This article shows you how to create a spinning or moving wheel animation in javascript. We are going to use simple HTML, CSS, and Javascript to develop this example.
Here basically we will use the setInterval() method which is available in window Object of Javascript. The setInterval() method calls the callback function at the specified interval time (in milliseconds). The setInterval() method continues calling the callback function until clearInterval() method is called.
In this example, we have used the clearInterval() method to stop moving the wheel.
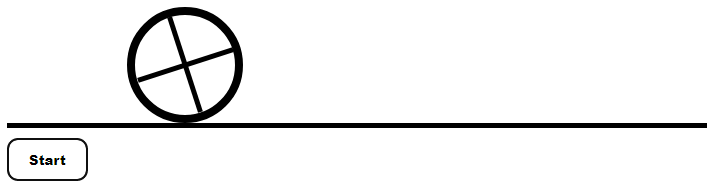
Table of Contents
How to make Spinning/Moving Wheel using Javascript?
We are going to create this example in the following steps:
- Writing HTML Code
- Writing CSS Code
- Writing Javascript Code
1. The HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Moving wheel</title>
</head>
<body>
<div id="wheel">
<div id="line1"></div>
<div id="line2"></div>
</div>
<div id="line3"></div>
<button id="start-btn" onclick="start()">Start</button>
</body>
</html>
Explanation:
- We have defined a wheel using
<div>
withid="wheel"
and inside that defined two lines withid="line1"
andid="line2"
. - We have defined a straight line using
<div>
withid="line3"
where the wheel will spin. - Defined a
<button>
element to handle start and stop functionality for the spinning wheel.
2. The CSS Code
Here we have written the CSS style for the above-defined HTML elements.
#wheel{
height:100px;
width:100px;
border-radius: 50%;
border: 8px solid black;
background:#fff;
}
#line1{
width:5px;
height:100px;
background:#000;
margin-left:48px;
}
#line2{
width:100px;
height:5px;
background:#000;
margin-top:-53px;
}
#line3{
height:5px;
width:700px;
background:#000;
}
#start-btn{
padding: 10px 20px;
margin-top:10px;
color:#000;
background-color:#fff;
border:2px solid #000;
border-radius:10px;
font-weight:900;
}
#start-btn:hover{
background-color:#eee;
}
3. The Javascript Code
//global variables
var timer;
var left=0;
var degree=0;
//method to move the wheel
function moveWheel () {
var line1=document.getElementById("line1");
var line2=document.getElementById("line2");
var wheel=document.getElementById("wheel");
left +=30;
degree+=18;
wheel.style.marginLeft= left+"px";
line1.style.transform = "rotate("+degree+"deg)";
line2.style.transform = "rotate("+degree+"deg)";
if(left==600){
left=0;
}
if(degree==360){
degree=0;
}
}
//method to handle start/stop of wheel
function start(){
var btn=document.getElementById("start-btn");
if(btn.innerHTML.trim()==='Start'){
//start moving the wheel
timer=setInterval(function () {
moveWheel();
},300);
btn.innerHTML='Stop';
}else{
//stop moving the wheel
clearInterval(timer);
btn.innerHTML='Start';
}
}
Explanation:
- First of all we have defined some global variables ie.
timer
,left
, anddegree
to hold the specific value. - We have created a timer using
setInterval()
method to call it’s callback at specified interval time(here 300 milliseconds). - Inside this timer we are calling the
moveWheel()
function where the moving logic has been defined. - The main logic for moving the wheel is, increasing the left margin of circle and rotating the circle’s middle line with specific degree.
- We have used
clearInterval()
method to pause the moving wheel. - To start and stop the moving wheel, we have defined a
start()
method which has been called on a button click.
Complete Spinning/moving wheel Example
Following is the complete code of this example(putting all HTML, CSS, and Javascript code together in a single file).
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Moving wheel</title>
<style>
#wheel{
height:100px;
width:100px;
border-radius: 50%;
border: 8px solid black;
background:#fff;
}
#line1{
width:5px;
height:100px;
background:#000;
margin-left:48px;
}
#line2{
width:100px;
height:5px;
background:#000;
margin-top:-53px;
}
#line3{
height:5px;
width:700px;
background:#000;
}
#start-btn{
padding: 10px 20px;
margin-top:10px;
color:#000;
background-color:#fff;
border:2px solid #000;
border-radius:10px;
font-weight:900;
}
#start-btn:hover{
background-color:#eee;
}
</style>
</head>
<body>
<div id="wheel">
<div id="line1"></div>
<div id="line2"></div>
</div>
<div id="line3"></div>
<button id="start-btn" onclick="start()">Start</button>
<script>
//global variables
var timer;
var left=0;
var degree=0;
//method to move the wheel
function moveWheel () {
var line1=document.getElementById("line1");
var line2=document.getElementById("line2");
var wheel=document.getElementById("wheel");
left +=30;
degree+=18;
wheel.style.marginLeft= left+"px";
line1.style.transform = "rotate("+degree+"deg)";
line2.style.transform = "rotate("+degree+"deg)";
if(left==600){
left=0;
}
if(degree==360){
degree=0;
}
}
//method to handle start/stop of wheel
function start(){
var btn=document.getElementById("start-btn");
if(btn.innerHTML.trim()==='Start'){
//start moving the wheel
timer=setInterval(function () {
moveWheel();
},300);
btn.innerHTML='Stop';
}else{
//stop moving the wheel
clearInterval(timer);
btn.innerHTML='Start';
}
}
</script>
</body>
</html>
Preview and Live Demo
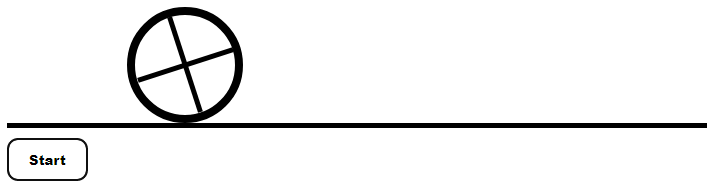
Conclusion
In this article, you have seen how to create a spinning wheel animation example using javascript.