In this article of most common CSS properties Part-1, you will see the commonly used properties such as colors, backgrounds, height, width, margin, padding, borders, and box model.
Table of Contents
CSS Colors
In CSS, color properties are used to set the text color, background color, border color, etc of an HTML element. The followings are a few color CSS properties:
color
background-color
border-color
CSS Text Color
You can set the text color of an HTML element using color
property of CSS.
Javacodepoint – Passionate about coding
This is a paragraph in red color.
This is another paragraph in green color.
Example
<h3 style="color:Orange;">Javacodepoint – Passionate about coding</h3>
<p style="color:Red;">This is a paragraph in red color.</p>
<p style="color:Green;">This is another paragraph in green color.</p>
CSS Background Color
You can set the background color of an HTML element using background-color
property of CSS.
Javacodepoint – Passionate about coding
This is a paragraph with a light blue background color.
This is a paragraph with a yellow background color.
Example
<h3 style="background-color:#DDDDDD">Javacodepoint - Passionate about coding</h3>
<p style="background-color:LightBlue;">
This is a paragraph with a light blue background color.
</p>
<p style="background-color:Yellow;">
This is a paragraph with a yellow background color.
</p>
CSS Border Color
You can set the border color of an HTML element using border
property of CSS.
This is a Heading
This is another Heading
This is a sample paragraph.
Example
<h3 style="border:1px solid red;"> This is a Heading </h3>
<h3 style="border:1px solid green;"> This is another Heading </h3>
<p style="border:1px solid #0000ff;"> This is a sample paragraph. </p>
CSS Backgrounds
In CSS, background properties are used to set the background color, background image, background-repeat, etc of an HTML element. Followings are a few background CSS properties:
background-color
background-image
background-repeat
background-position
background
(Shorthand property)
CSS Background Color
You can set the background color of an HTML element using background-color
property of CSS. As you have seen already in the CSS Colors section.
CSS Background Image
You can set the background image of an HTML element using background-image
property. You should have an image file to use this property. The value of the background-image
property will be a url( ), which takes the path of an image file.
For example:
body{
background-image:url("wallpaper.png");
}
By default, the background-image
property repeats an image both horizontally and vertically. If you want to modify it, then by using background-repeat
property it can be modified.
The values for this are no-repeat, repeat-x, repeat-y, etc. For example:
body{
background-image:url("wallpaper.png");
background-repeat:no-repeat;
}
div{
background-image:url("wallpaper2.png");
background-repeat: repeat-x;
}
CSS Background (Shorthand property)
The background
property is a shorthand property used to specify the multiple values in a single line to background
property.
For example:
body{
background-color:#ffffff;
background-image:url("wallpaper.png");
background-repeat:no-repeat;
}
/* These property can be defined with shorthand like this */
body{
background:#fffff url("wallpaper.png") no-repeat;
}
CSS Height & Width
The height
and width
properties are used to set the height and width of an element.
The height and width properties do not include padding, borders, or margins. It sets the height/width of the area inside the padding, border, and margin of the element.
The value for the height
and width
properties are the following:
Values | Description |
---|---|
auto | This is the default value. The browser calculates the height and width automatically. |
length | Defines the height and width in percent, px, cm, etc. |
initial | Sets the height and width to its default value. |
inherit | The height and width value will be inherited from its parent value. |
Example
div.square{
height:200px;
width:200px;
background-color:pink;
}
div.rectangle{
height:200px;
width:400px;
background-color:green;
}
div.percentsize{
height:200px;
width:50%;
background-color:rgb(255,255,0);
}
div.autosize{
height:auto;
width:auto;
background-color:#00f;
}
CSS Margins & Padding
The CSS margin
properties are used to create space around elements, outside of any defined borders. Whereas the CSS padding
properties are used to generate space around an element’s content, inside of any defined borders.
The value for the margin
and padding
properties are the following:
Values | Description |
---|---|
auto | The browser calculates the value automatically. |
length | Defines the margin/padding in percent, px, cm, etc. |
inherit | The margin/padding value will be inherited from its parent value. |
NOTE: Negative values are allowed for margin but not allowed for padding.
Individual Sides
There are properties for setting the margin for each side of an element (top, right, bottom, and left).
margin-top
margin-right
margin-bottom
margin-left
Similarly, there are properties for setting the padding for each side of an element.
padding-top
padding-right
padding-bottom
padding-left
Example
.paragraph-with-margin{
margin-top:25px;
margin-right:30px;
margin-bottom:50px;
margin-left:100px;
border:1px solid red;
}
.paragraph-with-padding{
padding-top:25px;
padding-right:25px;
padding-bottom:25px;
padding-left:25px;
border:1px solid green;
}
Shorthand Property
To shorten the code, it is possible to specify all the margin/padding properties in one property.
The value for the margin
and padding
property can have one value, two values, three values, or four values.
/* four values (top=30px, right=20px, bottom=40px, left=50px) */
p{
margin: 30px 20px 40px 50px;
}
/* three values (top=30px, right=40px, bottom=50px, left=40px) */
p{
margin: 30px 40px 50px;
}
/* two values (top and bottom=30px, left and right=20px) */
p{
margin: 30px 20px;
}
/* one value (all side 50px) */
p{
margin: 50px;
}
NOTE: Similarly the above four types value applicable in padding property also.
Example
.paragraph-with-margin{
margin:25px 30px 50px 100px;
border:1px solid red;
}
.paragraph-with-padding{
padding:25px;
border:1px solid green;
}
CSS Borders
The border properties allow you to specify the border of an element. There are three properties of a border you can specify:
Properties | Description |
---|---|
border-width | Specifies the width of a border. |
border-style | Specifies the style of a border such as solid, dashed line, double line, etc. |
border-color | Specifies the color of a border. |
CSS Border Width
The border-width
property specifies how much width size should be for a border of an HTML element. The value of this property could be either a length in px, pt, cm, etc.
Example
#first-paragraph{
border-width:1px;
border-style:solid;
}
#second-paragraph{
border-width:5px;
border-style:solid;
}
#third-paragraph{
border-width:10px;
border-style:solid;
}
OUTPUT:
first paragraph with border-width 1px
second paragraph with border-width 5px
third paragraph with border-width 10px
You can also individually specify the width of the top, right, bottom, and left borders of an element using the following properties:
border-top-width
border-right-width
border-bottom-width
border-left-width
Example
p{
border-top-width:2px;
border-right-width:5px;
border-bottom-width:10px;
border-left-width:20px;
border-style:solid;
border-color:red;
}
OUTPUT:
Some paragraph with individual border width size
CSS Border Style
The border-style
 property specifies how border-style should look. The value of this property could be one of the followings:
Values | Description |
---|---|
solid | The border is a single solid line. |
dotted | The border is a series of dots. |
dashed | The border is a series of short lines. |
double | The border is two solid lines. |
groove | The border looks as though it is carved into the page. |
ridge | The border looks the opposite of the groove. |
inset | The border makes the box look like it is embedded in the page. |
outset | The border makes the box look like it is coming out of the canvas. |
none | No border, same as border-width:0px; |
hidden | Same as none. |
Example
#first-paragraph{
border-style:solid;
}
#second-paragraph{
border-style:dashed;
}
#third-paragraph{
border-style:double;
}
#fourth-paragraph{
border-style:dotted;
}
OUTPUT:
first paragraph with border-style solid
second paragraph with border-style dashed
third paragraph with border-style double
third paragraph with border-style dotted
You can also individually specify the style of the top, right, bottom, and left borders of an element using the following properties:
border-top-style
border-right-style
border-bottom-style
border-left-style
Example
p{
border-top-style:dotted;
border-right-style:solid;
border-bottom-style:dashed;
border-left-style:double;
border-color:red;
}
OUTPUT:
Some paragraph with individual border style
CSS Border (Shorthand Property)
The border
 property allows you to specify the color, style, and width of the border in a single property.
This shorthand border property is the most commonly used CSS property to set all three properties into a single property.Â
Example
p{
border:1px solid red;
}
OUTPUT:
Some paragraph with shorthand border property
CSS Rounded Borders
The border-radius
 property is used to add rounded borders to an HTML element. The value for this property is either in percentage, px, cm, etc.
Example
#paragraph1{
border : 2px solid #22f;
border-radius : 5px;
}
#paragraph2{
border : 4px double green;
border-radius : 10px;
}
#circle{
height : 200px;
width : 200px;
border : 2px solid orange;
border-radius : 50%;
}
OUTPUT:
First paragraph with rounded border
Second paragraph with rounded border
You can also individually specify the rounded border of the four corners using the following properties:
border-top-right-radius
border-bottom-right-radius
border-top-left-radius
border-bottom-left-radius
Example
#example1{
border : 2px solid green;
border-top-left-radius : 15px;
border-top-right-radius : 15px;
border-bottom-right-radius : 5px;
border-bottom-left-radius : 5px;
}
#example2{
height : 100px;
width : 100px;
border : 2px solid orange;
border-top-right-radius : 50%;
}
#example3{
height : 100px;
width : 250px;
border : 2px solid green;
border-top-right-radius : 30px;
border-bottom-right-radius : 30px;
}
#example4{
height : 100px;
width : 250px;
border : 2px solid red;
border-top-left-radius : 50px;
border-bottom-right-radius : 50px;
}
#example5{
height : 100px;
width : 250px;
border : 1px solid #000;
background-color:#ff2;
border-top-left-radius : 50px;
border-top-right-radius : 50px;
border-bottom-right-radius : 50px;
border-bottom-left-radius : 50px;
}
OUTPUT:
Some examples of rounded borders
CSS Box Model
In CSS, each element in an HTML page is represented as a box model. The box model represents margins, borders, padding, and the actual content as you can see in the below image:
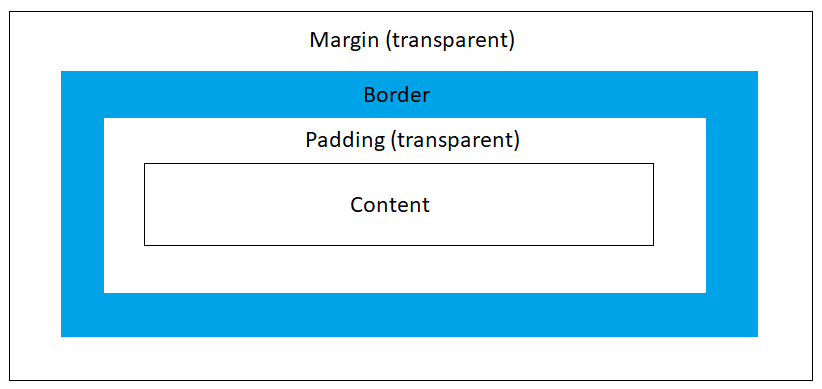
Content | Content includes the actual data in the form of text, images, or other media content. |
Margin | Spaces around outside the border. It is transparent. |
Padding | Spaces around inside the border. It is also transparent. |
Border | A border that goes around the padding and content. By default, its width is set to 0. |
Example
div {
width: 400px;
border: 20px solid lightblue;
padding: 50px;
margin: 20px;
}
Height & Width Calculation
Here, we have defined the size of the <div>
element with the following CSS properties:
div{
height:100px;
width:300px;
margin:20px;
padding:10px;
border:20px solid #4366f2;
}
Total width = 400px which is calculated as follows:
Width of the element +
left margin +
right margin +
left padding +
right padding +
left border +
right border
300px + 20px + 20px + 10px + 10px + 20px + 20px = 400px
Total height = 200px which is calculated as follows:
Height of the element +
top margin +
bottom margin +
top padding +
bottom padding +
top border +
bottom border
100px + 20px + 20px + 10px + 10px + 20px + 20px = 200px
Conclusion
In this article of most common CSS properties part-1, you have seen the frequently used CSS properties such as
Here you have also seen the shorthand property for some CSS property which are very popular to specify the styles.