This article shows you how to read HTML table data in JSON using JavaScript. Sometimes we are showing the data in an HTML table. So we are required to read or get the data from the HTML table. There are multiple ways to read or get table data in JSON format. Here you will learn a few simple ways for reading data from an HTML table. You will also see read dynamic table row data in the Bootstrap table.
Table of Contents
How to read HTML table data?
Reading the data from an HTML table is very simple. Let’s assume we have a simple Employee’s table as follows-
Employee Id | Employee Name | Mobile | Email Id |
---|---|---|---|
101 | Akash Kumar | 9850009000 | akash.k@gmail.com |
102 | Johnson Matthey | 8899776655 | johnson.m@gmail.com |
103 | Williamson | 9000088800 | williamson103@gmail.com |
OUTPUT: (Table data in JSON string format)
[{“Employee Id”:”101″,”Employee Name”:”Akash Kumar”,”Mobile”:”9850009000″,”Email Id”:”akash.k@gmail.com”},{“Employee Id”:”102″,”Employee Name”:”Johnson Matthey”,”Mobile”:”8899776655″,”Email Id”:”johnson.m@gmail.com”},{“Employee Id”:”103″,”Employee Name”:”Williamson”,”Mobile”:”9000088800″,”Email Id”:”williamson103@gmail.com”}]
Step to get data from a table in javascript
- Get HTML table reference object using table ID. eg-
var eTable = document.getElementById('employee-table');
- Get the number of row counts in the table. eg-
var rowLength = eTable.rows.length;
- Loops through all rows and get the all cells for each row. eg-
var oCells = eTable.rows.item(i).cells;
- Get the number of cell counts in the current row. eg-
var cellLength = oCells.length;
- Loops through each cell in the current row and get the cell value. eg-
var cellVal = oCells.item(j).innerHTML;
Get data from a table in JSON Example
Let’s see the complete code for reading the data from an HTML table in JSON format using Javascript.
<!DOCTYPE html>
<html>
<head>
<title>Read HTML Table Data | Javacodepoint</title>
<style>
#container{
margin:20px auto;
width:800px;
}
#employee-table{
width:800px;
border: 1px solid #aaa;
}
</style>
</head>
<body>
<div id="container">
<table id="employee-table">
<thead>
<tr><th>Employee Id</th><th>Employee Name</th><th>Mobile</th><th>Email Id</th></tr>
</thead>
<tbody>
<tr><td>101</td><td>Akash Kumar</td><td>9850009000</td><td>akash.k@gmail.com</td></tr>
<tr><td>102</td><td>Johnson Matthey</td><td>8899776655</td><td>johnson.m@gmail.com</td></tr>
<tr><td>103</td><td>Williamson</td><td>9000088800</td><td>williamson103@gmail.com</td></tr>
</tbody>
</table>
<br><br>
<button type="button" onclick="readTableData()">Read Data</button>
<br><br>
<div id="total-data"></div>
</div>
</body>
<script type="text/javascript">
/* This method will read the table data in json format */
function readTableData(){
//gets table
var eTable = document.getElementById('employee-table');
//gets rows of table
var rowLength = eTable.rows.length;
var totalEmp = [];
var headers = [];
//loops through rows
for (i = 0; i < rowLength; i++){
//gets cells of current row
var oCells = eTable.rows.item(i).cells;
//gets count of cells of current row
var cellLength = oCells.length;
var rowData = {};
//loops through each cell in current row
for(var j = 0; j < cellLength; j++){
if(i==0){
//reading the table headers
/* get your cell info here */
var cellVal = oCells.item(j).innerHTML;
headers.push(cellVal);
}else{
//reading the table data
var cellVal = oCells.item(j).innerHTML;
var headerName = headers[j];
rowData[headerName] = cellVal;
}
}
//skip adding first row (header row) to total record
if(i!=0){
totalEmp.push(rowData);
}
}
document.getElementById("total-data").innerHTML=JSON.stringify(totalEmp);
}
</script>
</html>
OUTPUT:
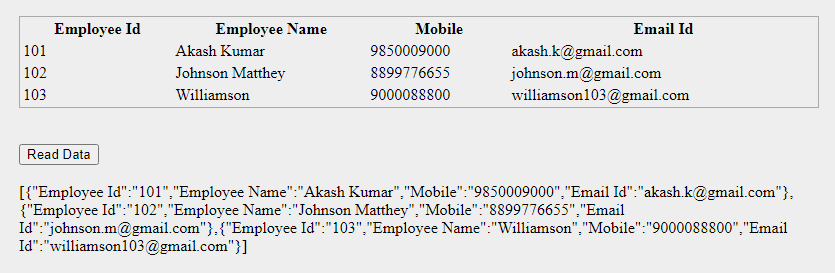
Explanation of the above Example
- Here we have created an HTML table for employee data (Employee Id, Employee Name, Mobile, Email Id) with
id="employee-table"
. - We have defined a button (Read Data) to read data from the table. And we are calling a
readTableData()
method on this button click. - The
readTableData()
is a javascript method we have defined for reading the data and setting results to a div component (id="total-data"
). - In
readTableData()
method, we are getting all rows and iterating them and iterating each cell of each row and reading each cell value. - In this example, we are reading the table data and converting them into a JSON object array.
- The first row of data is the header of the table, so we save it in different ‘headers’ variables and skip it to store it in the ‘totalEmp’ array.
- Finally, converting the JSON data into string format and displaying it on the div component.
Read Dynamic Table Data using JavaScript Example
In this example, we will see the following points in a Bootstrap table:
- Dynamically add and remove the table row on a button click.
- Read the dynamically created table row data in JSON format.
- Display the prepared JSON data on the screen.
Here, we have created a few JavaScript function for the above point such as addNewRow()
, deleteRow()
, and readTableData()
. Let’s see the complete code example below:
<!DOCTYPE html>
<html>
<head>
<title>Read Dynamic Table Data | Javacodepoint</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.3/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
<style>
#container{
margin:0px auto;
text-align:center;
}
</style>
</head>
<body>
<div id="container" class="container-fluid">
<h3>Read Dynamic Bootstrap Table Data using Javascript</h3>
<button onclick="addNewRow()" class="btn btn-primary">Add New Row</button>
<button onclick="readTableData()" class="btn btn-primary">Read Table Data</button>
<button onclick="deleteRow()" class="btn btn-danger">Delete Row</button>
<br><br>
<table id="employee-table" class="table table-bordered table-striped">
<tr>
<th>First Name</th>
<th>Last Name</th>
<th>Mobile No</th>
<th>Email ID</th>
<th>Action</th>
</tr>
</table>
<br><br>
<div id="total-data"></div>
</div>
</body>
<script type="text/javascript">
/* This method will add a new row */
function addNewRow(){
var table = document.getElementById("employee-table");
var rowCount = table.rows.length;
var cellCount = table.rows[0].cells.length;
var row = table.insertRow(rowCount);
for(var i =0; i < cellCount; i++){
var cell = row.insertCell(i);
if(i < cellCount-1){
cell.innerHTML='<input type="text" class="form-control" />';
}else{
cell.innerHTML = '<input class="btn btn-danger" '
+' type="button" value="delete" onclick="deleteRow(this)" />';
}
}
}
/* This method will read the table data in json format */
function readTableData(){
//gets table
var eTable = document.getElementById('employee-table');
//gets rows of table
var rowLength = eTable.rows.length;
var totalEmp = [];
var headers = [];
//loops through rows
for (i = 0; i < rowLength; i++){
//gets cells of current row
var oCells = eTable.rows.item(i).cells;
//gets count of cells of current row
var cellLength = oCells.length;
var rowData = {};
//loops through each cell (except last cell) in current row
for(var j = 0; j < cellLength-1; j++){
if(i==0){
//reading the table headers
/* get your cell info here */
var cellVal = oCells.item(j).innerHTML;
headers.push(cellVal);
}else{
//reading the table data
var cellVal = oCells.item(j).childNodes[0].value;
var headerName = headers[j];
rowData[headerName] = cellVal;
}
}
//skip adding first row (header row) to total record
if(i!=0){
totalEmp.push(rowData);
}
}
document.getElementById("total-data").innerHTML=JSON.stringify(totalEmp);
}
/* This method will delete a row */
function deleteRow(ele){
var table = document.getElementById('employee-table');
var rowCount = table.rows.length;
if(rowCount <= 1){
alert("There is no row available to delete!");
return;
}
if(ele){
//delete specific row
ele.parentNode.parentNode.remove();
}else{
//delete last row
table.deleteRow(rowCount-1);
}
}
</script>
</html>
OUTPUT:
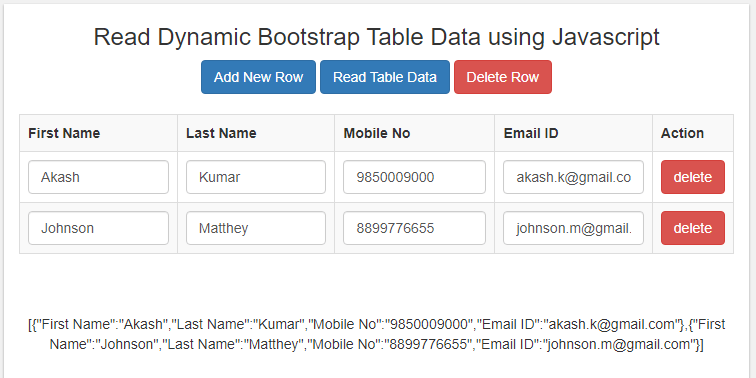
How to convert JSON to readable format in JavaScript?
Sometime the string JSON format is hard to read meaning we can not understand easily, so to overcome this problem we should convert that string JSON to readable format using JSON.stringify() method.
JSON.stringify(jsonObj, replacer, space):Â method to convert JavaScript objects into strings in readable format. In the below example, we use space size 4. It display the JSON object in pretty human readable format.
<!DOCTYPE html>
<html>
<head>
<title>Convert JSON in Readable format | Javacodepoint</title>
</head>
<body>
<h2>Convert JSON string to readable format</h2>
<h3>String JSON Format:</h3>
<p id="json-string"></p>
<h3>Readable JSON Format:</h3>
<pre id="readable-json" style="color:blue;"></pre>
</body>
<script type="text/javascript">
var jsonData= [{"Employee Id":"101","Employee Name":"Akash Kumar","Mobile":"9850009000","Email Id":"akash.k@gmail.com"},{"Employee Id":"102","Employee Name":"Johnson Matthey","Mobile":"8899776655","Email Id":"johnson.m@gmail.com"},{"Employee Id":"103","Employee Name":"Williamson","Mobile":"9000088800","Email Id":"williamson103@gmail.com"}];;
document.getElementById("json-string").innerHTML=JSON.stringify(jsonData);
document.getElementById("readable-json").innerHTML=JSON.stringify(jsonData, null, 4);
</script>
</html>
OUTPUT:
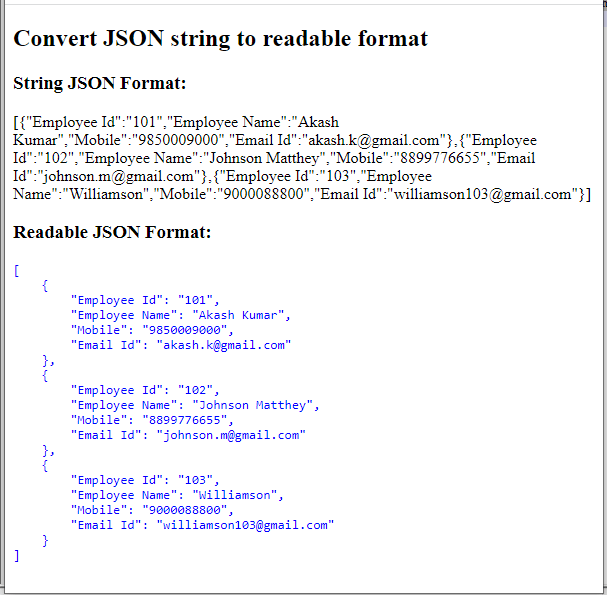
Conclusion
In this article, you have seen the reading of html table data in JSON format using JavaScript. You have also seen how you can read dynamically created table row data in bootstrap table. Here, you have also seen how to convert string JSON to human readable JSON format.
Read about dynamic table row creation here: Dynamically add/remove table rows in JavaScript.
FAQ
How to get Html table cell value in JavaScript?
To get the cell value in JavaScript, you can use the below code if you know the table id.
JavaScript code:
var eTable = document.getElementById(‘example-table-id’);
var rowLength = eTable.rows.length;
for (i = 0; i < rowLength; i++){
var oCells = eTable.rows.item(i).cells;
var cellLength = oCells.length;
for(var j = 0; j < cellLength; j++){
var cellVal = oCells.item(j).innerHTML;
}
}
How to get row numbers in an Html table using javascript?
JavaScript code to get row numbers:
var eTable = document.getElementById(‘example-table-id’);
var rowLength = eTable.rows.length;
for (i = 0; i < rowLength; i++){
// here i contains the row number of table
}