This article shows you how can you convert JSON to HTML table using Javascript. It also shows you to display the JSON data in the Bootstrap table.
Table of Contents
Overview
Javascript JSON is a standard key-value pair (text-based format) for representing structured data based on Javascript object syntax. It is commonly used for transferring data in web applications such as sending data from the server to the client or vice-versa.
The JSON object format is a little technical for the end user to understand, so displaying the JSON data on the web page in HTML table format is very helpful for the end user to understand.
In this article, you will learn to display JSON data in an HTML table using javascript as well as display JSON data objects in a responsive bootstrap table.
How do convert JSON to a Table?
To convert a JSON object to an HTML table, we should have some JSON. Let’s assume, we have employee records in the form of a JSON object which contains the employee information (employee name, address, email id, and age) as follow:
[
{
"Employee Name":"Rahul Singh",
"Address":"Hyderabad",
"Email ID":"rahul.singh@gmail.com",
"Age":25
},
{
"Employee Name":"Pawan Patil",
"Address":"Mumbai",
"Email ID":"pawan.patil@gmail.com",
"Age":27
},
{
"Employee Name":"karl Jablonski",
"Address":"Seattle",
"Email ID":"jablonski.karl@gmail.com",
"Age":25
},
{
"Employee Name":"Jhon Smith",
"Address":"New Yark",
"Email ID":"smith.jh@gmail.com",
"Age":22
}]
Javascript code to show JSON data in HTML
Let’s see the javascript code with a complete example to create a table from a JSON object as follow:
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>JSON to HTML Table | Javacodepoint</title>
</head>
<body>
<h1>Convert JSON data to simple HTML Table</h1>
<button onclick="convertJsonToHtmlTable()">Convert JSON to HTML Table</button>
<br>
<br>
<!-- table to display the json data -->
<table id="display_json_data" border="1"></table>
<script>
var employeeJson = [
{
"Employee Name":"Rahul Singh",
"Address":"Hyderabad",
"Email ID":"rahul.singh@gmail.com",
"Age":25
},
{
"Employee Name":"Pawan Patil",
"Address":"Mumbai",
"Email ID":"pawan.patil@gmail.com",
"Age":27
},
{
"Employee Name":"karl Jablonski",
"Address":"Seattle",
"Email ID":"jablonski.karl@gmail.com",
"Age":25
},
{
"Employee Name":"Jhon Smith",
"Address":"New Yark",
"Email ID":"smith.jh@gmail.com",
"Age":22
}];
function convertJsonToHtmlTable(){
//Get the headers from JSON data
var headers = Object.keys(employeeJson[0]);
//Prepare html header
var headerRowHTML='<tr>';
for(var i=0;i<headers.length;i++){
headerRowHTML+='<th>'+headers[i]+'</th>';
}
headerRowHTML+='</tr>';
//Prepare all the employee records as HTML
var allRecordsHTML='';
for(var i=0;i<employeeJson.length;i++){
//Prepare html row
allRecordsHTML+='<tr>';
for(var j=0;j<headers.length;j++){
var header=headers[j];
allRecordsHTML+='<td>'+employeeJson[i][header]+'</td>';
}
allRecordsHTML+='</tr>';
}
//Append the table header and all records
var table=document.getElementById("display_json_data");
table.innerHTML=headerRowHTML + allRecordsHTML;
}
</script>
</body>
</html>
JSON to HTML Table Live Demo
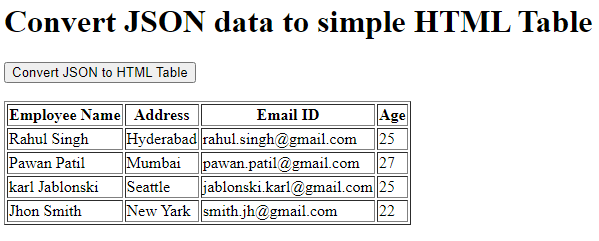
How to convert JSON to a bootstrap Table in javascript?
To make the table responsive, we create the bootstrap table. With the small modification in the above example, we can convert JSON to a bootstrap table example in javascript. Bootstrap has a lot of pre-defined class names for making tables responsive such as .table, .table-bordered, .table-striped, etc.
We have to import the following bootstrap CSS library into the <head> tag of the HTML document.
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/css/bootstrap.min.css" rel="stylesheet">
Create a bootstrap table from JSON data example
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>JSON to Bootstrap Table | Javacodepoint</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<div class="container-fluid">
<h1>Convert JSON data to Bootstrap Table</h1>
<button onclick="convertJsonToBootstrapTable()">Convert JSON to Bootstrap Table</button>
<br>
<br>
<!-- table to display the json data -->
<table id="display_json_data" class="table table-bordered table-striped"></table>
</div>
<script>
var employeeJson = [
{
"Employee Name":"Rahul Singh",
"Address":"Hyderabad",
"Email ID":"rahul.singh@gmail.com",
"Age":25
},
{
"Employee Name":"Pawan Patil",
"Address":"Mumbai",
"Email ID":"pawan.patil@gmail.com",
"Age":27
},
{
"Employee Name":"karl Jablonski",
"Address":"Seattle",
"Email ID":"jablonski.karl@gmail.com",
"Age":25
},
{
"Employee Name":"Jhon Smith",
"Address":"New Yark",
"Email ID":"smith.jh@gmail.com",
"Age":22
}];
function convertJsonToBootstrapTable(){
//Get the headers from JSON data
var headers = Object.keys(employeeJson[0]);
//Prepare html header
var headerRowHTML='<tr>';
for(var i=0;i<headers.length;i++){
headerRowHTML+='<th>'+headers[i]+'</th>';
}
headerRowHTML+='</tr>';
//Prepare all the employee records as HTML
var allRecordsHTML='';
for(var i=0;i<employeeJson.length;i++){
//Prepare html row
allRecordsHTML+='<tr>';
for(var j=0;j<headers.length;j++){
var header=headers[j];
allRecordsHTML+='<td>'+employeeJson[i][header]+'</td>';
}
allRecordsHTML+='</tr>';
}
//Append the table header and all records
var table=document.getElementById("display_json_data");
table.innerHTML=headerRowHTML + allRecordsHTML;
}
</script>
</body>
</html>
JSON To Bootstrap Table Live Demo
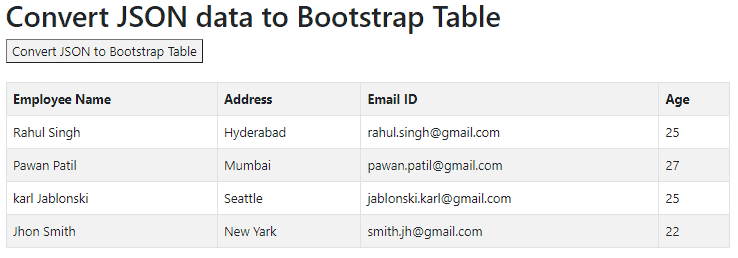
Conclusion
In this article, you have seen displaying the JSON object data to a simple HTML table as well as a bootstrap responsive table using javascript.
If you want to display an Excel file data into a table, read another article here: Display Excel data in HTML Table using SheetJS in JavaScript.