In this article, you will learn how to Convert CSV to JSON using Javascript. A CSV file is a comma-separated value with a .csv extension, which allows users to store data in a tabular format. Each line of the file is a data record. Each record consists of one or more fields, separated by a delimiter such as a comma(,). Let’s take a CSV file (Employee.csv) to convert that into JSON.
Employee.csv
Employee Id, Employee Name, Age, Email Id, Date of Joining
101,Rahul Singh,25,[email protected],20-08-2014
102,Neeraj Kumar,30,[email protected],05-01-2012
103,Raj Kumar Yadav,27,[email protected],15-12-2017
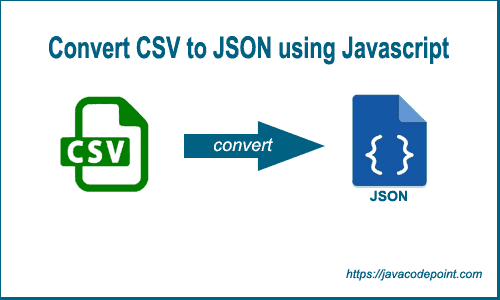
Table of Contents
Convert CSV to JSON Javascript
In this approach, we will upload a CSV file using the HTML <input type="file">
tag and read the file content using the FileReader
class in string format. Then split the string using “\r\n” to get all employee records in an Array. Then loop through the array and split each record with Comma(,) delimiter. Finally, create a JSON object for each record and pushed that into the resulting JSON Array. Let’s follow the below steps:-
- Upload a CSV file using an HTML
<input>
file type element. - Read CSV files using
readAsBinaryString()
method of FileReader class in string. - Convert the data to a string and split it into an array.
- Create a separate array of headers and read the first record as CSV file headers.
- Loop through the remaining records and split each with a delimiter comma(,) and create a JSON object.
- Add the created JSON object to the result JSON array.
CSV file to JSON Example in Javascript
See below the complete code for converting a CSV file data into JSON format in javascript. In this example, we will take the above Employee.csv file as input.
<!DOCTYPE html>
<html>
<head>
<title>CSV to JSON | Javacodepoint</title>
</head>
<body>
<center><h2>Upload a CSV file to convert in JSON</h2>
<!-- Input element to upload an csv file -->
<input type="file" id="file_upload" />
<button onclick="upload()">Upload</button>
<br>
<br>
<!-- container to display the csv data -->
<div id="display_csv_data"></div>
</center>
<script>
// Method to upload a valid csv file
function upload() {
var files = document.getElementById('file_upload').files;
if(files.length==0){
alert("Please choose any file...");
return;
}
var filename = files[0].name;
var extension = filename.substring(filename.lastIndexOf(".")).toUpperCase();
if (extension == '.CSV') {
//Here calling another method to read CSV file into json
csvFileToJSON(files[0]);
}else{
alert("Please select a valid csv file.");
}
}
//Method to read csv file and convert it into JSON
function csvFileToJSON(file){
try {
var reader = new FileReader();
reader.readAsBinaryString(file);
reader.onload = function(e) {
var jsonData = [];
var headers = [];
var rows = e.target.result.split("\r\n");
for (var i = 0; i < rows.length; i++) {
var cells = rows[i].split(",");
var rowData = {};
for(var j=0;j<cells.length;j++){
if(i==0){
var headerName = cells[j].trim();
headers.push(headerName);
}else{
var key = headers[j];
if(key){
rowData[key] = cells[j].trim();
}
}
}
//skip the first row (header) data
if(i!=0){
jsonData.push(rowData);
}
}
//displaying the json result in string format
document.getElementById("display_csv_data").innerHTML=JSON.stringify(jsonData);
}
}catch(e){
console.error(e);
}
}
</script>
</body>
</html>
OUTPUT:
[{“Employee Id”:”101″,”Employee Name”:”Rahul Singh”,”Age”:”25″,”Email Id”:”[email protected]”,”Date of Joining”:”20-08-2014″},{“Employee Id”:”102″,”Employee Name”:”Neeraj Kumar”,”Age”:”30″,”Email Id”:”[email protected]”,”Date of Joining”:”05-01-2012″},{“Employee Id”:”103″,”Employee Name”:”Raj Kumar Yadav”,”Age”:”27″,”Email Id”:”[email protected]”,”Date of Joining”:”15-12-2017″}]
Convert CSV file to human-readable JSON format
The output of the above example is in plain JSON string format which is quite difficult to read as a human. In this example, we will convert the CSV file data into human-readable JSON format. Here we will use the same above code but only the change is we use JSON.stringify() method with some extra parameters to make that JSON easy to read.
The JSON.stringify() method takes the third argument which is a spacer. The spacer is a string or number that’s used to insert white space (including indentation, line break characters, etc.) into the output JSON string for readability purposes. For example- JSON.stringify(jsonData, null, 4). Let’s see the complete code below:
<!DOCTYPE html>
<html>
<head>
<title>CSV to JSON | Javacodepoint</title>
</head>
<body>
<center><h2>Upload a CSV file to convert in human readable JSON</h2>
<!-- Input element to upload an csv file -->
<input type="file" id="file_upload" />
<button onclick="upload()">Upload</button>
<br>
<br>
<!-- container to display the csv data -->
<textarea id="display_data_in_readable_format" style="height:400px;width:400px;"></textarea>
</center>
<script>
// Method to upload a valid csv file
function upload() {
var files = document.getElementById('file_upload').files;
if(files.length==0){
alert("Please choose any file...");
return;
}
var filename = files[0].name;
var extension = filename.substring(filename.lastIndexOf(".")).toUpperCase();
if (extension == '.CSV') {
//Here calling another method to read CSV file into json
csvFileToJSON(files[0]);
}else{
alert("Please select a valid csv file.");
}
}
//Method to read csv file and convert it into JSON
function csvFileToJSON(file){
try {
var reader = new FileReader();
reader.readAsBinaryString(file);
reader.onload = function(e) {
var jsonData = [];
var headers = [];
var rows = e.target.result.split("\r\n");
for (var i = 0; i < rows.length; i++) {
var cells = rows[i].split(",");
var rowData = {};
for(var j=0;j<cells.length;j++){
if(i==0){
var headerName = cells[j].trim();
headers.push(headerName);
}else{
var key = headers[j];
if(key){
rowData[key] = cells[j].trim();
}
}
}
//skip the first row (header) data
if(i!=0){
jsonData.push(rowData);
}
}
//displaying the json result in string format
document.getElementById("display_data_in_readable_format").value=JSON.stringify(jsonData,null,4);
}
}catch(e){
console.error(e);
}
}
</script>
</body>
</html>
OUTPUT:
[
{
“Employee Id”: “101”,
“Employee Name”: “Rahul Singh”,
“Age”: “25”,
“Email Id”: “[email protected]”,
“Date of Joining”: “20-08-2014”
},
{
“Employee Id”: “102”,
“Employee Name”: “Neeraj Kumar”,
“Age”: “30”,
“Email Id”: “[email protected]”,
“Date of Joining”: “05-01-2012”
},
{
“Employee Id”: “103”,
“Employee Name”: “Raj Kumar Yadav”,
“Age”: “27”,
“Email Id”: “[email protected]”,
“Date of Joining”: “15-12-2017”
}
]
Create and Download JSON files in Javascript
In this example, we will create a JSON file and download it from the browser. Here also we will use the above code, only we write a new createAndDownloadJsonFile(jsonData) method for converting JSON object data into JSON file, which will be called once the CSV file data is converted into JSON object format. Let’s see the complete code below:
<!DOCTYPE html>
<html>
<head>
<title>CSV to JSON | Javacodepoint</title>
</head>
<body>
<center><h2>Upload a CSV file and download in JSON</h2>
<!-- Input element to upload an csv file -->
<input type="file" id="file_upload" />
<button onclick="upload()">Upload CSV & Download JSON File</button>
</center>
<script>
// Method to upload a valid csv file
function upload() {
var files = document.getElementById('file_upload').files;
if(files.length==0){
alert("Please choose any file...");
return;
}
var filename = files[0].name;
var extension = filename.substring(filename.lastIndexOf(".")).toUpperCase();
if (extension == '.CSV') {
//Here calling another method to read CSV file into json
csvFileToJSON(files[0]);
}else{
alert("Please select a valid csv file.");
}
}
//Method to read csv file and convert it into JSON
function csvFileToJSON(file){
try {
var reader = new FileReader();
reader.readAsBinaryString(file);
reader.onload = function(e) {
var jsonData = [];
var headers = [];
var rows = e.target.result.split("\r\n");
for (var i = 0; i < rows.length; i++) {
var cells = rows[i].split(",");
var rowData = {};
for(var j=0;j<cells.length;j++){
if(i==0){
var headerName = cells[j].trim();
headers.push(headerName);
}else{
var key = headers[j];
if(key){
rowData[key] = cells[j].trim();
}
}
}
//skip the first row (header) data
if(i!=0){
jsonData.push(rowData);
}
}
createAndDownloadJsonFile(jsonData);
}
}catch(e){
console.error(e);
}
}
// Method to create and download json file
function createAndDownloadJsonFile(jsonData){
var dataStr = "data:text/json;charset=utf-8," + encodeURIComponent(JSON.stringify(jsonData,null,4));
var downloadAnchorNode = document.createElement('a');
downloadAnchorNode.setAttribute("href", dataStr);
downloadAnchorNode.setAttribute("download", "employee.json");
document.body.appendChild(downloadAnchorNode);
downloadAnchorNode.click();
downloadAnchorNode.remove();
}
</script>
</body>
</html>
CSV to JSON Converter Online
There are various converters available for converting CSV to JSON online easily. As a developer, format conversion is something we sometimes have to do. We often look online for solutions to fulfill our needs quickly. Let’s see below some links for CSV to JSON Converter online:
Related articles:
- Convert Excel file data to JSON in Javascript.
- Convert JSON data into HTML table using javascript.
- Display CSV data in HTML Table in JavaScript.