The Java Collection Framework is an essential part of Java programming, providing powerful data structures like ArrayList, LinkedList, Stack, Queue, and PriorityQueue. Mastering collections will help you write efficient code, improve data management, and perform well in coding interviews. In this article, we cover important Java collection programs that will strengthen your understanding of lists, queues, and stacks.
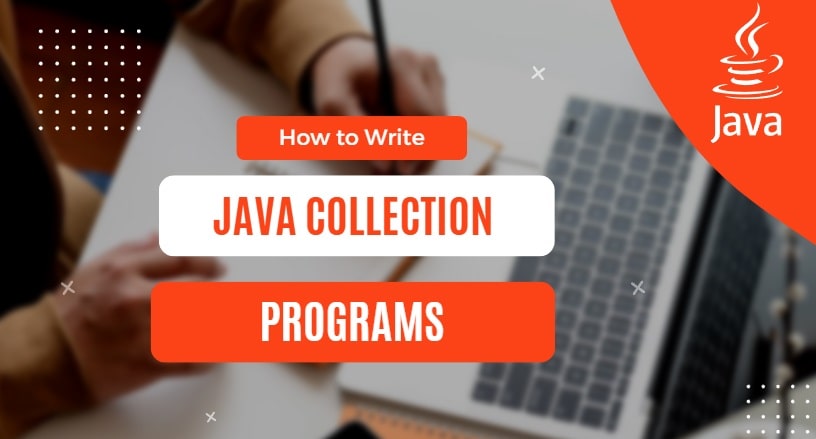
ArrayList Manipulation Programs
- ArrayList Manipulation: add, remove Elements, find size
- Write a Java program to reverse a List without using the built-in Collections.reverse() method.
- Find duplicate elements in an ArrayList
- Implement a Java program to sort an ArrayList of integer numbers.
- Implement a Java program to sort an ArrayList of custom objects based on a specific attribute.
- Create a stack using an ArrayList and implement push, pop, and peek operations.
- Convert an ArrayList of strings into a string array.
- Write a Java program to remove all elements from an ArrayList that are greater than a certain value.
- Write a Java program to remove duplicates from an ArrayList while preserving the original order.
- Write a Java program to find the common elements in two given ArrayLists.
- ArrayList partition into two separate lists of odd/even numbers.
- Write a program that extracts a sublist from an ArrayList, given a start and end index.
- Merge two sorted ArrayLists into a single sorted ArrayList.
- Find a unique string in ArrayList with its frequency
- Write a program to swap the positions of two elements in an ArrayList given their indices.
LinkedList and Queue Implementation
- Write a program to Create a Custom LinkedList in Java
- Write a Java program for Basic Queue Implementation using LinkedList
- Write a Java program to create a PriorityQueue to manage a to-do list
Why Practice Java Collection Programs?
Collections are widely used in real-world applications for data storage, retrieval, and manipulation. Understanding collections helps in solving algorithmic problems efficiently and prepares you for Java interview questions related to data structures. By practicing these programs, you will improve your coding skills and confidence for interviews. Start coding today and master Java Collections!