Sorting is a fundamental concept in Java programming and is frequently asked in technical interviews. It helps in organizing data efficiently, making search and retrieval operations faster. In this article, we will cover basic to advanced sorting techniques in Java, including both manual implementations and built-in sorting methods.
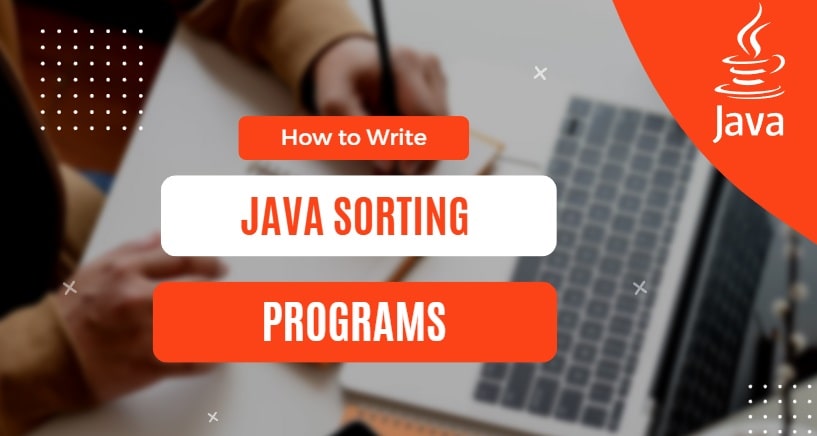
Basic Sorting Algorithms in Java
- Selection Sort – A simple sorting technique that repeatedly selects the smallest element and moves it to the correct position.
- Bubble Sort – A beginner-friendly algorithm that repeatedly swaps adjacent elements to sort the array.
- Insertion Sort – A method that builds a sorted list of one element at a time by placing each element in its correct position.
Advanced Sorting Algorithms in Java
- Merge Sort – A divide-and-conquer sorting algorithm that splits an array, sorts both halves and merges them efficiently.
- Quick Sort – A fast sorting algorithm that uses partitioning to arrange elements and works well for large datasets.
- Heap Sort – A comparison-based sorting technique that organizes elements into a heap structure before sorting them.
Built-in Sorting Methods in Java
- Arrays.sort() in Java – A powerful method from the Java Arrays class that sorts arrays efficiently using dual-pivot quicksort.
- Collections.sort() in Java – A method from the Java Collections class that sorts lists in ascending order.
Sorting algorithms are essential for optimizing performance in data processing applications. Understanding how different sorting techniques work will help you in competitive programming, real-world applications, and technical interviews. Start practicing these sorting programs today and enhance your Java skills!