Creating an ‘Age Calculator by Date of Birth‘ is a fascinating journey into the world of web development for beginners. In this project, we build an interactive web application using HTML, CSS, and JavaScript. The HTML structure sets the stage, while CSS styles give the page an appealing and user-friendly design. Users can input their date of birth into a form, click a ‘Calculate Age’ button, and the JavaScript computes their age in years, months, and days. It’s an ideal starting point for those eager to learn and experiment with web development.
Age Calculator Source Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Interactive Age Calculator with HTML, CSS, and JavaScript</title>
<style>
body {
font-family: Arial, sans-serif;
background-color: #f5f5f5;
margin: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.container {
background-color: #fff;
padding: 20px;
border-radius: 10px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
text-align: center;
}
h1 {
color: #333;
}
.form {
margin: 20px 0;
}
label {
font-weight: bold;
}
input[type="date"] {
padding: 5px;
border: 1px solid #ccc;
border-radius: 5px;
font-size: 16px;
}
button {
background-color: #007BFF;
color: #fff;
border: none;
padding: 10px 20px;
border-radius: 5px;
font-size: 16px;
cursor: pointer;
}
.result {
margin-top: 20px;
font-size: 18px;
}
</style>
</head>
<body>
<div class="container">
<h1>Age Calculator</h1>
<div class="form">
<label for="dob">Date of Birth:</label>
<input type="date" id="dob">
<button onclick="calculateAge()">Calculate Age</button>
</div>
<div class="result">
<p>Your age is:</p>
<p id="ageResult">0 years, 0 months, 0 days</p>
</div>
</div>
<script>
function calculateAge() {
const dobInput = document.getElementById('dob').value;
const dob = new Date(dobInput);
const currentDate = new Date();
const ageInMilliseconds = currentDate - dob;
const ageInYears = ageInMilliseconds / (365 * 24 * 60 * 60 * 1000);
const age = Math.floor(ageInYears);
currentDate.setFullYear(currentDate.getFullYear() - age);
const monthDiff = currentDate.getMonth() - dob.getMonth();
let months = monthDiff;
if (currentDate.getDate() < dob.getDate()) {
months--;
}
let days = currentDate.getDate() - dob.getDate();
if (days < 0) {
const lastDayOfMonth = new Date(currentDate.getFullYear(), currentDate.getMonth(), 0).getDate();
days = lastDayOfMonth - dob.getDate() + currentDate.getDate();
}
document.getElementById('ageResult').textContent = `${age} years, ${months} months, ${days} days`;
}
</script>
</body>
</html>
The JavaScript function calculateAge()
takes the user’s input date of birth and the current date, then calculates the age difference between the two. It computes the age in years, months, and days, taking into account any potential adjustments for months and days. Finally, it displays the calculated age on the webpage for the user to see. This function is triggered when the “Calculate Age” button is clicked in the age calculator application.
Test Age Calculator Application
Now, open your HTML file in a web browser and test your age calculator. Enter your date of birth and click the “Calculate Age” button to see the magic happen!
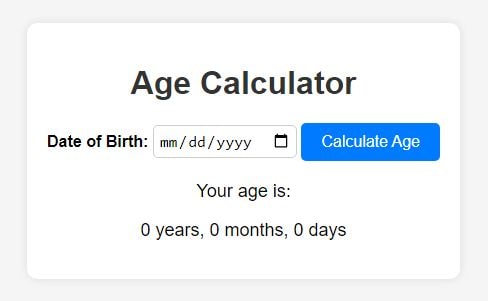
Conclusion
In this article, we explored the ‘Age Calculator by Date of Birth,’ a beginner-friendly web development project using HTML, CSS, and JavaScript. We discussed how these technologies work together to create an interactive tool. Users input their birthdate, and the JavaScript function ‘calculateAge()’ computes their age in years, months, and days, displaying the result on the webpage. It’s a practical project that not only showcases the fundamentals of web development but also provides a customizable template for learning and experimentation.
After clicking calculate age button the month shows in negative value. I couldn’t understand why. Please explain it by mail to me.