In this article, you will see add/remove the table rows dynamically using JavaScript. You will also see how can you do the same in the Bootstrap table.
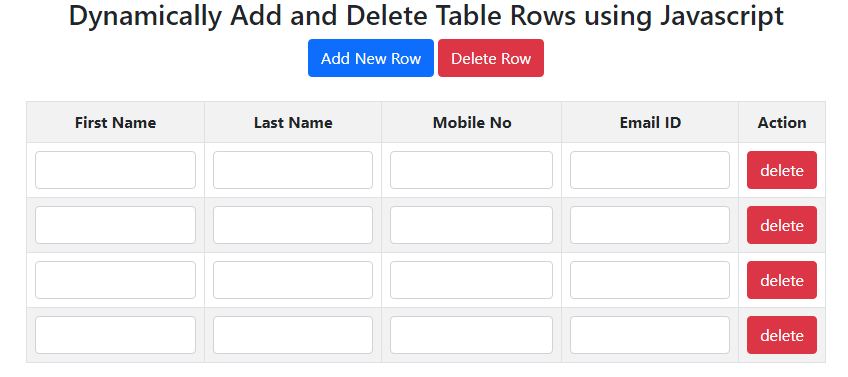
Table of Contents
Overview
Sometimes we are required to add or delete the records (table rows) from the HTML table at runtime. We can add as many records as we want to add and delete any record that we want to delete from the HTML table. We will do the same functionality here with the Bootstrap table also to make that responsive.
How do you add and delete HTML table rows in JavaScript?
Here we have defined an addNewRow() method to add a new row (a record) and a deleteRow() method to delete the existing row from the table. Let’s understand each one step by step.
JavaScript add rows to the table dynamically
1.) Create an empty HTML table with table headers. eg.–
<table id="employee-table">
<tr>
<th>First Name</th>
<th>Last Name</th>
<th>Mobile No</th>
<th>Email ID</th>
<th>Action</th>
</tr>
</table>
2.) To add a row dynamically, create a JavaScript method say addNewRow(), and call it on a button click.
3.) Inside addNewRow(), find the rowCount and insert the new row at the bottom of the table. eg.-
var table = document.getElementById("employee-table");
var rowCount = table.rows.length;
var row = table.insertRow(rowCount);
4.) Now find the cellCount and iterate that many times to create a cell and insert it into a created row. eg.-
var cellCount = table.rows[0].cells.length;
for(var i =0; i < cellCount; i++){
var cell = row.insertCell(i);
if(i < cellCount-1){
cell.innerHTML='<input type="text" />';
}else{
cell.innerHTML = '<input type="button" value="delete" onclick="deleteRow(this)" />';
}
}
Steps: Javascript deletes rows from the table dynamically
1.) To delete the table row, define a JavaScript method say deleteRow(), and call it on a button click.
2.) Inside deleteRow(), find the rowCount, and if the count is more than 1 then go for the deletion. eg.-
var table = document.getElementById('employee-table');
var rowCount = table.rows.length;
if(rowCount <= 1){
alert("There is no row available to delete!");
return;
}
//go for the deletion here...
3.) Now based on the calling place of deleteRow(), whether we want to delete a specific row or the last row need to write the code as follows:-
//ele is an argument to this method
if(ele){
//delete specific row
ele.parentNode.parentNode.remove();
}else{
//delete last row
table.deleteRow(rowCount-1);
}
Complete example to add and delete table rows dynamically
<!DOCTYPE html>
<html>
<head>
<title>Add/Delete Table Rows | Javacodepoint</title>
<style>
#container{
margin:0px auto;
width:800px;
text-align:center;
}
#employee-table{
width:800px;
border: 1px solid #aaa;
}
</style>
</head>
<body>
<div id="container">
<h2>Dynamically Add and Delete Table Rows using Javascript</h2>
<button onclick="addNewRow()">Add New Row</button>
<button onclick="deleteRow()">Delete Row</button>
<br><br>
<table id="employee-table">
<tr><th>First Name</th><th>Last Name</th><th>Mobile No</th><th>Email ID</th><th>Action</th></tr>
</table>
</div>
</body>
<script type="text/javascript">
/* This method will add a new row */
function addNewRow(){
var table = document.getElementById("employee-table");
var rowCount = table.rows.length;
var cellCount = table.rows[0].cells.length;
var row = table.insertRow(rowCount);
for(var i =0; i < cellCount; i++){
var cell = row.insertCell(i);
if(i < cellCount-1){
cell.innerHTML='<input type="text" />';
}else{
cell.innerHTML = '<input type="button" value="delete" onclick="deleteRow(this)" />';
}
}
}
/* This method will delete a row */
function deleteRow(ele){
var table = document.getElementById('employee-table');
var rowCount = table.rows.length;
if(rowCount <= 1){
alert("There is no row available to delete!");
return;
}
if(ele){
//delete specific row
ele.parentNode.parentNode.remove();
}else{
//delete last row
table.deleteRow(rowCount-1);
}
}
</script>
</html>
OUTPUT:
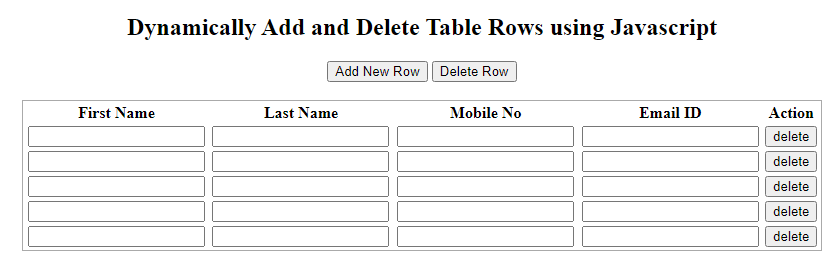
How do you add and delete rows from the bootstrap table in JavaScript?
Here we will use the code to do this, the only thing is we will do it for the bootstrap table to make it responsive. In order to use bootstrap we have to import the library into the HTML document. The below .css and .js library import we are going to use in this example:-
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.3/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
Now we have to use bootstrap CSS classes such as table, table-bordered, table-striped, etc. to create a bootstrap table.
Complete example for adding and removing table rows dynamically
<!DOCTYPE html>
<html>
<head>
<title>Add/Delete Table Rows | Javacodepoint</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.3/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
<style>
#container{
margin:0px auto;
text-align:center;
}
</style>
</head>
<body>
<div id="container" class="container-fluid">
<h3>Dynamically Add and Delete Table Rows using Javascript</h3>
<button onclick="addNewRow()" class="btn btn-primary">Add New Row</button>
<button onclick="deleteRow()" class="btn btn-danger">Delete Row</button>
<br><br>
<table id="employee-table" class="table table-bordered table-striped">
<tr>
<th>First Name</th>
<th>Last Name</th>
<th>Mobile No</th>
<th>Email ID</th>
<th>Action</th>
</tr>
</table>
</div>
</body>
<script type="text/javascript">
/* This method will add a new row */
function addNewRow(){
var table = document.getElementById("employee-table");
var rowCount = table.rows.length;
var cellCount = table.rows[0].cells.length;
var row = table.insertRow(rowCount);
for(var i =0; i < cellCount; i++){
var cell = row.insertCell(i);
if(i < cellCount-1){
cell.innerHTML='<input type="text" class="form-control" />';
}else{
cell.innerHTML = '<input class="btn btn-danger" '
+' type="button" value="delete" onclick="deleteRow(this)" />';
}
}
}
/* This method will delete a row */
function deleteRow(ele){
var table = document.getElementById('employee-table');
var rowCount = table.rows.length;
if(rowCount <= 1){
alert("There is no row available to delete!");
return;
}
if(ele){
//delete specific row
ele.parentNode.parentNode.remove();
}else{
//delete last row
table.deleteRow(rowCount-1);
}
}
</script>
</html>
OUTPUT:
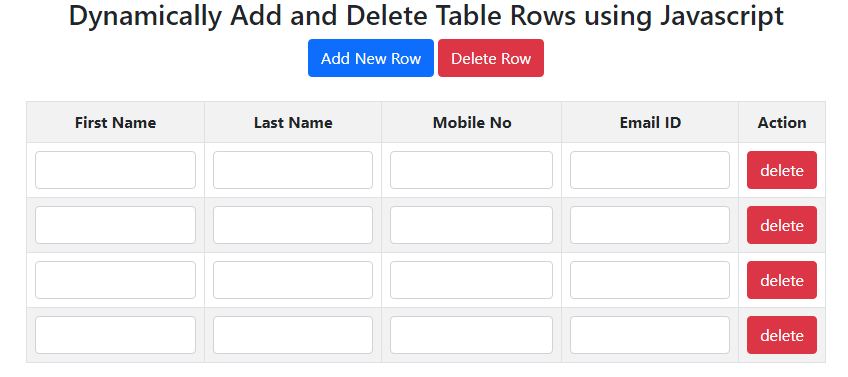
Conclusion
In this article, you have seen how you add and delete HTML table rows dynamically using JavaScript. Here you have also seen to work with the bootstrap table to make it responsive.
If you want to do the same using jQuery, then visit another article here How to Add and Delete Rows Dynamically using jQuery.