Are you a web developer looking to enhance your skills in HTML, CSS, and JavaScript? If so, you’re in the right place! In this beginner-friendly tutorial, we’ll walk you through creating an age calculator that takes a user’s date of birth and displays their age in years, months, and days. Plus, we’ll make sure it looks great with a user-friendly design. So, grab your coding tools, and let’s get started!
Table of Contents
Prerequisites
Before we dive into the code, make sure you have the following:
- A text editor like Notepad++, Edit Plus, or Visual Studio Code.
- Basic knowledge of HTML, CSS, and JavaScript.
- Enthusiasm to learn and explore.
Setting Up Your HTML (Age calculator HTML code)
Let’s start by creating the structure of our age calculator using HTML. Open your text editor and create a new HTML file. Here’s a basic HTML template to get you started:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Age Calculator</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="container">
<h1>Age Calculator</h1>
<div class="form">
<label for="dob">Date of Birth:</label>
<input type="date" id="dob">
<button onclick="calculateAge()">Calculate Age</button>
</div>
<div class="result">
<p>Your age is:</p>
<p id="ageResult">0 years, 0 months, 0 days</p>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
In this code, we’ve created a basic HTML structure with an input field to enter the date of birth and a button to trigger the age calculation. We also added a placeholder for displaying the calculated age.
Styling with CSS
To make our age calculator visually appealing, let’s add some CSS to a separate styles.css
file. Here’s a simple CSS stylesheet to get you started:
body {
font-family: Arial, sans-serif;
background-color: #f5f5f5;
margin: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.container {
background-color: #fff;
padding: 20px;
border-radius: 10px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
text-align: center;
}
h1 {
color: #333;
}
.form {
margin: 20px 0;
}
label {
font-weight: bold;
}
input[type="date"] {
padding: 5px;
border: 1px solid #ccc;
border-radius: 5px;
font-size: 16px;
}
button {
background-color: #007BFF;
color: #fff;
border: none;
padding: 10px 20px;
border-radius: 5px;
font-size: 16px;
cursor: pointer;
}
.result {
margin-top: 20px;
font-size: 18px;
}
This CSS code will give your age calculator a clean and user-friendly design.
Calculating Age with JavaScript
Now, let’s add the functionality to calculate the age using JavaScript. Create a script.js
file and add the following code:
function calculateAge() {
const dobInput = document.getElementById('dob').value;
const dob = new Date(dobInput);
const currentDate = new Date();
const ageInMilliseconds = currentDate - dob;
const ageInYears = ageInMilliseconds / (365 * 24 * 60 * 60 * 1000);
const age = Math.floor(ageInYears);
currentDate.setFullYear(currentDate.getFullYear() - age);
const monthDiff = currentDate.getMonth() - dob.getMonth();
let months = monthDiff;
if (currentDate.getDate() < dob.getDate()) {
months--;
}
let days = currentDate.getDate() - dob.getDate();
if (days < 0) {
const lastDayOfMonth = new Date(currentDate.getFullYear(), currentDate.getMonth(), 0).getDate();
days = lastDayOfMonth - dob.getDate() + currentDate.getDate();
}
document.getElementById('ageResult').textContent = `${age} years, ${months} months, ${days} days`;
}
This JavaScript code calculates the age based on the input date of birth and updates the result on the webpage.
Age Calculator using HTML, CSS, Javascript (complete code)
You can also combine all the above code into a single HTML file and give it a title like “Interactive Age Calculator with HTML, CSS, and JavaScript.” Here’s the complete code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Interactive Age Calculator with HTML, CSS, and JavaScript</title>
<style>
body {
font-family: Arial, sans-serif;
background-color: #f5f5f5;
margin: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.container {
background-color: #fff;
padding: 20px;
border-radius: 10px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
text-align: center;
}
h1 {
color: #333;
}
.form {
margin: 20px 0;
}
label {
font-weight: bold;
}
input[type="date"] {
padding: 5px;
border: 1px solid #ccc;
border-radius: 5px;
font-size: 16px;
}
button {
background-color: #007BFF;
color: #fff;
border: none;
padding: 10px 20px;
border-radius: 5px;
font-size: 16px;
cursor: pointer;
}
.result {
margin-top: 20px;
font-size: 18px;
}
</style>
</head>
<body>
<div class="container">
<h1>Age Calculator</h1>
<div class="form">
<label for="dob">Date of Birth:</label>
<input type="date" id="dob">
<button onclick="calculateAge()">Calculate Age</button>
</div>
<div class="result">
<p>Your age is:</p>
<p id="ageResult">0 years, 0 months, 0 days</p>
</div>
</div>
<script>
function calculateAge() {
const dobInput = document.getElementById('dob').value;
const dob = new Date(dobInput);
const currentDate = new Date();
const ageInMilliseconds = currentDate - dob;
const ageInYears = ageInMilliseconds / (365 * 24 * 60 * 60 * 1000);
const age = Math.floor(ageInYears);
currentDate.setFullYear(currentDate.getFullYear() - age);
const monthDiff = currentDate.getMonth() - dob.getMonth();
let months = monthDiff;
if (currentDate.getDate() < dob.getDate()) {
months--;
}
let days = currentDate.getDate() - dob.getDate();
if (days < 0) {
const lastDayOfMonth = new Date(currentDate.getFullYear(), currentDate.getMonth(), 0).getDate();
days = lastDayOfMonth - dob.getDate() + currentDate.getDate();
}
document.getElementById('ageResult').textContent = `${age} years, ${months} months, ${days} days`;
}
</script>
</body>
</html>
Testing Your Age Calculator
Now that everything is set up, open your HTML file in a web browser and test your age calculator. Enter your date of birth and click the “Calculate Age” button to see the magic happen!
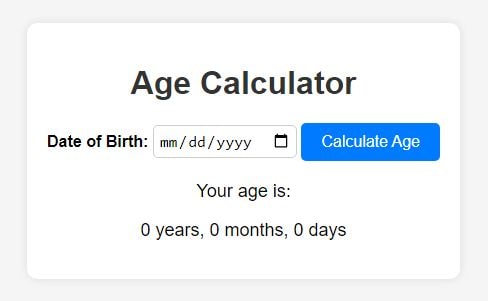
Congratulations! You’ve successfully created an age calculator using HTML, CSS, and JavaScript. This project not only helps you practice your coding skills but also provides a practical tool for calculating ages. Feel free to customize the design and functionality further to make it your own.
Happy coding!