Searching is one of the most important operations in Java programming, widely used in data structures and algorithms. A good understanding of searching techniques will help you optimize performance in problem-solving and real-world applications. In this article, we cover basic to advanced searching algorithms in Java, which are frequently asked in technical interviews.
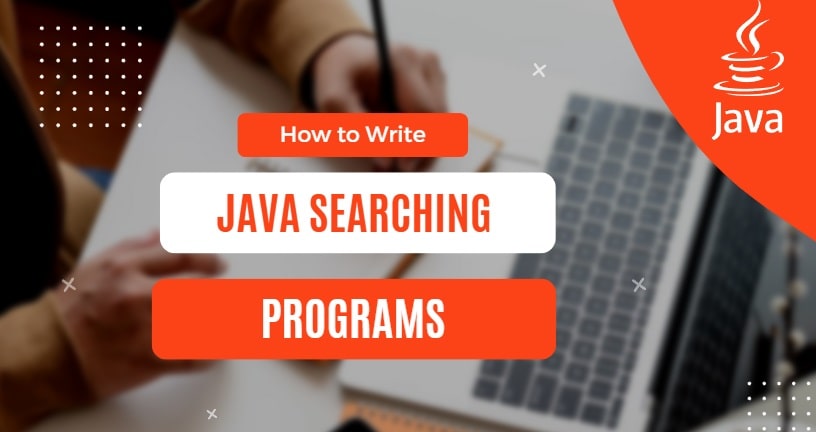
Basic Searching Algorithms in Java
- Linear Search in Java – The simplest searching algorithm that checks each element one by one until the target is found. Best for small or unsorted datasets.
- Binary Search in Java – A faster search method that works only on sorted arrays by dividing the search space into two halves in each step.
Advanced Searching Algorithms in Java
- Fibonacci Search in Java – A searching technique that divides the array using Fibonacci numbers, making it efficient for large datasets.
- Interpolation Search in Java – A modified version of binary search, best suited for uniformly distributed datasets.
- Exponential Search in Java – Used for searching in unbounded or infinite-sized arrays, starting with an exponential jump and then applying binary search.
- Jump Search in Java – An efficient searching method that divides the array into blocks and jumps ahead instead of checking every element.
- Ternary Search in Java – Similar to binary search but divides the array into three parts instead of two, optimizing performance in certain cases.
Searching is a fundamental concept that plays a crucial role in database indexing, information retrieval, and algorithm optimization. Mastering these search algorithms will help you excel in coding interviews and improve your problem-solving skills. Start practicing today and take your Java expertise to the next level!