Backend developers juggle APIs, databases, authentication, data structures, business logic, performance tuning, and a whole lot more. It’s a field that demands accuracy, efficiency, and clarity.
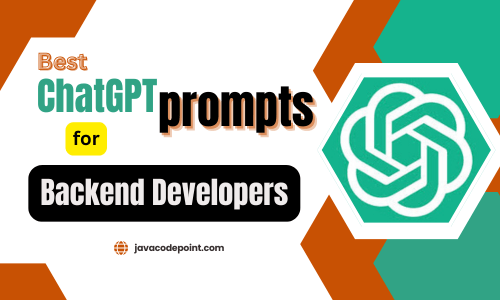
Enter ChatGPT — your virtual coding assistant. With the right prompts, you can use ChatGPT to:
Generate API endpoints
Optimize SQL queries
Write unit tests
Fix bugs
Create documentation
Handle edge cases
In this post, you’ll find the best ChatGPT prompts for backend developers, each with example code responses and practical explanations.
Table of Contents
1️⃣ ChatGPT Prompts to Generate REST API Endpoints
Write a REST API in Node.js with Express to manage a list of users. Include GET, POST, PUT, DELETE routes.
const express = require('express');
const app = express();
app.use(express.json());
let users = [];
app.get('/users', (req, res) => res.json(users));
app.post('/users', (req, res) => {
users.push(req.body);
res.status(201).json({ message: 'User added' });
});
app.put('/users/:id', (req, res) => {
const id = req.params.id;
users[id] = req.body;
res.json({ message: 'User updated' });
});
app.delete('/users/:id', (req, res) => {
const id = req.params.id;
users.splice(id, 1);
res.json({ message: 'User deleted' });
});
app.listen(3000, () => console.log('Server running on port 3000'));
Use Case:
Kickstart any REST API project without writing from scratch.
2️⃣ ChatGPT Prompts to Write SQL Queries for Common Use Cases
Write an SQL query to find the top 5 highest-paid employees in each department.
SELECT *
FROM (
SELECT *,
RANK() OVER (PARTITION BY department_id ORDER BY salary DESC) as rank
FROM employees
) ranked
WHERE rank <= 5;
Use Case:
Ideal when working with reporting, dashboards, or performance analytics.
3️⃣ ChatGPT Prompts to Generate Authentication Logic
Implement JWT-based login in Node.js using Express and jsonwebtoken.
const jwt = require('jsonwebtoken');
const SECRET_KEY = 'your_secret_key';
app.post('/login', (req, res) => {
const { username, password } = req.body;
// Mock validation
if (username === 'admin' && password === '1234') {
const token = jwt.sign({ username }, SECRET_KEY, { expiresIn: '1h' });
res.json({ token });
} else {
res.status(401).json({ message: 'Invalid credentials' });
}
});
Use Case:
Quickly integrate token-based authentication in your backend system.
4️⃣ Optimize Performance Bottlenecks
Suggest performance improvements for a slow MongoDB aggregation pipeline.
Add indexes on frequently filtered fields
Use $project early to reduce document size
Avoid $lookup unless indexed
Use $facet smartly
Use Case:
Enhance database efficiency and reduce response time in data-heavy APIs.
5️⃣ Debug Backend Code with ChatGPT
Why is this Express route not returning a response?
app.get('/test', (req, res) => {
const data = processData(); // missing await
res.send(data);
});
processData() might return a promise. You should await it or use .then():
app.get('/test', async (req, res) => {
const data = await processData();
res.send(data);
});
Use Case:
Perfect when stuck with async/await, middleware issues, or non-blocking logic bugs.
6️⃣ Write Unit Tests for API Endpoints with ChatGPT
Write unit tests using Jest for an Express GET endpoint /users.
const request = require('supertest');
const app = require('./app');
describe('GET /users', () => {
it('should return 200 and a list of users', async () => {
const res = await request(app).get('/users');
expect(res.statusCode).toBe(200);
expect(Array.isArray(res.body)).toBe(true);
});
});
Use Case:
Instant test cases for routes, controllers, services, and middlewares.
7️⃣ Convert Business Logic to Reusable Services
Convert this user creation logic to a reusable service in NestJS
// user.service.ts
@Injectable()
export class UserService {
private users = [];
createUser(user: CreateUserDto) {
this.users.push(user);
return { message: 'User created' };
}
}
Use Case:
Organize logic into services for better architecture and testability.
8️⃣ Generate API Documentation
Generate Swagger/OpenAPI documentation for a GET /products endpoint in Node.js.
paths:
/products:
get:
summary: Get all products
responses:
'200':
description: List of products
content:
application/json:
schema:
type: array
items:
$ref: '#/components/schemas/Product'
Use Case:
Save time documenting APIs, especially for teams or third-party integrations.
9️⃣ Suggest Security Best Practices
What are the best practices for securing a REST API?
Use HTTPS
Implement rate limiting
Use JWTs or OAuth2
Sanitize inputs to prevent SQL/NoSQL injection
Avoid exposing internal error messages
Validate request schemas
Use Case:
Great for audits, secure deployments, and working on fintech/healthcare APIs.
🔟 Generate Mock Data for Development
Generate JSON mock data for 5 blog posts with ID, title, author, date, and content.
[
{
"id": 1,
"title": "Understanding REST APIs",
"author": "Alice",
"date": "2024-01-15",
"content": "This post explains the basics of REST..."
},
...
]
Use Case:
Helps in mocking the frontend before APIs are ready or for local testing.
Conclusion
With the right prompts, ChatGPT can help backend developers:
- Save time writing boilerplate code
- Solve tough bugs
- Improve code quality
- Boost learning
- Maintain secure and scalable APIs
The key is to be clear, specific, and concise in your prompts to get the best responses.