This Apache POI tutorial shows you about setting Excel cell Alignment in Java. The Apache POI is an open-source Java API library for manipulating the various Microsoft files format such as Excel, PowerPoint, and Word in Java.
Table of Contents
How to set Excel cell alignment using Apache POI?
Steps to set Excel cell alignment in Java:
- Create a Cell using
createCell()
method. - Set some cell content in it using
setCellValue()
method. - Create a cell style using
createCellStyle()
method. - Set the Horizontal and Vertical alignment properties using
setAlignment()
andsetVerticalAlignment()
method respectively. - Finally, apply the created cell style to the Excel cell using
setCellStyle()
method.
Classes and Methods for Align Excel Cell – Apache POI
HorizontalAlignment: The enumeration value indicating the horizontal alignment of a cell, i.e., whether it is aligned general, left, right, horizontally centered, filled (replicated), justified, centered across multiple cells, or distributed.
VerticalAlignment: This enumeration value indicates the type of vertical alignment for a cell, i.e., whether it is aligned top, bottom, vertically centered, justified, or distributed.
createCell(): This is a method to create new cells within the row and return it.
setCellValue(): Set the cell value of supported datatypes.
createCellStyle(): Create a new Cell style and add it to the workbook’s style table.
setAlignment(): Set the type of horizontal alignment for the cell.
setVerticalAlignment(): Set the type of vertical alignment for the cell.
setCellStyle(): Set the style for the cell. The style should be a CellStyle created/retrieved from the Workbook.
All available alignment properties with their description in HorizontalAlignment
:
Properties | Description |
---|---|
LEFT | Aligns contents at the left edge of the cell. |
RIGHT | The cell contents are aligned at the right edge of the cell, even in Right-to-Left mode. |
CENTER | The text is horizontally centered and align across the cell. |
CENTER_SELECTION | The horizontal alignment is centered across multiple cells. |
GENERAL | Text data is left-aligned. Numbers, dates, and times are right aligned. Boolean types are centered. Changing the alignment does not change the type of data. |
FILL | Indicates that the value of the cell should be filled across the entire width of the cell. |
JUSTIFY | The horizontal alignment is justified (flush left and right). For each line of text, aligns each line of the wrapped text in a cell to the right and left (except the last line). If no single line of text wraps in the cell, then the text is not justified. |
DISTRIBUTED | Indicates that each ‘word’ in each line of text inside the cell is evenly distributed across the width of the cell, with flush right and left margins. When there is also an indent value to apply, both the left and right sides of the cell are padded by the indent value. |
All available alignment properties with their description in VerticalAlignment
:
Properties | Description |
---|---|
TOP | The vertical alignment is aligned to the top. |
CENTER | The vertical alignment is centered across the height of the cell. |
BOTTOM | The vertical alignment is aligned to the bottom. (typically the default value) |
JUSTIFY | When text direction is horizontal: the vertical alignment of lines of text is distributed vertically, where each line of text inside the cell is evenly distributed across the height of the cell, with flush top and bottom margins. When text direction is vertical: similar behavior as horizontal justification. The alignment is justified (flush top and bottom in this case). For each line of text, each line of the wrapped text in a cell is aligned to the top and bottom (except the last line). If no single line of text wraps in the cell, then the text is not justified. |
DISTRIBUTED | When text direction is horizontal: the vertical alignment of lines of text is distributed vertically, where each line of text inside the cell is evenly distributed across the height of the cell, with a flush top. When text direction is vertical: it behaves exactly as distributed horizontal alignment. The first words in a line of text (appearing at the top of the cell) are flush with the top edge of the cell, and the last words of a line of text are flush with the bottom edge of the cell, and the line of text is distributed evenly from top to bottom. |
Cell alignment in Excel file using Apache POI Example
In this example, we are going to create an XLSX file “xssf-align-example.xlsx” with different Horizontal alignment as well as Vertical alignment properties. Here we are defining createCellWithAlignment() method to create and set the cell alignment. Let’s see the java code below:
CellAlignmentsExcelExample.java
package com.javacodepoint.excel;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.CellStyle;
import org.apache.poi.ss.usermodel.HorizontalAlignment;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.VerticalAlignment;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
/**
* An example of cell alignments in Excel file
*/
public class CellAlignmentsExcelExample {
/*
* Main method
*/
public static void main(String[] args) throws FileNotFoundException, IOException {
/*
* Creating a workbook for XLSX file using XSSFWorkbook, Use HSSFWorkbook for
* XLS File
*/
Workbook wb = new XSSFWorkbook();
Sheet sheet = wb.createSheet("Cell Align Example");
// Creating a new row at index 2
Row row = sheet.createRow(2);
// Setting row height to see the alignment properly
row.setHeightInPoints(30);
// Creating multiple column with different cell align in the same row
createCellWithAlignment(wb, row, 0, HorizontalAlignment.CENTER, VerticalAlignment.BOTTOM);
createCellWithAlignment(wb, row, 1, HorizontalAlignment.CENTER_SELECTION, VerticalAlignment.BOTTOM);
createCellWithAlignment(wb, row, 2, HorizontalAlignment.FILL, VerticalAlignment.CENTER);
createCellWithAlignment(wb, row, 3, HorizontalAlignment.GENERAL, VerticalAlignment.CENTER);
createCellWithAlignment(wb, row, 4, HorizontalAlignment.JUSTIFY, VerticalAlignment.JUSTIFY);
createCellWithAlignment(wb, row, 5, HorizontalAlignment.LEFT, VerticalAlignment.TOP);
createCellWithAlignment(wb, row, 6, HorizontalAlignment.RIGHT, VerticalAlignment.TOP);
// Write the output to a file
try (OutputStream fileOut = new FileOutputStream("xssf-align-example.xlsx")) {
wb.write(fileOut);
}
// Close the workbook
wb.close();
// Print the confirmation message
System.out.println("An Excel file has been created successfully!");
}
/**
* Method to create a cell and align it in a certain way.
*/
private static void createCellWithAlignment(Workbook wb, Row row, int columnIndex, HorizontalAlignment hAlign,
VerticalAlignment vAlign) {
// Create a cell at specified column index
Cell cell = row.createCell(columnIndex);
// Setting the cell value
cell.setCellValue("Align It");
// Create a cell style and setting its property
CellStyle cellStyle = wb.createCellStyle();
cellStyle.setAlignment(hAlign);
cellStyle.setVerticalAlignment(vAlign);
// Finally setting the created style to cell
cell.setCellStyle(cellStyle);
}
}
OUTPUT:
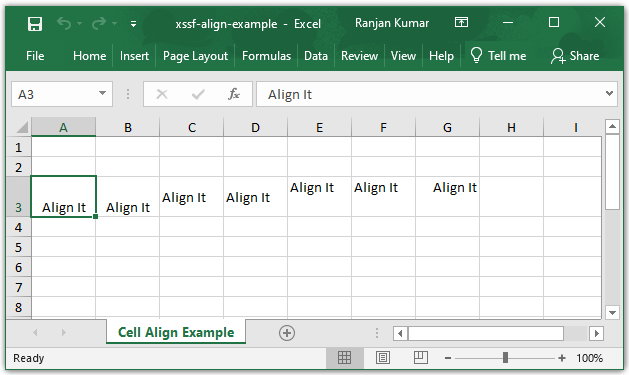
Apache POI – Environment setup in Eclipse
There are two ways for installing Apache POI in Eclipse, based on your project type:
- Maven Project
- Stand-alone Java Project (Non-Maven)
Note: In this article, we have used the Apache POI-4.0.1 version of the library to test this example.
Maven Project
If you are going to create a maven project then you have to add the following maven dependency in the pom.xml file of your project:
<!-- Used for Excel 2003 or before (xls) -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.0.1</version>
</dependency>
<!-- Used for Excel 2007 or later (xlsx) -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.0.1</version>
</dependency>
Stand-alone Java Project (Non-Maven)
If you are going to create a standalone java project then you have to add the following jar files to your java build path:
- poi-4.0.1.jar
- poi-ooxml-4.0.1.jar
- poi-ooxml-schemas-4.0.1.jar
- xmlbeans-3.0.2.jar
- curvesapi-1.05.jar
- commons-codec-1.11.jar
- commons-collections4-4.2.jar
- commons-compress-1.18.jar
- commons-math3-3.6.1.jar
You can easily download all the above jars in one place:Â Download Apache POI Jars.
If you want to know the complete environment setup for Apache POI in Eclipse IDE, follow another article here: Apache POI – Getting Started
Conclusion
In this tutorial, you have learned about setting the Excel cell alignment property using the Apache POI library in Java.
FAQ
What is horizontal alignment in Apache POI Java?
A public enum HorizontalAlignment extends java.lang.Enum<HorizontalAlignment>Â The enumeration value indicating the horizontal alignment of a cell, i.e., whether it is aligned general, left, right, horizontally centered, filled (replicated), justified, centered across multiple cells, or distributed.
How to center align in Excel in Java?
There is a CENTER property available in the public enum HorizontalAlignment, as well as VerticalAlignment to set the cell text, align centered. Now set the vertical and horizontal alignment to the cellStyle and set this style to the cell which will align the text to the center.
Example:
CellStyle cellStyle = wb.createCellStyle();
cellStyle.setAlignment(HorizontalAlignment.CENTER);
cellStyle.setVerticalAlignment(VerticalAlignment.CENTER);
cell.setCellStyle(cellStyle);
Related Articles:
- Apache POI – Read and Write Excel files in java
- How to create password-protected Excel in java?
- How to Read password-protected Excel in java?
- How to write data to an existing Excel file in java?