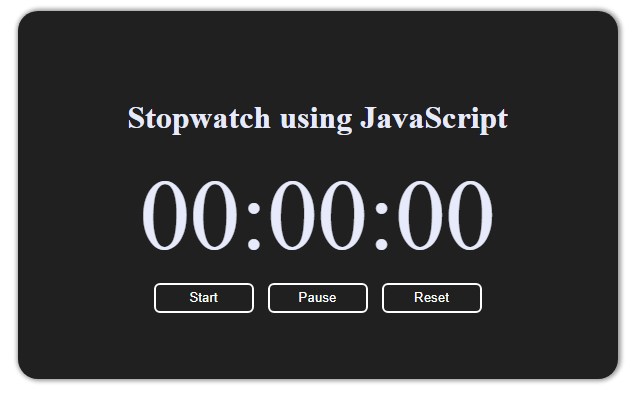
Let’s start creating a Stopwatch application.
Table of Contents
Creating Stopwatch using JavaScript
We are going to develop this application in the following steps-
- Define simple HTML
- Define CSS
- Define Javascript logic
1. Define Simple HTML
<!DOCTYPE html>
<html>
<head>
<title>JavaScript StopWatch</title>
</head>
<body>
<div id="stopwatch-container">
<h1>Stopwatch using JavaScript</h1>
<div id="stopwatch">00:00:00</div>
<button onclick="start(true)" id="start-btn">Start</button>
<button onclick="pause()">Pause</button>
<button onclick="reset()">Reset</button>
</div>
</body>
</html>
Explanation:
- Here we have defined one division(div) area with
id="stopwatch"
to display stopwatch time. - We have defined three buttons to do start, pause, and reset functionality of the stopwatch.
- Again we have defined another div container with
id="stopwatch-container"
to contain all the above-defined elements.
2. Define CSS
#stopwatch-container {
width: 600px;
margin: 10% auto;
padding: 5% 0;
color: #e7eafb;
text-align: center;
background-color: #202020;
box-shadow: 1px 0px 4px 2px #9c9a9a;
border-radius: 20px;
}
#stopwatch {
font-size: 100px;
margin-bottom: 10px;
}
button {
width: 100px;
height: 30px;
background-color: #202020;
color: white;
border-radius: 6px;
border: 2px solid #fff;
margin-left: 5px;
margin-right: 5px;
}
button:hover {
background-color: #fff;
color: #202020;
cursor: pointer;
}
3. Define Javascript logic
var stopwatch = document.getElementById("stopwatch");
var startBtn = document.getElementById("start-btn");
var timeoutId = null;
var ms = 0;
var sec = 0;
var min = 0;
/* function to start stopwatch */
function start(flag) {
if (flag) {
startBtn.disabled = true;
}
timeoutId = setTimeout(function() {
ms = parseInt(ms);
sec = parseInt(sec);
min = parseInt(min);
ms++;
if (ms == 100) {
sec = sec + 1;
ms = 0;
}
if (sec == 60) {
min = min + 1;
sec = 0;
}
if (ms < 10) {
ms = '0' + ms;
}
if (sec < 10) {
sec = '0' + sec;
}
if (min < 10) {
min = '0' + min;
}
stopwatch.innerHTML = min + ':' + sec + ':' + ms;
// calling start() function recursivly to continue stopwatch
start();
}, 10); // setTimeout delay time 10 milliseconds
}
/* function to pause stopwatch */
function pause() {
clearTimeout(timeoutId);
startBtn.disabled = false;
}
/* function to reset stopwatch */
function reset() {
ms = 0;
sec = 0;
min = 0;
clearTimeout(timeoutId);
stopwatch.innerHTML = '00:00:00';
startBtn.disabled = false;
}
Explanation:
- Here we defined some global variable such as
stopwatch
,startBtn
,timeoutId
, ms,sec
,min
. stopwatch
hold the refrence of stopwatch element and it is used for display the time.startBtn
hold the refrence of start button which is used for enable and disable it.timoutId
hold the id returnd bysetTimeout()
function to clear the timer.ms
,sec
, andmin
hold the value of milliseconds, seconds, and minutes respectivaly.- We have defined
start()
method to start the stopwatch. Inside this we usedsetTimeout()
method to calculate ms,sec,and min after specified number of milliseconds. - Inside
start()
method, making start button disable at first time to restrict user clicking multiple time to it. - We have defined
pause()
,reset()
method to pause and reset the stopwatch. Inside both the method making start button enable to allow user to start it again. - We are clearing the timer using
clearTimeout()
in both methodpause()
andreset()
to stop the stopwatch calculations.
Complete Stopwatch Application
Putting the HTML, CSS, and JavaScript code all together in a single .html document.
<!DOCTYPE html>
<html>
<head>
<title>JavaScript StopWatch</title>
<style>
#stopwatch-container {
width: 600px;
margin: 10% auto;
padding: 5% 0;
color: #e7eafb;
text-align: center;
background-color: #202020;
box-shadow: 1px 0px 4px 2px #9c9a9a;
border-radius: 20px;
}
#stopwatch {
font-size: 100px;
margin-bottom: 10px;
}
button {
width: 100px;
height: 30px;
background-color: #202020;
color: white;
border-radius: 6px;
border: 2px solid #fff;
margin-left: 5px;
margin-right: 5px;
}
button:hover {
background-color: #fff;
color: #202020;
cursor: pointer;
}
</style>
</head>
<body>
<div id="stopwatch-container">
<h1>Stopwatch using JavaScript</h1>
<div id="stopwatch">00:00:00</div>
<button onclick="start(true)" id="start-btn">Start</button>
<button onclick="pause()">Pause</button>
<button onclick="reset()">Reset</button>
</div>
<script>
var stopwatch = document.getElementById("stopwatch");
var startBtn = document.getElementById("start-btn");
var timeoutId = null;
var ms = 0;
var sec = 0;
var min = 0;
/* function to start stopwatch */
function start(flag) {
if (flag) {
startBtn.disabled = true;
}
timeoutId = setTimeout(function() {
ms = parseInt(ms);
sec = parseInt(sec);
min = parseInt(min);
ms++;
if (ms == 100) {
sec = sec + 1;
ms = 0;
}
if (sec == 60) {
min = min + 1;
sec = 0;
}
if (ms < 10) {
ms = '0' + ms;
}
if (sec < 10) {
sec = '0' + sec;
}
if (min < 10) {
min = '0' + min;
}
stopwatch.innerHTML = min + ':' + sec + ':' + ms;
// calling start() function recursivly to continue stopwatch
start();
}, 10); // setTimeout delay time 10 milliseconds
}
/* function to pause stopwatch */
function pause() {
clearTimeout(timeoutId);
startBtn.disabled = false;
}
/* function to reset stopwatch */
function reset() {
ms = 0;
sec = 0;
min = 0;
clearTimeout(timeoutId);
stopwatch.innerHTML = '00:00:00';
startBtn.disabled = false;
}
</script>
</body>
</html>
Preview and Live Demo
Let’s have a look at the output of the stopwatch application here:
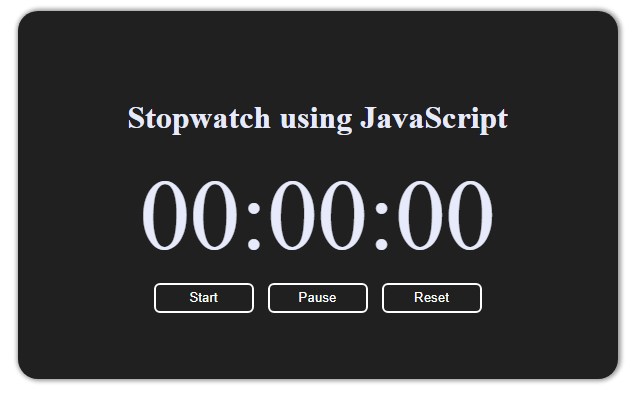
Conclusion
In this article, you have seen how to create a stopwatch application using the setTimeout() method in javascript.
Here you might observe the result of Stopwatch is not accurate. In another article, we used the Date.now() along with setTimeout() to make it more accurate.
Visit the article here: Accurate and Easy Stopwatch in JavaScript.
FAQ
What is setTimeout()?
The setTimeout() is a javascript method available in the window object. This method calls a function after a specified number of milliseconds.Â
How do I cancel setTimeout?
To cancel a setTimeout() method from running, you need to use the clearTimeout() method by passing the ID which is returned by the setTimeout() method.