In the web application, from the security point of view file upload validations are the most important parameter that needs to be considered by each developer especially when doing the file upload. The validations can be either client-side or server-side or maybe both.
This article shows you how could you validate the File Type (Extension) and File Size before uploading it to the server. This demonstration will be shown for the client-side validation using javascript.
Table of Contents
File Type (Extension) Validation
Using Javascript, we could easily validate the file type by extracting the file extension with the allowed file types.
Example of File Type Validation
Below is the sample example for the file type validation. In this example, we upload files having extensions .jpeg/.jpg/.png/.gif only. We are going to define a javascript function validateFileType()
that will be called on the upload button click.
<!DOCTYPE html>
<html>
<head>
<title>
File type validation while uploading using JavaScript
</title>
<style>
body{
text-align:center;
}
</style>
</head>
<body>
<h1>File type validation while uploading using JavaScript</h1>
<p>Upload an image (.jpg,.jpeg,.png,.gif)</p>
<!-- input element to choose a file for uploading -->
<input type="file" id="file-upload" />
<br><br>
<!-- button element to validate file type on click event -->
<button onclick="validateFileType()">Upload</button>
<script>
/* javascript function to validate file type */
function validateFileType() {
var inputElement = document.getElementById('file-upload');
var files = inputElement.files;
if(files.length==0){
alert("Please choose a file first...");
return false;
}else{
var filename = files[0].name;
/* getting file extenstion eg- .jpg,.png, etc */
var extension = filename.substr(filename.lastIndexOf("."));
/* define allowed file types */
var allowedExtensionsRegx = /(\.jpg|\.jpeg|\.png|\.gif)$/i;
/* testing extension with regular expression */
var isAllowed = allowedExtensionsRegx.test(extension);
if(isAllowed){
alert("File type is valid for the upload");
/* file upload logic goes here... */
}else{
alert("Invalid File Type.");
return false;
}
}
}
</script>
</body>
</html>
Let’s understand the above code implementation with sample points:
- An HTML
<input>
element is defined with attribute type=”file” to choose a file for uploading. - An HTML
<button>
element is defined to call thevalidateFileType()
function on click event to validate file type before uploading. - Inside
validateFileType()
, getting inputElement by calling javascript functiongetElementById()
. - Using inputElement.files, getting the files object.
- Using files.length, checking wether user has chosen any file or not.
- Using files[0].name, getting the name of the file with extension (eg- wallpager.png).
- Defining regular expression allowedExtensionsRegx variable with allowed file types(.JPG, .JPEG, .PNG, .GIF only).
- Using the
test()
function, checking wether the file type is allowed or not. It returns boolean value true or false.
Preview & Live Demo
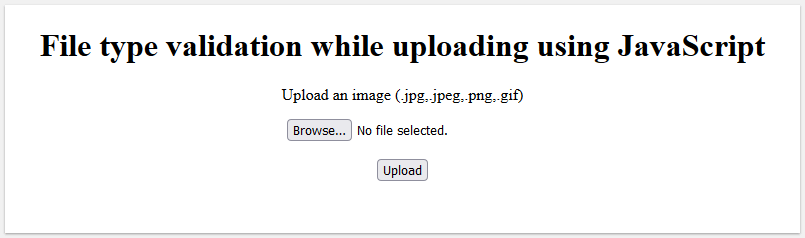
Working With Multiple Files Upload
Sometimes based on project requirements we required multiple files to upload at a time. This scenario can be handled easily with a few modifications in the above sample example.
Let’s understand the required code modification:
1.) In the HTML <input>
element, add the multiple
attribute. The multiple
attribute is used to allow choosing multiple files at a time.
See the below image to understand how it can be added to an HTML <input>
element:
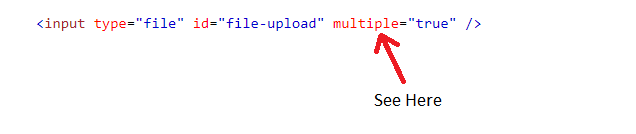
2.) The next modification is required in validateFileType()
function. Inside this function, inputElement.files will return the Array of files object. So as it is an Array, we can iterate it through a for loop and validate the file type one by one for each file.
See the below modified validateFileType()
function:
/* javascript function to validate file type */
function validateFileType() {
var inputElement = document.getElementById('file-upload');
var files = inputElement.files;
if(files.length==0){
alert("Please choose a file first...");
return false;
}else{
/* iterating over the files array */
for(var i=0;i<files.length;i++){
var filename = files[i].name;
/* getting file extenstion eg- .jpg,.png, etc */
var extension = filename.substr(filename.lastIndexOf("."));
/* define allowed file types */
var allowedExtensionsRegx = /(\.jpg|\.jpeg|\.png|\.gif)$/i;
/* testing extension with regular expression */
var isAllowed = allowedExtensionsRegx.test(extension);
if(isAllowed){
alert("File type is valid for the upload");
/* file upload logic goes here... */
}else{
alert("Invalid File Type.");
}
}
}
}
File Size Validation
The size of the uploading file can also be validated easily in javascript.
Example of File Size Validation
Below is the sample example for the file size validation. In this example, we upload files having a size less than or equal to 2MB only. We are going to define a javascript function validateFileSize()
that will be called on the upload button click.
<!DOCTYPE html>
<html>
<head>
<title>
File size validation while uploading using JavaScript
</title>
<style>
body{
text-align:center;
}
</style>
</head>
<body>
<h1>File size validation while uploading using JavaScript</h1>
<p>Upload a file (size less than or equal to 2MB) only</p>
<!-- input element to choose a file for uploading -->
<input type="file" id="file-upload" />
<br><br>
<!-- button element to validate file type on click event -->
<button onclick="validateFileSize()">Upload</button>
<script>
/* javascript function to validate file size */
function validateFileSize() {
var inputElement = document.getElementById('file-upload');
var files = inputElement.files;
if(files.length==0){
alert("Please choose a file first...");
return false;
}
var fileSize = files[0].size;
/* 1024 = 1MB */
var size = Math.round((fileSize / 1024));
/* checking for less than or equals to 2MB file size */
if (size <= 2*1024) {
alert("Valid file size");
/* file uploading code goes here... */
} else {
alert(
"Invalid file size, please select a file less than or equal to 2mb size");
}
}
</script>
</body>
</html>
Let’s understand the above code implementation with sample points:
- An HTML
<input>
element is defined with attribute type=”file” to choose a file for uploading. - An HTML
<button>
element is defined to call thevalidateFileSize()
function on click event to validate file size before uploading. - Inside
validateFileSize()
, getting inputElement by calling javascript functiongetElementById()
. - Using inputElement.files, getting the files object.
- Using files.length, checking wether user has chosen any file or not.
- Using files[0].size, getting the size of the file in bytes format.
- Calculating the file size in rounded figure using
Math.round()
function in MB. - Checking wether the file size is less than or equals to 2MB or not.
Preview & Live Demo
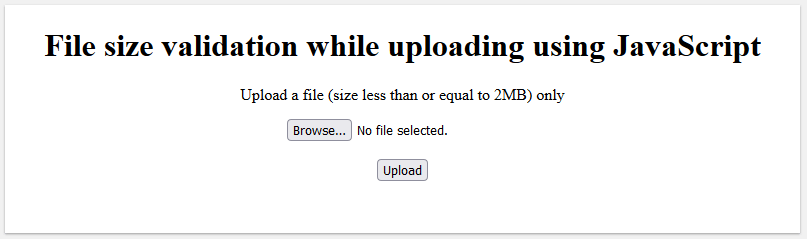
Working With Multiple Files Upload
In this case also when we are required to work with multiple files upload, we can handle it easily with few modifications in the above sample example.
Let's understand the required code modification:
1.) In the HTML <input>
element, add the multiple
attribute. The multiple
attribute is used to allow choosing multiple files at a time.
See the below image to understand how it can be added to an HTML <input>
element:
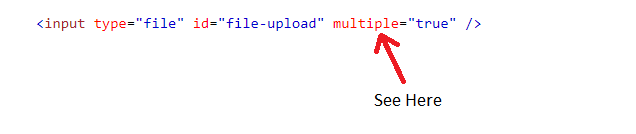
2.) The next modification is required in validateFileSize()
function. Inside this function, inputElement.files will return the Array of files object. So here also, we can iterate it through a for loop and validate the file size one by one for each file.
See the below modified validateFileSize()
function:
/* javascript function to validate file size */
function validateFileSize() {
var inputElement = document.getElementById('file-upload');
var files = inputElement.files;
if(files.length==0){
alert("Please choose a file first...");
return false;
}
/* iterating over the files array */
for(var i=0; i<files.length; i++){
var fileSize = files[i].size;
/* 1024 = 1MB */
var size = Math.round((fileSize / 1024));
/* checking for less than or equals to 2MB file size */
if (size <= 2*1024) {
alert("Valid file size");
/* file uploading code goes here...*/
} else {
alert(
"Invalid file size, please select a file less than or equal to 2mb size");
}
}
}
Conclusion
In this article, you have seen how to validate file type and file size before uploading it to the server. You have also learned how to work with multiple file upload validation using Javascript.
Also, learn how to validate file upload using jQuery
If you want to learn how to do file upload to the server with server-side file validation in Java you can visit the below links:
Spring Boot File Upload with Advance Progress bar in Ajax
File Upload in Java Servlet Example