This article shows you how to create a bouncing ball animation using javascript. Here we are going to use simple HTML, CSS, and Javascript to develop this example.
Basically, we will use the setInterval() method of Javascript available in the window Object. The setInterval() method calls the callback function at the specified interval time. The setInterval() method continues calling the callback function until clearInterval() method is called.
In this article, we have used the clearInterval() method to stop bouncing the ball.
Let’s have a look at the below screenshot of a bouncing ball example.
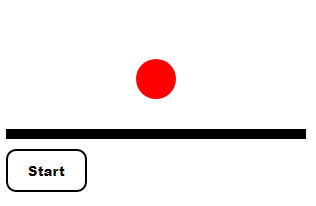
Table of Contents
The Bouncing/Jumping Ball Example using Javascript
We are going to create this example in the following steps:
- Writing HTML Code
- Writing CSS Code
- Writing Javascript Code
1. The HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Bouncing Ball</title>
</head>
<body>
<div id="container">
<div id="ball"></div>
</div>
<div id="line"></div>
<button id="start-btn" onclick="start()">Start</button>
</body>
</html>
Explanation:
- We have defined a
<div>
tag withid="container"
inside a ball will bounce. - Defined a ball with an element
<div>
withid="ball"
. - And we defined another
<div>
tag withid="line"
on which that ball will bounce. - We have defined a
<button>
to start and stop the bouncing ball.
2. The CSS Code
#container{
height:400px;
width:300px;
}
#ball{
position:absolute;
height:40px;
width:40px;
border-radius: 50%;
background:red;
margin-top:360px;
margin-left:130px;
}
#line{
width:300px;
height:10px;
background:#000;
}
#start-btn{
padding: 10px 20px;
margin-top:10px;
color:#000;
background-color:#fff;
border:2px solid #000;
border-radius:10px;
font-weight:900;
}
#start-btn:hover{
background-color:#eee;
}
3. The Javascript Code
//global variables
var timer;
var marginTop=360;
var bottomToTop=true;
//method to jump the ball
function jumpBall () {
var ball=document.getElementById("ball");
if(bottomToTop){
marginTop -=10;
}else{
marginTop +=10;
}
ball.style.marginTop= marginTop+"px";
if(marginTop==60){
bottomToTop=false;
}
if(marginTop==360){
bottomToTop=true;
}
}
//method to handle start/stop of jumping ball
function start(){
var btn=document.getElementById("start-btn");
if(btn.innerHTML.trim()==='Start'){
//start jumping the ball
timer=setInterval(function () {
jumpBall();
},100);
btn.innerHTML='Stop';
}else{
//stop jumping the ball
clearInterval(timer);
btn.innerHTML='Start';
}
}
Explanation:
- Here we have defined some global variables ie.
timer
,marginTop
,bottomToTop
. - We have defined a Timer with
setInterval()
method, called every 100 milliseconds. - Inside timer we are calling
jumpBall()
method where the bouncing ball logic has been defined. - The main logic of a bouncing ball is increasing and decreasing the
margin-top
css property of ball(<div id="ball"></div>
) based on flag variablebottomToTop
. - To handle the start and stop functionality, we have defined a
start()
method.
Complete Bouncing/Jumping Ball Example
Following is the complete code of this example(putting all HTML, CSS, and Javascript code together in a single file).
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Jumping Ball</title>
<style>
#container{
height:400px;
width:300px;
}
#ball{
position:absolute;
height:40px;
width:40px;
border-radius: 50%;
background:red;
margin-top:360px;
margin-left:130px;
}
#line{
width:300px;
height:10px;
background:#000;
}
#start-btn{
padding: 10px 20px;
margin-top:10px;
color:#000;
background-color:#fff;
border:2px solid #000;
border-radius:10px;
font-weight:900;
}
#start-btn:hover{
background-color:#eee;
}
</style>
</head>
<body>
<div id="container">
<div id="ball"></div>
</div>
<div id="line"></div>
<button id="start-btn" onclick="start()">Start</button>
<script>
//global variables
var timer;
var marginTop=360;
var bottomToTop=true;
//method to jump the ball
function jumpBall () {
var ball=document.getElementById("ball");
if(bottomToTop){
marginTop -=10;
}else{
marginTop +=10;
}
ball.style.marginTop= marginTop+"px";
if(marginTop==60){
bottomToTop=false;
}
if(marginTop==360){
bottomToTop=true;
}
}
//method to handle start/stop of jumping ball
function start(){
var btn=document.getElementById("start-btn");
if(btn.innerHTML.trim()==='Start'){
//start jumping the ball
timer=setInterval(function () {
jumpBall();
},100);
btn.innerHTML='Stop';
}else{
//stop jumping the ball
clearInterval(timer);
btn.innerHTML='Start';
}
}
</script>
</body>
</html>
Preview and Live Demo
Conclusion
In this article, you have seen how to create a Bouncing Ball animation example using javascript.