This tutorial explains Apache POI RichTextString with sample examples. The RichTextString
is an interface used to create Rich text Unicode strings. These strings can have fonts applied to arbitrary parts of the string.
To apply different formatting to different parts of a cell, you need to use RichTextString
, which permits the styling of parts of the text within the cell. In another word, if you want to apply multiple font styles to a single Excel cell content, then you can use the implementation class of the interface RichTextString
.
There are two known implementation classes HSSFRichTextString
and XSSFRichTextString
that we will explain in this article with examples.
Table of Contents
Apache POI – RichTextString (Interface)
The RichTextString is an interface available under the package org.apache.poi.ss.usermodel in the Apache POI library. The following table describes the list of methods available in this interface:
void applyFont(Font font) – Sets the font of the entire string. |
void applyFont(short fontIndex) – Applies the specified font to the entire string. |
void applyFont(int startIndex, int endIndex, Font font) – Applies a font to the specified characters of a string. |
void applyFont(int startIndex, int endIndex, short fontIndex) – Applies a font to the specified characters of a string. |
void clearFormatting() – Removes any formatting that may have been applied to the string. |
String getString() – Returns the plain string representation. |
int length() – Return the number of characters in the font. |
int numFormattingRuns() – Return The number of formatting runs used. |
int getIndexOfFormattingRun(int index) – The index within the string to which the specified formatting run applies. |
Reference: RichTextString (POI API Documentation).
Apache POI – HSSFRichTextString (Class)
The HSSFRichTextString is an implementation class of the RichTextString interface. It is mainly used to create an Excel (XLS) 2003 or below file format. The following code snippet illustrates the example.
HSSF Example
// HSSF Example
Workbook wb = new HSSFWorkbook();
Sheet sheet = wb.createSheet();
Row row = sheet.createRow(1);
HSSFCell hssfCell = (HSSFCell) row.createCell(1);
HSSFFont font1 = (HSSFFont) wb.createFont();
font1.setBold(true);
font1.setColor(IndexedColors.BLUE.getIndex());
HSSFFont font2 = (HSSFFont) wb.createFont();
font2.setItalic(true);
font2.setUnderline(HSSFFont.U_DOUBLE);
font2.setColor(IndexedColors.GREEN.getIndex());
// Rich text consists of two fonts
HSSFRichTextString richString = new HSSFRichTextString("Hello, World!");
richString.applyFont(0, 6, font1);
richString.applyFont(6, 13, font2);
hssfCell.setCellValue(richString);
OUTPUT:
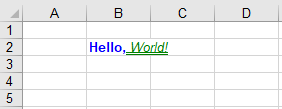
Note:
In certain cases creating too many HSSFRichTextString cells may cause Excel 2003 and lower to crash when changing the color of the cells and then saving the Excel file.
Apache POI – XSSFRichTextString (Class)
The XSSFRichTextString is another implementation class of the RichTextString interface. This class is mainly used to create an Excel (XLSX) 2007 or later file format. The following code snippet illustrates the example.
XSSF Example
// XSSF Example
Workbook wb = new XSSFWorkbook();
Sheet sheet = wb.createSheet();
Row row = sheet.createRow(1);
XSSFCell xssfCell = (XSSFCell) row.createCell(1);
XSSFFont font1 = (XSSFFont) wb.createFont();
font1.setFontName("Bauhaus 93");
font1.setColor(IndexedColors.DARK_BLUE.getIndex());
XSSFFont font2 = (XSSFFont) wb.createFont();
font2.setFontName("Bauhaus 93");
font2.setColor(IndexedColors.LIGHT_BLUE.getIndex());
// Rich text consists of two fonts
XSSFRichTextString richString = new XSSFRichTextString("JavaCodePoint");
richString.applyFont(0, 4, font1);
richString.applyFont(4, 8, font2);
richString.applyFont(8, 13, font1);
xssfCell.setCellValue(richString);
OUTPUT:
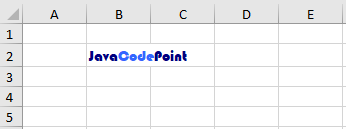
Apache POI RichTextString Cell Multiple Style Example
In this example, you will understand how you can create multiple styles and applied to a single cell in Excel with XSSFRichTextString using Apache POI. Here, we will create two Rich text strings with different-different font styles.
package com.javacodepoint.excel;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import org.apache.poi.ss.usermodel.IndexedColors;
import org.apache.poi.ss.usermodel.RichTextString;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFCell;
import org.apache.poi.xssf.usermodel.XSSFFont;
import org.apache.poi.xssf.usermodel.XSSFRichTextString;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
/**
* Apply multiple fonts in a single cell Example
*/
public class RichTextStringExample {
// main method
public static void main(String[] args) throws FileNotFoundException, IOException {
/*
* Creating a workbook for XLSX file using XSSFWorkbook, Use HSSFWorkbook for
* XLS File
*/
Workbook wb = new XSSFWorkbook();
Sheet sheet = wb.createSheet();
Row row = sheet.createRow(1);
XSSFCell xssfCell = (XSSFCell) row.createCell(1);
XSSFFont font1 = (XSSFFont) wb.createFont();
font1.setFontName("Bauhaus 93");
font1.setColor(IndexedColors.DARK_BLUE.getIndex());
XSSFFont font2 = (XSSFFont) wb.createFont();
font2.setFontName("Bauhaus 93");
font2.setColor(IndexedColors.LIGHT_BLUE.getIndex());
// Rich text consists of two fonts
RichTextString richString = new XSSFRichTextString("JavaCodePoint");
richString.applyFont(0, 4, font1);
richString.applyFont(4, 8, font2);
richString.applyFont(8, 13, font1);
xssfCell.setCellValue(richString);
// Creating another Rich text string
XSSFFont font3 = (XSSFFont) wb.createFont();
font3.setBold(true);
XSSFFont font4 = (XSSFFont) wb.createFont();
font4.setItalic(true);
XSSFFont font5 = (XSSFFont) wb.createFont();
font5.setUnderline(XSSFFont.U_DOUBLE);
font5.setColor(IndexedColors.RED.getIndex());
// create another row
row = sheet.createRow(3);
XSSFCell cell = (XSSFCell) row.createCell(1);
XSSFRichTextString richString2 = new XSSFRichTextString("This is another example of");
richString2.applyFont(0, 15, font3);
richString2.applyFont(15, 26, font4);
richString2.append(" XSSFRichTextString", font5);
cell.setCellValue(richString2);
// Write the output to a file
try (OutputStream fileOut = new FileOutputStream("RichTextStringExample.xlsx")) {
wb.write(fileOut);
}
// close the workbook
wb.close();
// Print the confirmation message
System.out.println("An Excel file has been created successfully!");
}
}
OUTPUT:
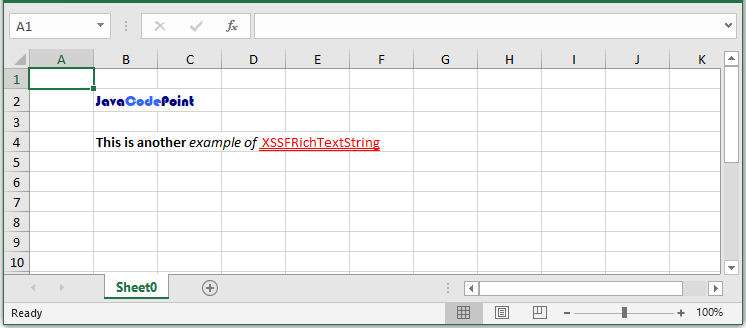
Conclusion
In this tutorial, you have seen HSSF and XSSF RichTextString to create multiple styles in a single cell of Excel. There are some slight differences between HSSF and XSSF, especially around font colors (the two formats store colors quite differently internally).
References: HSSFRichTextString, XSSFRichTextString.
Related Articles:
- Apache POI Excel Cell Color in Java.
- Apache POI Excel Cell Border in Java.
- Apache POI Excel Cell Alignment in Java.