In this article, you will see how to convert JSON data into an HTML table using jQuery. You will also see how to display the JSON data in the Bootstrap table using jQuery.
Table of Contents
Overview
Javascript JSON is a standard key-value pair (text-based format) for representing structured data based on Javascript object syntax. It is commonly used for transferring data in web applications such as sending data from the server to the client or vice-versa.
The JSON object format is a little technical for the end-user to understand, so displaying the JSON data on the web page in HTML table format is very helpful for the end-user to understand.
In this article, you will learn to display JSON data in an HTML table using jquery as well as display JSON data objects in a responsive bootstrap table.
How to convert JSON to HTML table using jQuery?
To convert a JSON object to an HTML table, we should have some JSON. Let’s assume, we have employee records in the form of a JSON object which contains the employee information (employee name, address, email id, and age) as follow:
[
{
"Employee Name":"Rahul Singh",
"Address":"Hyderabad",
"Email ID":"rahul.singh@gmail.com",
"Age":25
},
{
"Employee Name":"Pawan Patil",
"Address":"Mumbai",
"Email ID":"pawan.patil@gmail.com",
"Age":27
},
{
"Employee Name":"karl Jablonski",
"Address":"Seattle",
"Email ID":"jablonski.karl@gmail.com",
"Age":25
},
{
"Employee Name":"Jhon Smith",
"Address":"New Yark",
"Email ID":"smith.jh@gmail.com",
"Age":22
}]
jQuery code to convert JSON to HTML table
Let’s see the jquery code with a complete example to create a table from a JSON object as follow:
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>JSON to HTML Table | Javacodepoint</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
</head>
<body>
<h1>Convert JSON data to simple HTML Table</h1>
<button id="button1">Convert JSON to HTML Table</button>
<br>
<br>
<!-- table container to display the json data -->
<div id="table-container"></div>
<script>
var employeeJson = [
{
"Employee Name":"Rahul Singh",
"Address":"Hyderabad",
"Email ID":"rahul.singh@gmail.com",
"Age":25
},
{
"Employee Name":"Pawan Patil",
"Address":"Mumbai",
"Email ID":"pawan.patil@gmail.com",
"Age":27
},
{
"Employee Name":"karl Jablonski",
"Address":"Seattle",
"Email ID":"jablonski.karl@gmail.com",
"Age":25
},
{
"Employee Name":"Jhon Smith",
"Address":"New Yark",
"Email ID":"smith.jh@gmail.com",
"Age":22
}];
//this function will be called when button is clicked
$("#button1").on("click",function(){
//Get the headers from JSON data
var headers = Object.keys(employeeJson[0]);
//Prepare html header
var headerRowHTML='<tr>';
$.each(headers, function(i,header){
headerRowHTML+='<th>'+header+'</th>';
});
headerRowHTML+='</tr>';
//Creating table with headers
var tableHTML='<table border="1">'+headerRowHTML+'</table>';
$("#table-container").html(tableHTML);
//Prepare all the employee records as HTML
var allRecordsHTML='';
$.each(employeeJson, function(i,row){
//Prepare html row
allRecordsHTML+='<tr>';
$.each(headers, function(j,header){
allRecordsHTML+='<td>'+row[header]+'</td>';
});
allRecordsHTML+='</tr>';
});
//Appending the all records to table
$("table").append(allRecordsHTML);
});
</script>
</body>
</html>
JSON to HTML Table Live Demo
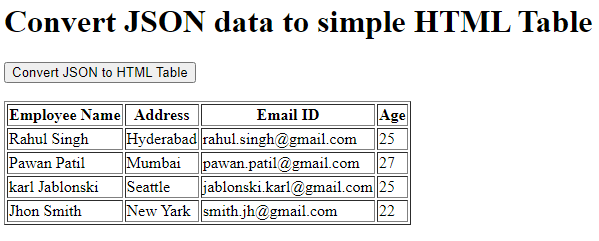
How to convert JSON to a bootstrap Table in jQuery?
To make the table responsive, we have to create the bootstrap table. With the small modification in the above example, we can convert JSON to a bootstrap table example in jquery. Bootstrap has a lot of pre-defined class names for making tables responsive such as .table, .table-bordered, .table-striped, etc.
We have to import the following libraries into the <head> tag of the HTML document.
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
Create a bootstrap table from JSON data example
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>JSON to Bootstrap Table | Javacodepoint</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
</head>
<body>
<div class="container-fluid">
<h1>Convert JSON data to Bootstrap Table</h1>
<button id="button1">Convert JSON to Bootstrap Table</button>
<br>
<br>
<!-- table container to display the json data -->
<div id="table-container"></div>
</div>
<script>
var employeeJson = [
{
"Employee Name":"Rahul Singh",
"Address":"Hyderabad",
"Email ID":"rahul.singh@gmail.com",
"Age":25
},
{
"Employee Name":"Pawan Patil",
"Address":"Mumbai",
"Email ID":"pawan.patil@gmail.com",
"Age":27
},
{
"Employee Name":"karl Jablonski",
"Address":"Seattle",
"Email ID":"jablonski.karl@gmail.com",
"Age":25
},
{
"Employee Name":"Jhon Smith",
"Address":"New Yark",
"Email ID":"smith.jh@gmail.com",
"Age":22
}];
//this function will be called when button is clicked
$("#button1").on("click",function(){
//Get the headers from JSON data
var headers = Object.keys(employeeJson[0]);
//Prepare html header
var headerRowHTML='<tr>';
$.each(headers, function(i,header){
headerRowHTML+='<th>'+header+'</th>';
});
headerRowHTML+='</tr>';
//Creating table with headers
var tableHTML='<table class="table table-bordered table-striped">'+headerRowHTML+'</table>';
$("#table-container").html(tableHTML);
//Prepare all the employee records as HTML
var allRecordsHTML='';
$.each(employeeJson, function(i,row){
//Prepare html row
allRecordsHTML+='<tr>';
$.each(headers, function(j,header){
allRecordsHTML+='<td>'+row[header]+'</td>';
});
allRecordsHTML+='</tr>';
});
//Appending the all records to table
$("table").append(allRecordsHTML);
});
</script>
</body>
</html>
JSON To Bootstrap Table Live Demo
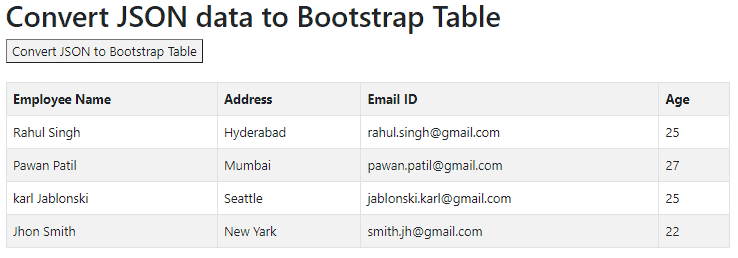
Conclusion
In this article, you have seen displaying the JSON object data to a simple HTML table as well as a bootstrap responsive table using jQuery. If you want to do the same example using pure javascript, visit the article: Convert json data into html table using javascript.
If you want to display an Excel file data into a table, read another article here: Display Excel data in HTML Table using SheetJS in JavaScript.
This article has helped me to display json data in html tables using jquery ajax. This website and this ( https://kodlogs.net/236/display-json-data-in-html-table-using-jquery-ajax ) website came in handy when I think about it. Thanks to the website authorities